Ever wondered about the best way to append strings in Python loops?
Look no further!
We’ve covered you with useful tips and amazing techniques you can use immediately. So get ready to level up your coding skills and make your code more efficient and easily read.
Let’s dive in! 😎
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Key Takeaways
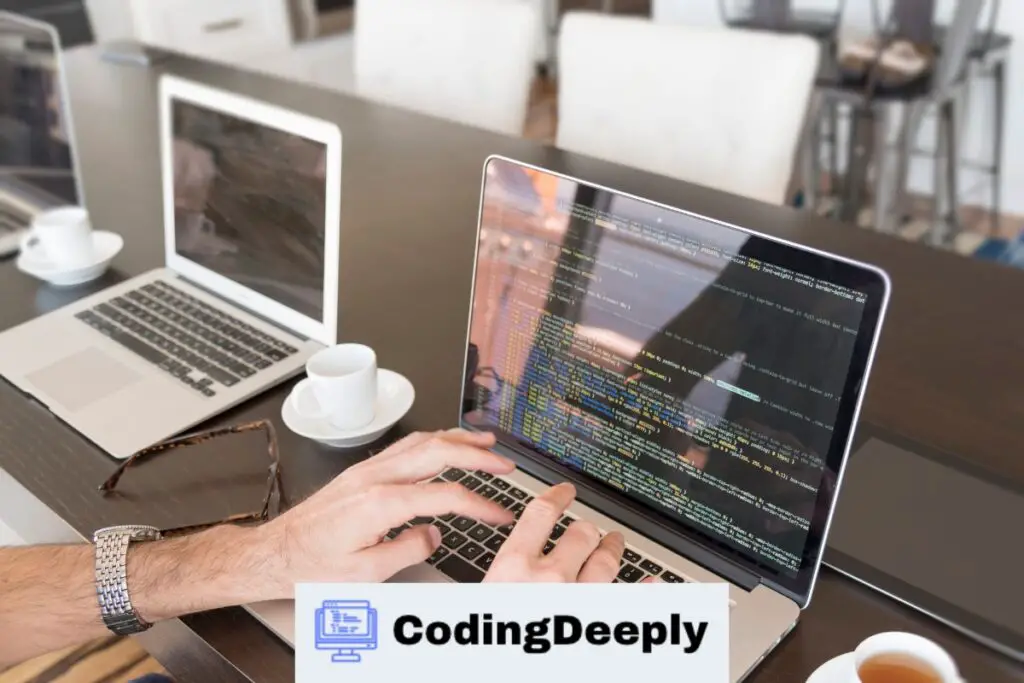
- Several techniques to append strings in Python loops include using the + operator, the join() method, list comprehensions, and f-strings.
- Each technique has pros and cons regarding performance, readability, and memory efficiency.
- When working with large data sets, choosing the right method to optimize performance and reduce memory usage is essential.
- Keep in mind the immutable nature of strings in Python, and avoid excessive concatenation in loops to maintain code readability and maintainability.
- Appending strings in loops is a versatile skill useful in many real-world situations, such as building dynamic SQL queries, generating HTML or XML files, and processing text.
Common Techniques to Append to a String in a Loop
Using the ‘+’ Operator
Syntax and Example
Let’s start with the most straightforward way to concatenate strings in Python: the + operator.
Here’s how it looks:
result = "" for word in words_list: result += word
Simple.
You loop through a list of words and add them to the result string.
Pros and Cons
While this method is easy to understand, there’s a catch: it could be more efficient.
Because strings are immutable in Python, you create a new string object each time you use the + operator. This can lead to performance issues when dealing with large amounts of data.
Using the ”.join() Method
Syntax and Example
The join() method is a more efficient way to concatenate strings.
Check it out:
result = "".join(words_list)
This one-liner does the trick!
It takes an iterable (like a list) of strings and combines them into a single string.
Pros and Cons
The join() method is way more efficient than the + operator, especially for large data sets.
It’s also easier to read.
However, if you’re dealing with non-string objects, you’ll have to convert them to strings before using this method.
Using a List Comprehension
Syntax and Example
List comprehensions are a cool Python feature that lets you create lists using a concise and readable syntax. You can use them to concatenate strings, too!
result = "".join([word for word in words_list])
This line of code creates a new list of strings using list comprehension and then uses join() to combine them.
Pros and Cons
List comprehensions are a great way to make your code more Pythonic and readable.
But they are harder to grasp for beginners. Also, like with join(), you’ll need to convert non-string objects to strings before using this method.
Using the f-string Method (Python 3.6+)
Syntax and Example
F-strings, introduced in Python 3.6, are a fantastic way to format and concatenate strings.
Here’s how you can use them in a loop:
result = "" for word in words_list: result = f"{result}{word}"
The f prefix tells Python it’s an f-string, allowing you to use curly braces {} to insert expressions directly into the string.
Pros and Cons
F-strings are super readable and make your code look clean. They also handle non-string objects easily by automatically converting them to strings. The only downside is that they’re available only in Python 3.6 and later versions.
So, there you have it!
Four awesome ways to append strings in loops. Try them out and find the one that fits your needs best.
Performance Comparison Between Techniques
Memory Efficiency
Now, let’s talk about memory efficiency. Since strings in Python are immutable, using the + operator can be quite memory-intensive, as each concatenation creates a new string object.
On the other hand, the join() method, list comprehensions, and f-strings are more memory-friendly, as they don’t create intermediate strings during the process.
So, if you’re concerned about memory usage, it’s better to avoid the + operator.
Speed and Performance
Regarding speed, the + operator must catch up again, especially when concatenating many strings.
The join() method and list comprehensions are the fastest techniques for most cases, while f-strings perform similarly to join() and can handle non-string objects without extra conversions.
So, if you’re after speed, go for the join() method, list comprehensions, or f-strings.
Choosing the Best Technique for Your Specific Use Case
So, which method should you choose?
It depends on your specific use case! If you’re a beginner and want something easy to understand, the + operator might be your best bet, but remember its limitations regarding memory and performance.
If you’re dealing with large data sets or care about speed, use the join() method or list comprehensions. And if you want clean, readable code that handles non-string objects easily, f-strings are the way to go.
In the end, the choice is yours! Try out different techniques and see which one works best for you.
Remember, writing efficient, readable, and easy-to-maintain code is the goal. So keep practicing, and you’ll get there in no time!
Common Pitfalls and Best Practices
Immutable Nature of Strings in Python
Always remember that strings in Python are immutable. This means you can’t change its content once you create a string.
Instead, any operation that appears to modify a string creates a new one. So, be mindful of this when appending strings, as it can affect performance and memory usage.
Avoiding Excessive Concatenation in Loops
More string concatenation in loops can lead to slow code and memory issues. To avoid these problems, use more efficient methods like join() or f-strings.
They’ll speed up your code, making it more readable and easier to maintain.
Properly Handling Large Data Sets
Choosing the right technique to append strings is crucial when working with large data sets.
As we’ve seen, there may be better choices than the + operator for this scenario. So instead, opt for join(), list comprehensions, or f-strings to ensure your code runs smoothly without hogging memory.
Ensuring Code Readability and Maintainability
Last but not least, always strive for easy-to-read and maintained code. Choose techniques that make your code more readable and avoid unnecessary complexity.
Remember, you (or someone else) might need to revisit your code later, so it’s essential to make it as clear and concise as possible.
By keeping these best practices in mind and avoiding common pitfalls, you’ll become a master at appending strings in Python loops.
Keep experimenting, learning, and improving your skills. You’ve got this!
Real-World Examples and Use Cases
Appending User Inputs in a Loop
Imagine you’re building a simple app that collects user inputs and stores them as a single string. Then, you could use any string concatenation techniques we’ve discussed to append each user input to the string in a loop.
Remember to choose the method that best fits your readability, performance, and memory usage needs.
Building Dynamic SQL Queries
When working with databases, you may need to build dynamic SQL queries based on user input or specific conditions.
Appending strings in a loop can help you construct these queries with ease.
For example, you could use f-strings to create a query by combining table names, column names, and values in a readable and maintainable way.
Generating HTML or XML Files Dynamically
If you’re creating a web app or working with XML data, you must dynamically generate HTML or XML files.
In this case, appending strings in a loop can help you build the file structure, add elements, and insert data. Techniques like join() or f-strings will make your code more efficient and readable.
Text Processing and Manipulation
Text processing is a common task in programming, and appending strings in loops is often necessary for jobs like tokenizing text, creating n-grams, or building word-frequency dictionaries.
Using efficient techniques like list comprehensions or the join() method can greatly improve your code’s performance in these scenarios.
As you can see, appending strings in loops is a versatile skill useful in many real-world situations.
By mastering the different techniques and knowing when to use each one, you’ll be better equipped to tackle various programming challenges. So keep practicing, and you’ll become a Python pro soon!
FAQ
Can I append strings with different data types in Python loops?
Yes, you can! However, you’ll need to convert non-string data types to strings before appending them. Using f-strings or str() makes this conversion easy and efficient.
How can I optimize string concatenation in nested loops?
Use the join() method or list comprehensions to optimize string concatenation in nested loops. These methods are more efficient and help reduce the performance impact of multiple loops.
Are there any Python libraries that can help with string concatenation in loops?
While Python’s built-in string methods cover most use cases, external libraries like numpy and pandas can provide additional functionality for working with strings in more complex scenarios, such as data analysis or scientific computing.
How do I handle errors and exceptions when appending strings in loops?
To handle errors and exceptions, use Python’s try and accept blocks. These allow you to catch exceptions, handle them gracefully, and continue the loop execution without terminating the program.
Wrapping It Up
And there you have it! We’ve explored various techniques to append strings in loops using Python and their pros, cons, and performance comparisons.
We’ve also covered common pitfalls, best practices, and real-world examples to help you understand when and how to use each method effectively.
Practice and experimentation are the keys to becoming a proficient Python programmer.
Try different techniques, learn from your mistakes, and always strive for efficient, readable, and maintainable code.
With dedication and perseverance, you’ll soon master the art of appending strings in Python loops and many other programming skills. So keep going, and happy coding!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.