As a Python programmer, it’s quite common to frequently require printing the first 10 lines of code. It could be for debugging, analysis, or any other reason. Knowing how to do this efficiently can save you time and effort.
In this section, we will explore how to print the first 10 lines of code using Python. We will cover all the steps required to achieve this task efficiently and without hassle.
Keep reading to learn more.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding the concept of lines in Python
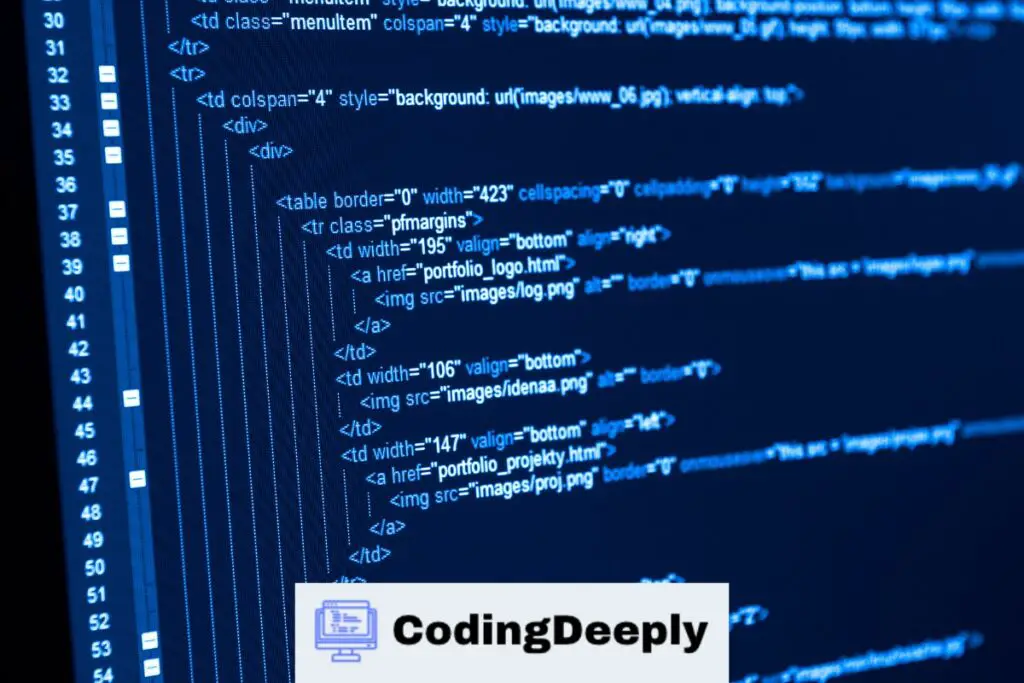
Before we dive into printing the first 10 lines of code using Python, it is essential to understand what we mean by lines in Python programming.
In Python, a line refers to a single instruction or sequence of characters between two line breaks. These line breaks can be represented by the newline character “\n” or the carriage return character “\r”.
When talking about lines of code, we refer to the individual instructions or sequences of characters written in a program. Each line of code is executed sequentially, one after the other.
Reading the file or code snippet
To print the first 10 lines of code using Python, we first need to read the file or code snippet we want to process. This can be achieved using Python’s open() function, which opens the file and returns a file object.
Let’s consider an example where we want to read a file named “example.txt” and print its first 10 lines. Here’s how we can accomplish this:
Code: | file = open(“example.txt”, “r”) |
---|---|
Description: | The open() function opens the file “example.txt” in read-only mode (‘r’) and returns a file object named “file”. |
It’s important to note that the file should be in the same directory as your Python script, or you should provide the full path to the file if it’s located elsewhere on your system.
If the file does not exist or you don’t have the necessary permissions to read it, Python will raise a FileNotFoundError or an IOError exception.
Once we have opened the file, we can read its contents using the readlines() method, which returns a list containing all the lines in the file:
Code: | lines = file.readlines() |
---|---|
Description: | The readlines() method reads all the lines from the file object “file” and stores them in a list named “lines”. |
After reading the file, we can close it using the close() method to free up system resources:
Code: | file.close() |
---|---|
Description: | The close() method closes the file object “file”. |
Now that we have the lines from the file stored in a list, we’re ready to move on to the next step: storing the lines in a list.
Storing lines in a list
Now that we have read the file or code snippet, the next step is to store all the lines in a list. This will help us access and manipulate the lines easily.
To store the lines in a list, we will create an empty list and then append each line to the list using a for loop. Here’s the code:
lines = [] for line in file: lines.append(line)
Here, we have initialized an empty list called ‘lines’. We then loop through each line in the file and append it to the ‘lines’ list using the append() method.
We can also use a list comprehension to achieve the same result in a single line of code:
lines = [line for line in file]
This code does the same thing as the previous code, but using a list comprehension instead of a for loop. It is a more concise way of writing the code.
Now that we have stored all the lines in a list, we can move on to the next step of printing the first 10 lines.
Printing the First 10 Lines
After storing the lines of the file or code snippet in a list, we need to print the first 10 lines. Here’s how you can do it:
Code | Explanation |
---|---|
| This code takes a slice of the list “lines” using the syntax [:10] to select the first 10 lines. Then, it uses a for loop to iterate over each line and print it. |
Alternatively, you can use a while loop to achieve the same results:
i = 0 while i < 10 and i < len(lines): print(lines[i]) i += 1
Both of these methods produce the same output: the first 10 lines of the file or code snippet. However, the first method is more concise and generally preferred.
Handling edge cases
While printing the first 10 lines of code in Python may seem straightforward, there are some potential issues to consider. Here are some edge cases to keep in mind:
Edge case | Solution |
---|---|
File or code snippet with less than 10 lines | Ignore blank lines while storing the lines in the list. Count only non-blank lines when printing the first 10 lines and adjust the range accordingly. |
File or code snippet with blank lines | Ignore blank lines while storing the lines in the list. When printing the first 10 lines, count only non-blank lines and adjust the range accordingly. |
File or code snippet with less than 10 non-blank lines | Similar to the first edge case, print all the non-blank lines if the list has less than 10 non-blank lines. |
File or code snippet with non-ASCII characters | Specify the encoding while reading the file to avoid any errors. For example, use the utf-8 encoding for Unicode characters. |
Considering these edge cases, you can ensure that your code to print the first 10 lines using Python works correctly and consistently.
FAQ about printing the first 10 lines in Python
Q: Can you print the first 20 lines instead of the first 10?
A: You can modify the code to print the desired number of lines. In the step where we slice the list to get the first 10 lines, you can change the value from 10 to 20 or any other number as per your requirement.
Q: What if the file or code snippet has less than 10 lines?
A: The code we have used to print the first 10 lines will print all the lines if the number of lines is less than 10. In case the file has no lines, the code will print nothing.
Q: How can I print the last 10 lines instead of the first 10?
A: You can modify the code to print the last 10 lines instead of the first 10. Instead of slicing the list to get the first 10 lines, you can slice it from the end using negative indexing. Here’s an example: print(lines[-10:])
Q: Can I print specific lines using this method?
A: You can modify the code to print specific lines instead of the first 10. Instead of slicing the list, you can use the index of the line you want to print. Here’s an example: print(lines[5])
will print the 6th line.
Q: What if the file or code snippet is too large?
A: If the file is too large, reading the entire file at once may not be the best approach. In such cases, you can read the file in chunks and process it accordingly. You can use the readline()
function inside a loop to read the file line by line.
Q: Can this method be used for files in different formats?
A: Yes, this method can be used for files in different formats as long as they are text files. However, some file formats may have special characters or formatting that affect the output. It’s important to consider these factors when printing the first 10 lines.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.