Python developers often work with large scripts and debugging code can be quite cumbersome. Oftentimes, it is necessary to jump to a specific line in the script to locate the issue quickly.
This guide will explore different ways to navigate through a Python script effortlessly. Whether you’re a beginner or an experienced programmer, this guide will help you learn how to jump to a specific line in Python.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Using the seek() method to jump to a specific line
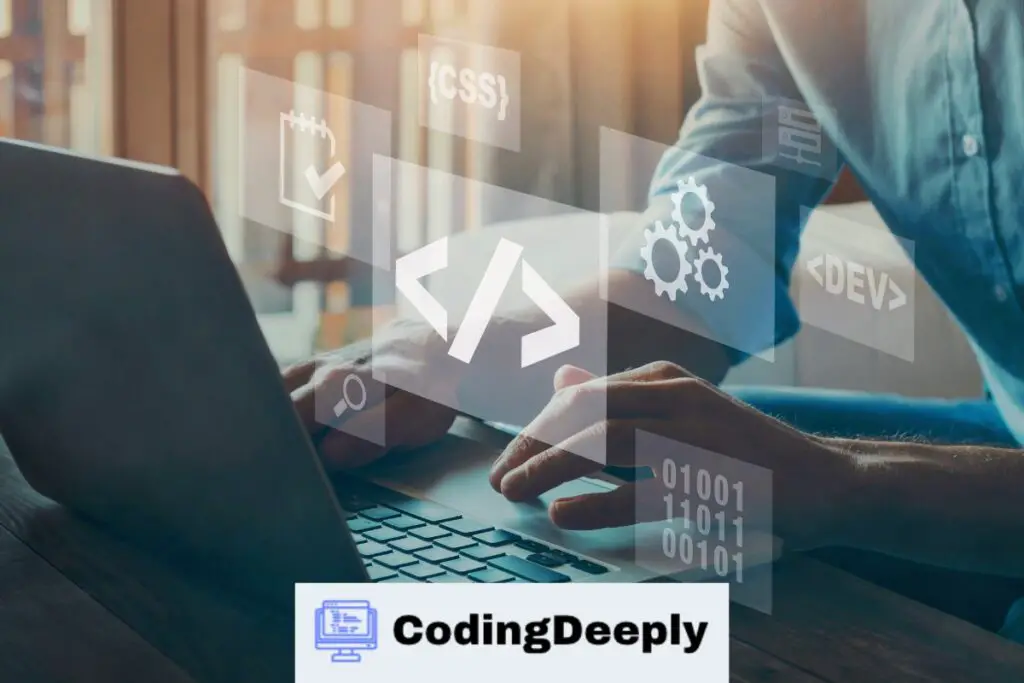
Python’s seek() method allows you to navigate to a specific position in a file. By using this method, we can efficiently jump to a desired line in our Python script.
The syntax for seek() method is as follows:
file_object.seek(offset[, whence])
The offset parameter specifies the number of bytes to move, while the optional whence parameter determines whether the offset is calculated from the beginning of the file (0), the current position (1), or the end of the file (2).
Now, let’s see how we can use seek() to jump to a specific line in our code:
Line Number | Code |
---|---|
1 | file_object = open(‘example.py’) |
2 | file_object.seek(0) |
3 | for line in file_object: |
4 | if ‘search_string’ in line: |
5 | file_object.seek(0) |
6 | break |
In this example, we are searching for the string ‘search_string’ in our Python script. If the string is found in the current line, we use the seek() method to move the file pointer back to the beginning of the file, allowing us to start again from the top.
This is just one example of how seek() can be used to jump to specific lines in a Python script. Experiment with different parameters to find the method that works best for you!
Finding line numbers in Python
We need to know the line number before jumping to a specific line in our Python script.
Fortunately, Python offers various ways to find line numbers.
Method 1: Using a text editor
If you’re using a text editor, you can usually see the line number of your current position in the bottom left corner. Alternatively, some editors provide a “go to line” feature that allows you to jump to a specific line.
Text Editor | Go to Line Shortcut |
---|---|
Visual Studio Code | Ctrl + G |
Sublime Text | Ctrl + G |
Atom | Ctrl + G |
Method 2: Using the built-in linecache module
The linecache module in Python allows you to get any line from any file, while also caching previously accessed lines for efficiency.
To use the linecache module:
- Import the linecache module using import linecache.
- Use the getline() method to get the desired line number of the file.
Example: Retrieve the 5th line of a file named “example.txt”.
import linecache
linecache.getline(“example.txt”, 5)
Method 3: Using the built-in traceback module
The traceback module in Python is primarily used for debugging but can also be used to get the currently executing line of code.
To use the traceback module:
- Import the traceback module using import traceback.
- Use the extract_stack() method to get a list of all currently executing code blocks.
- Get the last item from the list, which will be the currently executing block.
- Get the line number from the block using the lineno attribute.
Example: Get the line number of the currently executing block.
import traceback
lineno = traceback.extract_stack()[-1].lineno
By using one of these methods, you can find the line number you need to jump to in your Python script, making it faster and easier to navigate and debug your code.
Using breakpoints for line jumping in Python IDEs
Most Python Integrated Development Environments (IDEs) offer a great feature called breakpoints, which is particularly helpful when you want to pause the execution of your code at a particular line.
Using the breakpoint feature, you can easily jump to a line you choose without navigating through the entire script.
To set a breakpoint in your code, you need to click on the line where you want to pause the execution, usually in the margin area of the code editor. Once set, you can initiate the debugging mode, and the program will stop at that line, enabling you to inspect variables, step through the code line by line, or jump to another line quickly.
If you’re using PyCharm or another similar IDE, you can set breakpoints by simply clicking on the left margin of the code editor window. A red dot will show to indicate that the breakpoint is active.
Once the workflow is paused at the breakpoint, you can use the shortcuts provided by your IDE to jump to a specific line, using hotkeys like Ctrl+G in PyCharm, to jump to a line by number.
Frequently Asked Questions (FAQ)
Here are some commonly asked questions about jumping to a specific line in Python:
What is the seek() method in Python?
The seek() method in Python moves the file pointer to a specific position in a file. We can jump to a desired line in our Python script by utilizing this method.
How do I find line numbers in Python?
There are different ways to find line numbers in Python, including using the built-in enumerate() function or the linecache module. Understanding these methods will enable us to navigate our code more efficiently.
How do breakpoints work in Python IDEs?
Breakpoints are a handy feature offered by most Python Integrated Development Environments (IDEs) that allow programmers to pause the execution of their code at a specific line. This allows for convenient jumping to a desired line during the debugging process.
Why is it useful to jump to a specific line in Python?
Jumping to a specific line in Python can be useful for many reasons, including debugging your code or quickly navigating through a large script. It saves time and makes the coding process more efficient.
Can I jump to a specific line in a text file using Python?
Yes, you can jump to a specific line in a text file using Python by utilizing the seek() method. This is useful for reading large text files or navigating through data files.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.