If you’re a Python developer, you know that opening files one by one can be a time-consuming and tedious process.
However, opening multiple files simultaneously allows you to streamline your workflow and save precious time. In this article, we’ll show you how to do just that.
By the end of this guide, you’ll have a deep understanding of how to open multiple files at once in Python. We’ll also discuss the benefits of this method and provide best practices for efficient coding. Let’s dive in!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Why Open Multiple Files Simultaneously?
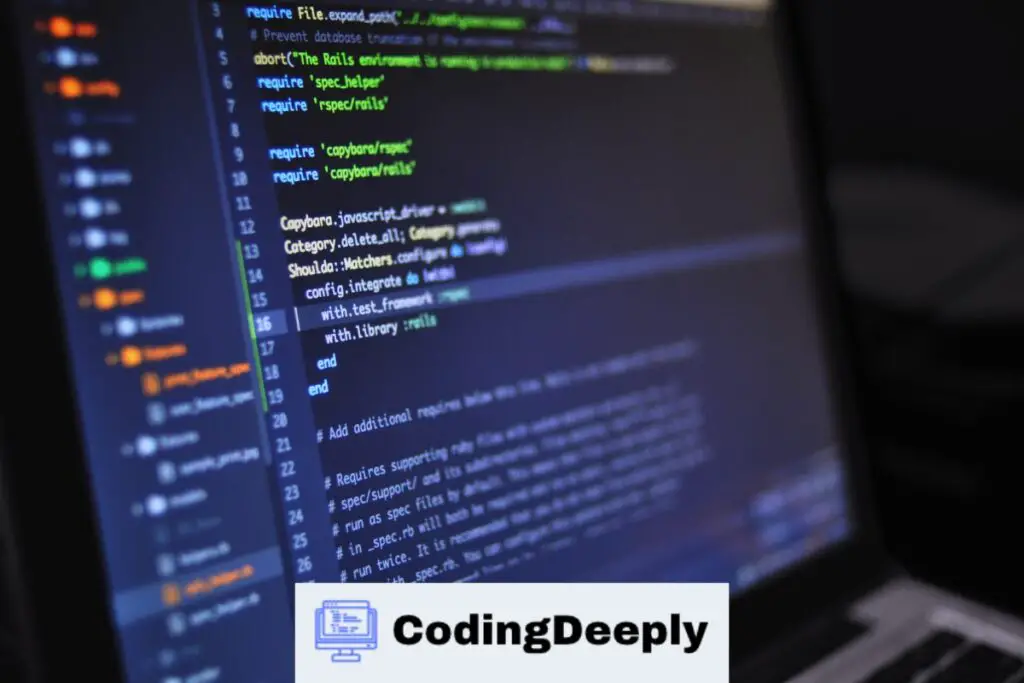
Opening multiple files simultaneously in Python can significantly impact your workflow.
Accessing multiple files at once can streamline your work process, increase speed, and reduce time spent on repetitive tasks.
Efficiency is essential in today’s fast-paced world, and optimizing your workflow by opening multiple files in Python can greatly improve your productivity. With this efficient method, you can easily access and process large amounts of data, making it a valuable tool for any developer or data analyst.
How to Open Multiple Files in Python
Opening multiple files can be a time-saving feature in Python, and in this section, we will guide you through the steps to achieve it. There are different methods to open multiple files, and we will explore a few of them.
Method 1: Using with statement and open() function
This method is the most common and widely used in Python. It uses the with statement in combination with the open() function to open multiple files:
Example: | with open(‘file1.txt’) as f1, open(‘file2.txt’) as f2: |
---|---|
Explanation: | We use the with statement to ensure that the files are closed after using them. The open() function is used to open each file, and the files are separated with a comma. |
Method 2: Using a list and for loop
This method is useful when you have many files to open. It uses a list to store the filenames and a for loop to open each file:
Example: | files = [‘file1.txt’, ‘file2.txt’, ‘file3.txt’] for file in files: with open(file) as f: #do something with each file |
---|---|
Explanation: | We create a list of files and use a for loop to iterate over each filename. In the loop, we use the with statement to open each file individually. |
Method 3: Using glob()
This method is useful when you want to open all the files with a particular extension in a directory. It uses the glob() function to find all the files with the specified extension:
Example: | import glob files = glob.glob(‘*.txt’) for file in files: with open(file) as f: #do something with each file |
---|---|
Explanation: | We import the glob module to use the glob() function. We use the function to find all the files with the .txt extension, and the for loop is used to open each file. |
These are some common methods to open multiple files in Python.
Choose the best suits your needs and start saving time and improving your workflow.
Handling Multiple File Types
Opening multiple files in Python is a great way to streamline your workflow and save time, but what about handling different file types?
Here, we’ll discuss how to handle various file formats so that you can make the most of this feature.
File Type | Method |
---|---|
Text (.txt) | open() function with “r” mode |
CSV (.csv) | csv.reader() function |
JSON (.json) | json.load() function |
XML (.xml) | xml.etree.ElementTree.parse() function |
As you can see, each file type requires a specific method for handling.
Using the appropriate method for each file format ensures that your code runs smoothly and efficiently.
Best Practices for Opening Multiple Files
Opening multiple files in Python can be a powerful tool, but following certain best practices is important to ensure your code runs efficiently and effectively.
Here are some tips to keep in mind when handling multiple files.
1. Use With Statements
When working with multiple files, ensuring they are properly closed when you’re finished handling them is important. To do this, use “with” statements. This will automatically close the file once you finish, even if an exception occurs.
2. Limit Memory Usage
If you’re working with large files, limiting the amount of memory your code uses is important. One way to do this is to process the files in smaller chunks rather than reading the entire file into memory simultaneously.
You can also use generators to read data from the files lazily.
3. Check for Errors
Always check for errors when opening multiple files. If a file is missing or cannot be opened, your program should handle the error gracefully. Use try-except statements to catch any errors that arise and handle them appropriately.
4. Use Efficient Coding Practices
To optimize the performance of your code, use efficient coding practices such as list comprehensions and built-in functions. This can help reduce the amount of code you need to write and make your code run faster.
5. Choose the Appropriate File Type
When opening multiple files, choose the appropriate file type for your needs. For example, if you’re working with text files, use the “open” function.
If you’re working with CSV files, use the “csv” module. Using the appropriate file type can make your code more efficient and easier to manage.
6. Close Files Properly
Always remember to close your files properly when you’re finished with them. This will ensure that your code runs smoothly and that there are no file handles or memory leaks issues.
FAQ – Frequently Asked Questions
Here are some common questions and concerns regarding opening multiple files in Python:
Q: Can I open multiple files of different file types?
A: Yes, you can open multiple files of different file types in Python. You need to specify the appropriate file type when opening each file.
Q: Is there a limit to the number of files I can open at once?
A: Theoretically, there is no limit to the number of files you can open at once. However, keep in mind that opening too many files at once can slow down your system and consume a lot of memory.
Q: How do I handle errors when opening multiple files?
A: You can handle errors using try-except statements. Using this method, you can catch any errors while opening files and handle them accordingly.
Q: Can I modify multiple files at once?
A: Yes, after opening multiple files, you can modify their contents using various Python modules, such as os and shutil.
Q: How do I close multiple files at once?
A: To close multiple files simultaneously, you can use a loop to iterate through the list of open files and call the close() method on each one.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.