Python is a popular programming language widely used for various purposes, from web development to data analysis and machine learning.
One essential aspect of writing clean and readable code is properly formatting the output, including adding whitespace or spacing between variables.
In this tutorial, you will learn how to print space between variables in Python, why it is important for better code readability and advanced techniques for formatting output. Whether a beginner or an experienced developer, this tutorial offers quick and easy steps to improve your coding skills and maintain a clean coding style.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding the importance of adding space between variables
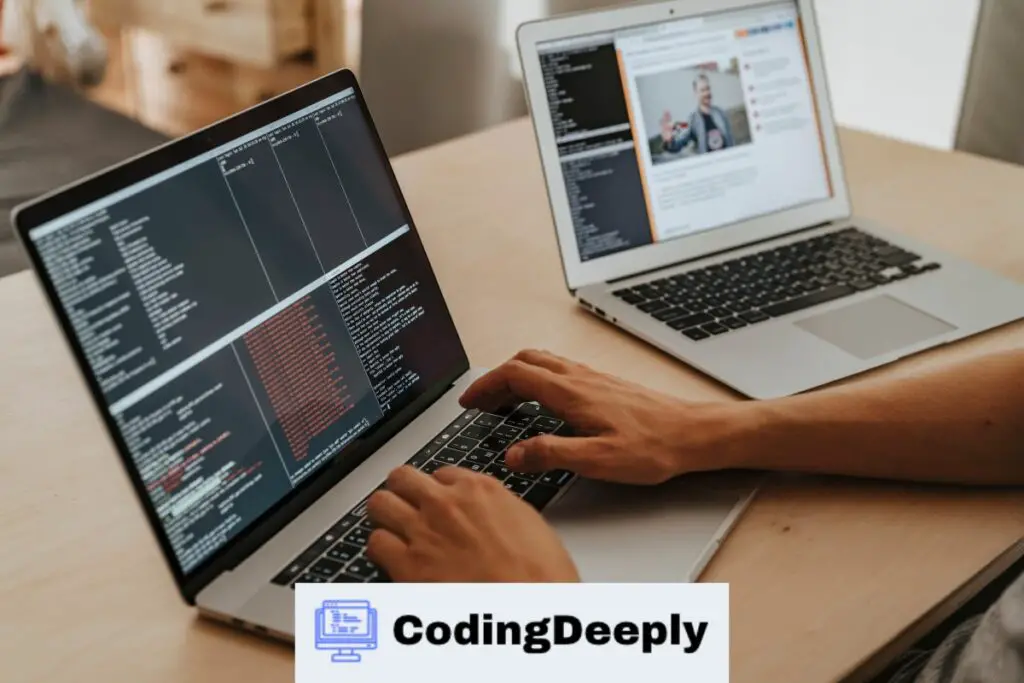
Python is a widely used programming language offering various built-in functions and modules.
It is essential to ensure readability when writing code, making it easy to understand and maintain. One crucial aspect of readability is proper spacing between variables and other elements.
Adding space between variables enhances the readability of the code, making it easier to read and comprehend.
Using proper spacing in coding helps programmers quickly identify and understand the code, thus shortening development time and reducing the likelihood of errors.
The importance of adding space for readability in Python
Proper spacing between variables and other elements of the code significantly increases readability. For instance, consider the following two lines of code:
x=5;y=10;z=15;
The above code may be challenging to read, especially for beginners. However, with proper spacing:
x = 5; y = 10; z = 15;
The code becomes more readable, and it is easy to identify the different variables.
When writing complex code, proper spacing helps the programmer organize and prioritize different parts of the code. It makes it easier and faster to identify errors and debug, thus saving time while developing the project.
Spacing also makes the code look more professional, which is essential when working on collaborative projects.
Other team members will likely appreciate and understand properly spaced code, enhancing teamwork and project success.
Using the print function to add space between variables
Now that we understand the importance of adding space between variables in Python, let’s explore how we can achieve this using the print function.
We can use different methods and techniques depending on our specific needs.
Separating variables with spaces using the print function
The most basic way to add space between variables is to separate them using a space character within the print function. For example:
print('Hello', 'World')
This will print “Hello World”, with a space separating the two variables. We can add even more variables by separating them with commas.
Using the separator argument
If we want more control over the spacing between variables, we can use the separator argument in the print function. By default, the separator argument is set to a space character. However, we can change it to any character of our choice. For example:
print('first', 'second', 'third', sep='-')
This will print “first-second-third”, with dashes (-) separating the variables instead of spaces.
Using string concatenation with space characters
Another way to add space between variables is to concatenate them using string concatenation with space characters. For example:
print('Hello' + ' ' + 'World')
This will print “Hello World” with a space character between the two strings. We can add more space characters for wider spacing.
The print function uses these basic methods for adding space between variables.
The next section will explore more advanced formatting options for adding space.
Formatting options for adding space between variables
While using the print function to add space between variables is sufficient for most cases, Python offers additional formatting options that provide more control and flexibility over the spacing.
These options allow for precise alignment and padding of variables, creating a more visually appealing and readable code.
Using the str.format() method
The str.format() method is a powerful way to format strings in Python. It allows for the insertion of variables into a string template, with various options for formatting and spacing. To add space between variables using the str.format() method, you can use the curly brackets {} with a space inside:
name = "John"
age = 25
print("My name is {} and I am {} years old.".format(name, age))
This will output:
My name is John and I am 25 years old.
You can also use the colon : to specify formatting options, such as setting a fixed width and alignment:
name = "John"
age = 25
print("My name is {:5} years old.".format(name, age))
This will output:
My name is John and I am 25 years old.
In this example, the curly brackets {} contain the placeholders for the variables, while the <:<10
and >:5
inside the curly brackets specify a left alignment with a width of 10 and a right alignment with a width of 5, respectively.
This results in a neatly aligned output.
F-strings
F-strings, also known as formatted string literals, offer a more concise and readable way to format strings in Python 3.6 and later versions. They allow for the direct insertion of variables into a string, with the values being evaluated and formatted during runtime:
name = "John"
age = 25
print(f"My name is {name} and I am {age} years old.")
This will output the same result as the previous example:
My name is John and I am 25 years old.
Like the str.format() method, f-strings also support formatting options:
name = "John"
age = 25
print(f"My name is {name:5} years old.")
This will output the same result as the previous str.format() example:
My name is John and I am 25 years old.
The % operator with string formatting
The % operator is an older method of string formatting in Python, but it is still widely used. It works by using placeholders, such as %s for strings and %d for integers, which are replaced by the corresponding variables:
name = "John"
age = 25
print("My name is %s and I am %d years old." % (name, age))
This will output the same result as the previous examples:
My name is John and I am 25 years old.
Similar to the other methods, the % operator also allows for formatting options:
name = "John"
age = 25
print("My name is %-10s and I am %5d years old." % (name, age))
This will output the same result as the str.format() and f-strings examples:
My name is John and I am 25 years old.
Overall, these formatting options provide a range of techniques for adding space between variables in Python, making it easy to create clean and readable code.
Best practices for adding space between variables
Proper spacing can greatly improve the readability and maintainability of your Python code. Here are some best practices to keep in mind when adding space between variables:
- Be consistent: Maintaining a consistent spacing style throughout your code is important. This not only makes it easier to read, but also helps identify errors or inconsistencies in code formatting.
- Use descriptive variable names: Using meaningful names for your variables can help reduce the need for excessive comments and improve code readability.
- Avoid excessive spacing: While some spacing is important for readability, excessive spacing can make your code look cluttered and difficult to read. Stick to a consistent style that is easy on the eyes.
- Use whitespace: Be sure to separate blocks of code and improve readability. This not only makes your code easier to read, but also helps identify sections of code with specific functionality.
- Avoid hardcoding: Hardcoding spaces between variables can be problematic if changes to the spacing are needed later on. Instead, use formatting options like f-strings or the str.format() method to achieve the desired spacing.
By following these best practices, you can improve the readability and maintainability of your Python code, making it easier to understand and modify as needed.
Advanced Techniques for Adding Space Between Variables
While the basic techniques covered in the previous section are sufficient for most situations, there are advanced techniques you can use to achieve more sophisticated spacing effects. These techniques include:
Aligning Variables with String Formatting Options
You can use string formatting options to align variables and create consistent spacing. The {} placeholders in the string represent the variables to be printed, and you can specify alignment and spacing options by adding additional formatting codes. For example:
num1 = 10 num2 = 20 print("{:<10} {}".format(num1, num2))
This code will align num1 to the left (using the < code) with a width of 10 characters, and num2 will be printed immediately after with no additional spacing.
Manipulating Whitespace Characters
You can use whitespace characters like spaces, tabs, and newlines to manipulate the spacing between variables. For example:
print("Hello,\tWorld!") print("One\nTwo\nThree")
This code will produce the output:
Hello, World! One Two Three
The \t character produces a tab, while the \n character creates a newline.
Using the join() Method for Concatenation
The join() method can be used to concatenate variables with specified delimiters to create custom spacing. For example:
list_of_words = ["hello", "world", "how", "are", "you"] print(" ".join(list_of_words))
This code will produce the output:
hello world how are you
The ” “ delimiter specifies a space between each list element.
Incorporating these advanced techniques into your Python code allows you to create more sophisticated and customizable spacing effects that enhance readability and maintainability.
Frequently Asked Questions (FAQs) about printing space between variables in Python
Do you still have questions about adding space between variables in Python? Check out these frequently asked questions for further clarification and guidance.
Q: How can I add space before or after a specific variable in my output?
To add space before a variable, you can use string concatenation and the space character (‘ ‘) before the variable. For example:
print('Hello, ' + name)
To add space after a variable, you can use string concatenation and the space character (‘ ‘) after the variable. For example:
print('Hello,' + name + ' how are you?')
Q: How do I handle multiple spaces between variables in my output?
If you have multiple spaces between variables in your output, you can use the join() method to concatenate the strings and replace any extra spaces with a single space character. For example:
print(' '.join([variable1, variable2, variable3]))
Q: What are some common mistakes to avoid when adding space between variables?
One common mistake is using too many spaces, making your code hard to read and follow. Another mistake is forgetting to include the space character between variables, which can cause your output to look cluttered and messy.
Remember to maintain consistent spacing and only add space where it is necessary for readability and clarity.
Q: How can I troubleshoot spacing issues in my output?
If you have trouble with spacing in your output, try using different formatting options like the str.format() method or f-strings to see if they produce different results. You can also experiment with manipulating whitespace characters and adjusting the separator argument in the print function.
Remember to keep in mind the principles of code readability and maintain a clean coding style to avoid spacing issues in the first place.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.