Python is a versatile programming language that offers a simple and elegant syntax.
However, performing operations on each element in a list or array can become time-consuming and cumbersome when working with large datasets. This is where multiplying each element in Python comes in handy.
This section will explore different methods to multiply each element in a Python list or array. By the end of this article, you will have a deeper understanding of simplifying your code using these techniques.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Using a For Loop for Element-wise Multiplication
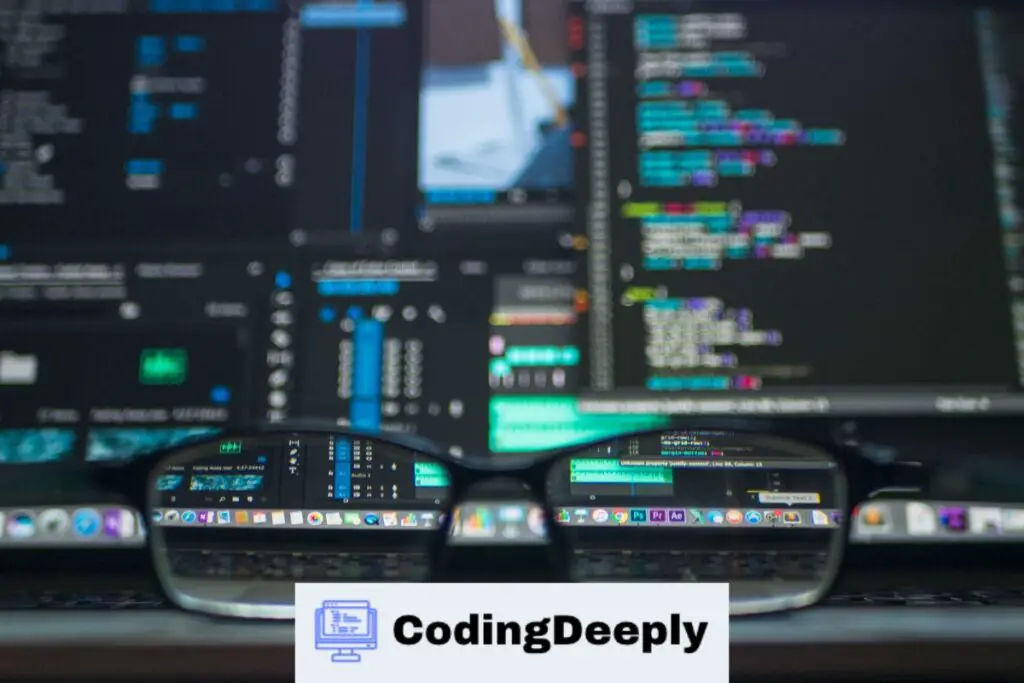
One way to multiply each element in a Python list or array is by using a for loop. This method lets you iterate through each element and perform the multiplication operation individually.
Here is an example code snippet that demonstrates how to use a for loop for element-wise multiplication:
Note: We will be using a list in this example, but the same concept applies to arrays as well.
Code: |
|
---|
In the above code, we create a list of numbers and initialize an empty list called “result”. We then use a for loop to iterate through each element in the “numbers” list and multiply it by 2, appending the result to the “result” list. Finally, we print the “result” list which contains the element-wise multiplication of the original “numbers” list.
This method is simple and easy to understand, making it a great choice for smaller lists or arrays.
Pros:
- Straightforward and easy to implement.
- No external libraries or modules needed.
Cons:
- Can be time-consuming for large lists or arrays.
- Not as efficient as other methods for element-wise multiplication.
While using a for loop for element-wise multiplication is a viable option, it may not be the best choice for larger lists or arrays.
The next section will explore a more efficient method using list comprehension.
Utilizing List Comprehension for Multiplying Elements
List comprehension is a concise and efficient method for multiplying each element in a Python list or array. It offers a more streamlined approach than using a for loop, making your code more readable and easier to maintain.
The syntax for list comprehension is straightforward. You can use it to perform operations on each element of an iterable object, such as a list or array, and return a new iterable with the modified values.
“List comprehension is a powerful tool for transforming data structures in Python. It allows you to express complex operations with just a few lines of code.”
To use list comprehension for element-wise multiplication, you can define a new list with the expression for the operation. Here’s an example:
Method: | [x * 2 for x in my_list] |
---|---|
Input: | [1, 2, 3, 4, 5] |
Output: | [2, 4, 6, 8, 10] |
In this example, we define a new list with the expression x * 2
, which multiplies each element in the original list by 2. The for loop iterates over each element in the original list and applies the expression to it.
One advantage of using list comprehension is that it is often faster than a for loop. Since list comprehension is implemented in C under the hood, it can be more efficient than Python’s interpreted for loop.
Another benefit of list comprehension is that it can be easier to read and understand than a for loop.
The compact syntax and lack of temporary variables make what the code is doing clearer, which can help make your code more maintainable.
Comparison to For Loop Method
Let’s compare the list comprehension method to using a for loop for element-wise multiplication:
Method: | List comprehension | For loop |
---|---|---|
Code: | [x * 2 for x in my_list] |
|
Advantages: | – More concise – Easier to read – Potentially faster | – More flexible – Can perform more complex operations – Can modify existing list in place |
As you can see, each method has its advantages and disadvantages. While list comprehension may be more concise and readable, a for loop can be more flexible and powerful.
Ultimately, the choice between list comprehension and a for loop will depend on your specific use case and personal preference. It’s worth experimenting with both methods to find the best solution for your particular task.
NumPy: Efficiently Multiplying Elements in Arrays
If you are working with large arrays in Python, NumPy can significantly improve the performance of your code. NumPy is a powerful library for scientific computing that provides optimized numerical operations on arrays, including element-wise multiplication.
To perform element-wise multiplication using NumPy, you can multiply two arrays with the *
operator.
For example:
import numpy as np
arr1 = np.array([1, 2, 3])
arr2 = np.array([4, 5, 6])
result = arr1 * arr2
print(result)
This will output:
[ 4 10 18]
NumPy also provides various useful functions for element-wise multiplication, such as multiply()
and prod()
.
The multiply()
function performs element-wise multiplication on two arrays, while the prod()
function returns the product of all elements in an array.
Here’s an example of using the prod()
function:
arr = np.array([1, 2, 3])
result = np.prod(arr)
print(result)
This will output:
6
Using NumPy for element-wise multiplication is faster than using a for loop or list comprehension and provides more flexibility and functionality. NumPy arrays can be sliced, reshaped, and transposed, which makes them suitable for various scientific and numerical computations.
FAQ: Common Questions about Multiplying Each Element in Python
As with any new concept, you may have some questions about multiplying each element in Python. Below are some frequently asked questions that we have answered to help improve your understanding of this topic.
What is the difference between the for loop and list comprehension methods?
The for loop method is useful when performing other operations alongside element-wise multiplication. It is also more flexible, as you can easily modify the code to fit your requirements.
On the other hand, list comprehension is more concise and easier to read. It is best used when the code needs to be simple and neat.
Why use NumPy instead of the built-in Python functions?
NumPy offers many advantages over the built-in Python functions when it comes to element-wise multiplication.
Firstly, it is faster due to its optimized algorithms. Secondly, it can handle large arrays more efficiently. Thirdly, NumPy offers more advanced functionality for scientific computing, such as linear algebra and Fourier transforms.
Can I multiply elements in a nested list or array?
Yes, you can.
However, you must use a nested for loop or list comprehension method to access the inner lists or arrays. Here’s an example code for multiplying elements in a nested list using a nested for loop:
lst = [[1, 2], [3, 4]] for i in range(len(lst)): for j in range(len(lst[i])): lst[i][j] *= 2 print(lst) // Output: [[2, 4], [6, 8]]
What data types can I use for element-wise multiplication?
You can use most data types in Python, such as integers, floats, and complex numbers. However, make sure that the data types are compatible with each other, or you may encounter unexpected results or errors. For example, multiplying a string with an integer will raise a TypeError.
We hope these answers have helped clear up some of your questions about multiplying each element in Python. Happy coding!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.