Printing an empty line in Python is a common task faced by programmers. It may seem simple, but multiple ways exist to achieve the desired result.
This article will provide a step-by-step guide on printing an empty line using Python.
We will cover three different methods, including the print() function with no arguments, the print() function with an empty string argument, and the escape sequence ‘\n’.
Additionally, we will address some frequently asked questions about printing empty lines in Python.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Method 1: Using the print() function with no arguments
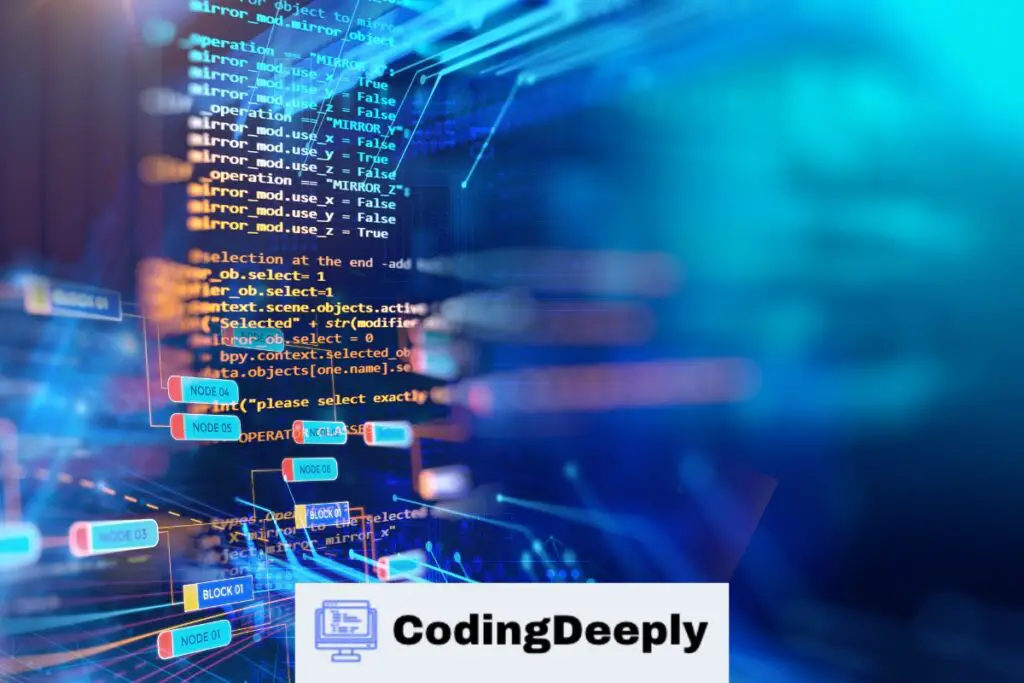
The print() function in Python is often used to display output on the screen. It can also be used to print an empty line.
The simplest way to do this is to call the print() function without arguments.
Here’s how:
Code | Output |
---|---|
print() |
The code above calls the print() function with no arguments, resulting in an empty line being printed to the console.
It is important to note that the print() function automatically adds a newline character at the end of each printed line, so we don’t need to add ‘\n’ to the argument list.
This method is particularly useful if you need to include an empty line between two lines of text:
Code | Output |
---|---|
print(“Hello, World!”) | Hello, World! |
print() | |
print(“Goodbye, World!”) | Goodbye, World! |
The code above will print “Hello, World!” on one line, an empty line on the next, and then “Goodbye, World!” on the following line.
Method 2: Using the print() function with an empty string argument
Another way to print an empty line in Python is to use the print() function with an empty string argument.
This method is similar to the first, but instead of not passing any arguments, we pass an empty string as the argument.
The syntax for using an empty string argument with the print() function looks like this:
Method | Syntax |
---|---|
Using an empty string argument | print(“”) |
As you can see, we pass an empty set of quotes as the argument to the print() function. When executed, this will result in a blank line being printed to the console.
Here’s an example:
print("Hello, world!") print("") print("Goodbye, world!")
Output:
Hello, world! Goodbye, world!
As you can see, the second print() statement, which has an empty string argument, results in an empty line being printed between the “Hello, world!” and “Goodbye, world!” lines.
Method 3: Using the escape sequence ‘\n’
Another way to print an empty line in Python is by using the escape sequence ‘\n’. This escape sequence represents a new line character in a string. When printed, it will create a line break, effectively printing an empty line.
To use the ‘\n’ escape sequence, include it as a string within the print() function, like this:
Example:
Code: | print(“This is the first line.\n\nThis is the third line.”) |
---|---|
Output: | This is the first line. |
This is the third line. |
In this example, we used the ‘\n’ escape sequence twice to create an empty line between the first and third lines.
It’s important to note that you must enclose the entire string in quotes when using escape sequences.
Otherwise, Python will treat the escape sequence as a literal string and won’t print the desired output.
FAQ: Printing an Empty Line in Python
Printing an empty line is a common task in Python programming. Here are some frequently asked questions related to printing empty lines in Python.
How do I print multiple empty lines?
To print multiple empty lines in Python, you can use the print() function with no arguments multiple times:
print() print() print()
This will print three empty lines.
Can I use a loop to print multiple empty lines?
Yes, you can use a loop to print multiple empty lines in Python:
for i in range(3): print()
This will print three empty lines.
What is the difference between using an empty string and the ‘\n’ character to print an empty line?
Using an empty string and the ‘\n’ character are two different approaches to print an empty line in Python.
When you use an empty string to argue the print() function, it will print nothing between the lines. This means that if you print a string after the empty line, it will be printed right after the empty line:
print("Line 1") print() print("Line 2")
This will output:
Line 1 Line 2
On the other hand, when you use the ‘\n’ character to print an empty line, it will print a newline character between the lines. This means that if you print a string after the empty line, it will be printed on the next line:
print("Line 1\n") print("Line 2")
This will output:
Line 1 Line 2
Notice that the second print statement is on a new line.
Can I print an empty line from the escape sequence ‘\r\n’?
Yes, you can use the escape sequence ‘\r\n’ to print an empty line, but it is not recommended.
The ‘\r\n’ sequence represents a Windows-style line break, consisting of a carriage return (‘\r’) followed by a line feed (‘\n’).
If you use ‘\r\n’ to print an empty line in Python may cause unexpected behavior when running your code on different platforms, particularly on Unix-based systems.
Is it necessary to print an empty line in my code?
No, printing an empty line in your code is not always necessary. You should only print an empty line if it serves a specific purpose, such as improving the readability of your output.
Printing unnecessary empty lines can make your code less efficient and harder to read. Therefore, you should only print an empty line if it adds value to your code.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.