If you are developing applications that require user authentication, it is crucial to ensure the security of user passwords.
One simple yet effective way to enhance password security is to display asterisks instead of the actual characters when inputting the password. This prevents potential attackers from stealing passwords by looking over the user’s shoulder or capturing the password using keylogging malware.
In this article, we will introduce the concept of securely inputting passwords in Python using asterisks to mask the actual characters. We will discuss the importance of this technique for enhancing the security of your applications.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Why Secure Password Input is Important
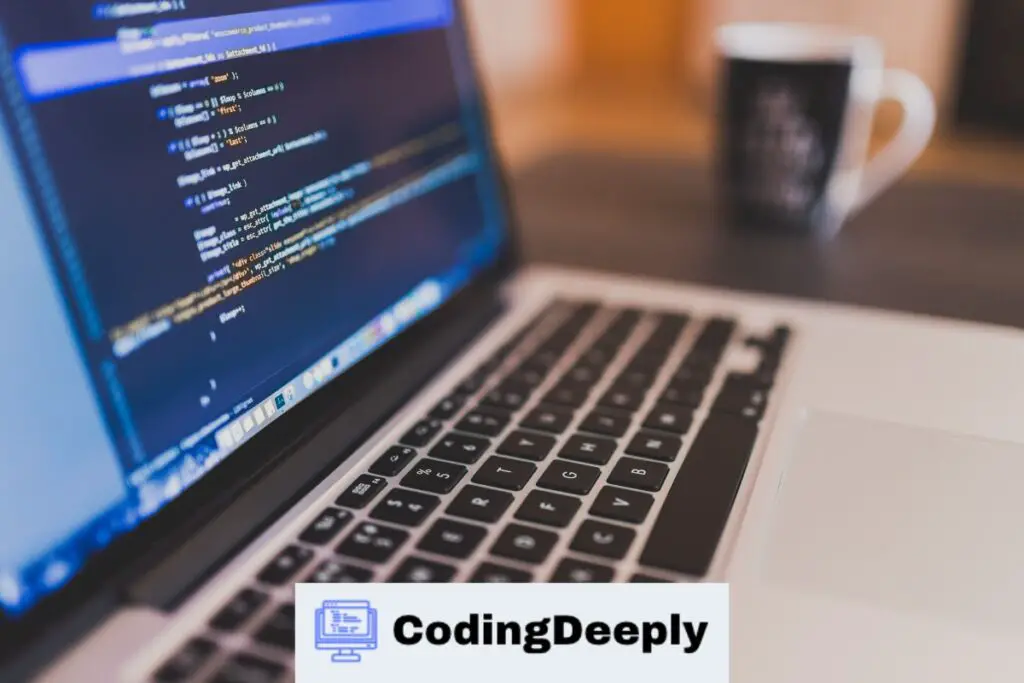
Secure password input is crucial for the overall security of your applications. Displaying passwords in plain text is not only unprofessional, but it also exposes them to potential security breaches.
By using asterisks to mask the actual characters, you can ensure that sensitive information remains secure.
Using secure password input not only protects your users’ data, but it also helps build trust between you and your clients. Clients are more likely to use your application if they know their information is secure.
As such, it is important to implement secure password input as a best practice in your development process.
Using the getpass Module in Python
When securely inputting passwords in Python, the getpass module offers a reliable and secure way to prompt users for password input.
Using this module, you can mask the password input with asterisks instead of displaying the actual characters, keeping the password hidden from prying eyes.
To use the getpass module, first import it into your Python script using the following code:
import getpass |
---|
Once you have imported the module, you can use the getpass() function to prompt the user for password input. Here’s an example of how to use the function:
password = getpass.getpass(prompt=’Enter your password: ‘) |
---|
In this example, the getpass() function prompts the user to enter their password and displays the message “Enter your password: ” to the user.
When the user enters their password, the characters are masked with asterisks, offering a secure way to input passwords in Python.
Password Masking with getpass()
By default, the getpass() function will mask the password input with asterisks.
This default behavior is considered a best practice for securely inputting passwords in Python.
However, you can customize the masking character by specifying it as an argument when calling the function.
Here’s an example of how to customize the masking character to use a hash (#) instead of an asterisk:
password = getpass.getpass(prompt='Enter your password: ', # Mask the password with a hash symbol masked=False, maskchar='#'
In this example, the getpass() function will mask the password input with a hash (#) instead of an asterisk.
The masked argument is set to False to ensure that the password is not masked with an asterisk, and the maskchar argument is set to ‘#’ to specify the masking character.
Implementing Password Input with Asterisks in Python
Now that we have discussed the importance of secure password input and explored the getpass module in Python, it’s time to implement password input with asterisks. Follow the steps below to ensure a secure process:
- Import the getpass module:
import getpass - Use the getpass function to prompt the user for a password input:
password = getpass.getpass(prompt=’Please enter your password: ‘, stream=None) - Optional: You can add a message to the prompt for further clarity. For example:
password = getpass.getpass(prompt=’Enter your account password (case-sensitive): ‘, stream=None) - Display asterisks instead of actual characters when the user inputs the password:
print(‘Password entered:’, ‘*’*len(password)) - You can also validate the password strength using regular expressions or other techniques for added security. For example, you can ensure that the password contains a mix of upper and lowercase letters, special characters, and numbers.
- Finally, ensure to store the password securely, such as using salted hashes or encryption techniques.
Following these steps, you can implement a secure password input process in your Python applications. Remember to regularly update passwords and follow best practices to enhance the security of your applications further.
Best Practices for Secure Password Input
Proper password input is critical to ensure the security of your applications. Below are some best practices to follow to enhance the security of your password input process.
Password Strength Validation
One essential practice is using password strength validation to encourage users to create strong passwords. A strong password should have a mix of uppercase and lowercase letters, numbers, and special characters. Additionally, passwords should be at least eight characters long.
You can use Python libraries like Password-Strength or zxcvbn to check for password strength and complexity. These tools can help you ensure that your users create strong passwords that can withstand attacks.
Use Salted Hashes
Another practice is to use salted hashes to store user passwords. Salting is adding random data to a password before hashing it, making it much more challenging to crack. Hashing algorithms like bcrypt or scrypt can help you generate salted hashes.
Also, avoid storing passwords in plain text. Instead, store hashed passwords in your database to prevent unauthorized access to user passwords.
Regular Password Updates
Encourage users to change their passwords regularly. Set up reminders to remind users to change their passwords periodically.
Doing so ensures that even if a user’s password is compromised, the exposure is limited to a brief period.
Limit Login Attempts
Limiting login attempts is another critical practice to prevent brute-force attacks.
Python libraries like Flask-Login or Django can limit login attempts to a set number.
These libraries can prevent users from making multiple login attempts quickly, limiting the risk of brute-force attacks.
Use Two-Factor Authentication
Two-factor authentication (2FA) is a practice that provides an additional layer of security. 2FA involves sending a one-time code to the user’s phone or email after they enter their password.
This code is required to access the account, making it much more challenging for attackers to gain access to an account with 2FA enabled.
Implementing 2FA adds layer of security to your applications, making it much more challenging for attackers to gain unauthorized access.
Conclusion
Implementing these best practices can significantly enhance the security of your password input process. Passwords are the first line of defense against unauthorized access to applications, and mitigating the risks associated with password security is critical for any application.
Additional Security Measures to Consider
While secure password input is crucial, it is not the only measure you should take to protect your applications. Here are additional security measures you can implement:
Two-Factor Authentication
Two-factor authentication adds an extra layer of security to your applications. It requires users to provide two forms of authentication, such as a password and a code sent to their phone or email. This reduces the risk of unauthorized access even if a password is compromised.
Password Encryption
Password encryption is the process of converting passwords into a form that hackers cannot easily read.
It uses algorithms to encrypt passwords, making them unreadable even if they are stolen.
Consider using password encryption techniques to enhance password security.
Regular Password Updates
Encourage users to update their passwords regularly.
This reduces the risk of compromised passwords and ensures that users use strong passwords that meet security requirements.
Minimize Data Collected
Only collect the data that is truly necessary for your application’s function. The more data you store, the more risk there is of a data breach.
Ensure that any sensitive data you collect, including passwords, is properly secured and encrypted.
Common Mistakes to Avoid
When implementing password input with asterisks in Python, it is essential to avoid common mistakes and errors that can compromise the security of your applications.
Here are some of the most common pitfalls to watch out for:
Avoid storing passwords in plain text
Storing passwords in plain text is a significant security risk. If a hacker gains access to your database, all passwords will be easily readable. Instead, use techniques such as salted hashes to store passwords securely.
Don’t rely solely on password strength requirements
While requiring strong passwords can enhance security, it is not foolproof. Users may still choose weak passwords or reuse passwords across different accounts.
Consider additional security measures such as two-factor authentication to protect user accounts further.
Avoid hard-coding passwords
Hard-coding passwords into your application’s source code is another significant security risk. Any developer with access to the code can view the passwords. Instead, use environment variables or configuration files to store sensitive information.
Don’t overlook regular password updates
Encourage users to update their passwords regularly to enhance security further. Consider implementing automated reminders or require periodic password changes.
By avoiding these common mistakes, you can ensure a robust and secure password input process in your applications.
FAQ – Frequently Asked Questions
Q: Is using asterisks to mask passwords a secure method?
A: Yes, using asterisks to mask passwords is a secure method as it prevents the password from being displayed in plain text. However, it should be noted that this method is not completely foolproof and additional security measures, such as password encryption, should also be implemented.
Q: How can I implement secure password input in my Python application?
A: To implement secure password input in your Python application, you can use the getpass module. This module allows you to display asterisks instead of the actual characters when the user enters their password. You can also implement additional security measures, such as password strength validation and hashing, to further enhance the security of your application.
Q: Can I use Python’s input() function to securely input passwords?
A: No, the input() function in Python is not secure for password input as it displays the password in plain text. It is recommended to use the getpass module or a similar secure input method to ensure the confidentiality of passwords.
Q: How often should I update my passwords?
A: It is recommended to update passwords regularly, such as every three to six months. Additionally, passwords should be updated immediately if there is a security breach or if they have been compromised in any way.
Q: What is two-factor authentication?
A: Two-factor authentication is a security process that requires users to provide two forms of identification before accessing an account or system. This can include something the user knows (such as a password) and something the user has (such as a security token or fingerprint). Two-factor authentication provides an extra layer of security to prevent unauthorized access to accounts or systems.
Q: What is password encryption?
A: Password encryption is converting a password into a coded or scrambled format. This makes it more difficult for attackers to access the password even if they manage to obtain it.
Various encryption algorithms can be used, such as AES and RSA, to encrypt passwords.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.