Ever struggled with a pesky “Can’t open file” error in Python?
You’re not alone!
In this article, we’ll unravel the mystery behind this common issue and explore practical solutions to get you back on track.
Ready to become a file-handling ninja? Let’s dive in and discover the reasons for this error and how to fix it while keeping things light and engaging.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Important Before We Start: Mastering Python’s File Handling
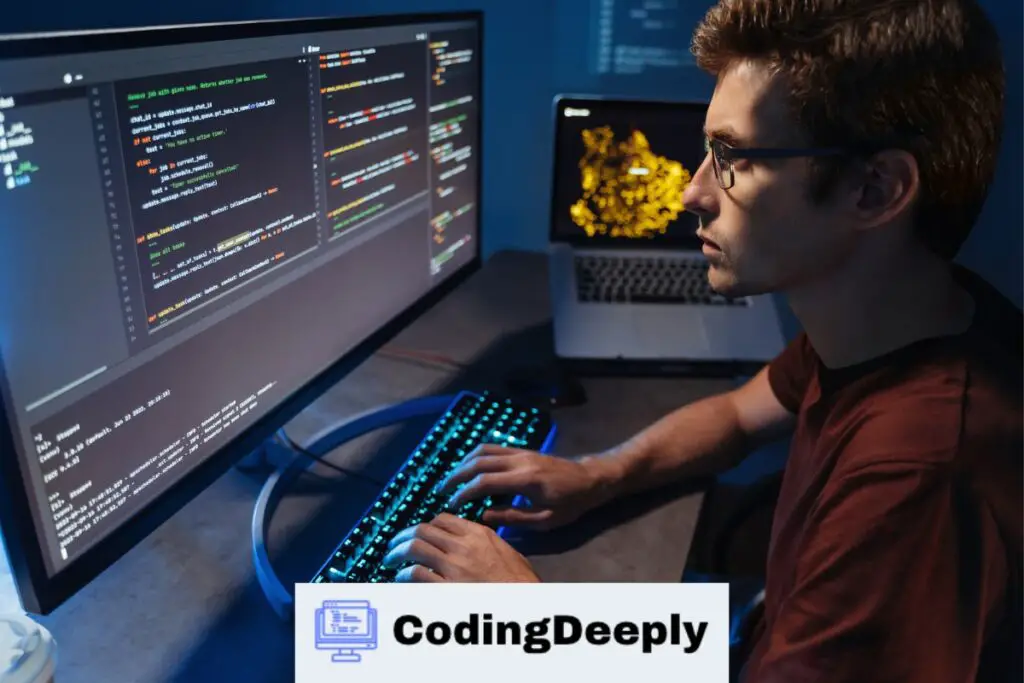
Do you want to work with files in Python? Great choice!
Python makes it super easy to read, write, and manipulate files. Let’s start by understanding the fundamentals, shall we?
Opening Files with Python
Python’s built-in open() function is your new best friend. It allows you to open files and work with them in different modes. Here’s a quick rundown of the most common modes:
- r: read mode, for reading files
- w: write mode, for creating new files or overwriting existing ones
- a: append mode, for adding content to existing files
Are you with me so far?
Good! Now, let’s see how to use the open() function:
file = open("example.txt", "r")
This line of code opens the file example.txt in read mode.
Simple. But wait, there’s more!
Handling Exceptions in File Operations
Sometimes, things go differently than planned. For example, you might encounter errors while working with files. Fret not. Python has got your back!
It provides exceptions that help you handle such situations gracefully.
Some standard file-handling exceptions are:
- FileNotFoundError: Raised when the file you’re trying to open doesn’t exist
- PermissionError: Raised when you don’t have the necessary permissions to access the file
Remember to handle these exceptions in your code to ensure smooth sailing. Now, let’s move on to the reasons behind that pesky “Can’t open file” error.
Uncovering the Reasons for the “Can’t Open File” Error
We’ve laid the groundwork; it’s time to dive into the heart of the matter.
Here are the most common reasons (the solutions are already waiting in the next chapter) for the “Can’t open file” error:
Incorrect File Path
Are you sure you’re pointing Python to the correct file? File paths can be tricky! Let’s break it down:
- Absolute file path: Refers to the complete path to the file, starting from the root directory. E.g., C:\Users\YourName\Documents\example.txt
- Relative file path: Refers to the path relative to your current working directory. E.g., example.txt or subfolder\example.txt
Take a moment to double-check your file path. Is it accurate? Let’s move on to the following reason.
The file Does Not Exist
This one might seem obvious, but it’s worth mentioning. Are you trying to open a file that doesn’t exist?
Python will raise a FileNotFoundError exception in this case. You can check for a file’s existence using the os.path.exists() function:
import os if os.path.exists("example.txt"): print("File exists!") else: print("File not found!")
Does your file exist? Great! If not, create it or adjust your code accordingly.
Insufficient Permissions
Uh-oh, you might need the necessary permissions to access the file! In this case, Python raises a PermissionError exception. File permissions vary based on the operating system and user account settings.
Are you trying to read from a write-protected file? Or perhaps attempting to write to a read-only file?
Double-check your file permissions and make sure they align with your intentions.
The file is in Use by Another Process
Sometimes, another program or process might use the file you’re trying to open. If you don’t close a file properly after using it, you might encounter issues when opening it again.
Using the with statement can help you avoid this problem, as it automatically closes the file once you’re done:
with open("example.txt", "r") as file: # Do something with the file
No more worrying about closing files manually!
Syntax Errors in the Code
Last but not least, take a close look at your code. For example, are you using the correct syntax when opening a file?
A typo or misplaced character can lead to the “Can’t open file” error.
Review your code, fix any syntax errors, and try again. You’ve got this!
Now that we’ve explored the possible reasons behind the “Can’t open file” error let’s learn how to tackle each issue in the next chapter.
Onward!
Fixing the “Can’t Open File” Error Like a Pro
You’ve made it this far; now it’s time to conquer the “Can’t open file” error! Armed with the possible reasons, let’s dive into practical solutions for each issue. Are you ready?
Verify the File Path
First things first, double-check your file path. Here’s how to make sure you’re pointing Python to the correct location:
Use os.path.abspath() to get the absolute path of a file:
import os abs_path = os.path.abspath("example.txt") print(abs_path)
If you’re working with multiple directories, os.path.join() can be a lifesaver:
import os path = os.path.join("folder", "subfolder", "example.txt") print(path)
Try these methods and see if your file path issue is resolved!
Check for File Existence
Remember that pesky FileNotFoundError? Here’s how to handle it like a champ:
import os if os.path.exists("example.txt"): with open("example.txt", "r") as file: # Do something with the file else: print("File not found!")
This way, you can prevent the error and take appropriate action.
Resolve Permission Issues
Have you encountered a PermissionError? Don’t worry. We’ve got your back! Here’s what you can do:
- Double-check your file permissions and adjust them if needed. This process depends on your operating system, so consult its documentation for details.
- Running your Python script with administrative privileges might sometimes be necessary. However, be cautious and only do this if you’re sure it’s safe!
Ensure Proper File Handling
You now know the importance of the with statement. Here’s a quick recap:
Always use the with statement when opening files:
with open("example.txt", "r") as file: # Do something with the file
If you need to close a file manually, use the close() method:
file = open("example.txt", "r") # Do something with the file file.close()
Proper file handling helps avoid conflicts with other processes!
Debug and Fix Syntax Errors
Last but not least, carefully review your code for syntax errors. Again, a keen eye and attention to detail will help you spot any mistakes.
- Check for typos and misplaced characters in your open() function call
- Make sure you’re using the correct file mode for your needs (e.g., “r”, “w”, or “a”)
Congratulations!
You’re now well-equipped to tackle Python’s “Can’t open file” error. Keep practicing and exploring more advanced file-handling techniques.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.