Python is a versatile programming language widely used to perform various file operations.
If you are looking for a quick and easy solution to extract the file name without the extension in Python, then you have come to the right place. This article will provide a step-by-step guide for getting the file name without extension in Python.
Our solution uses the os.path module, which provides functions for manipulating file paths. We will walk you through the necessary code and explain each step to ensure you understand the process.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding File Extensions in Python
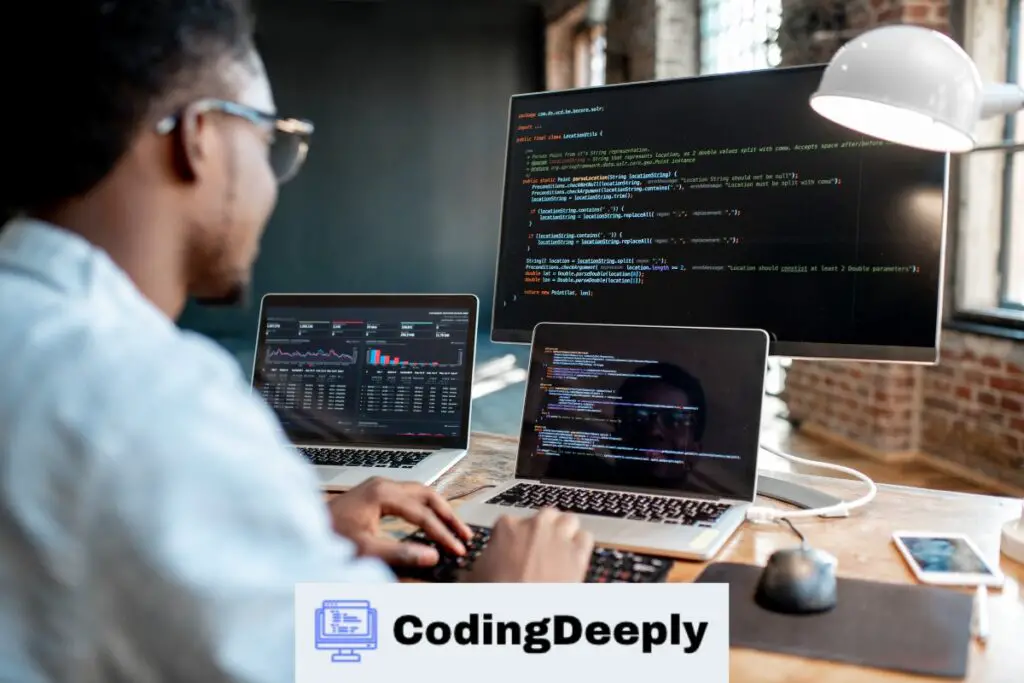
Before we dive into the code for getting file names without extensions in Python, it’s important to understand what file extensions are and how they work in Python.
In computing, a file extension is a series of characters that follow the last period in a filename, indicating the format or type of the file. For example, a file named “document.docx” has an extension of “.docx”, which indicates that it is a Microsoft Word document.
File extensions are important in Python because they allow the operating system and other software programs to identify and open files with the appropriate application.
In addition to identifying the file format, file extensions may also be used to indicate a text file’s encoding or character set.
Common File Extensions in Python
In Python, there are a variety of file extensions that are commonly used, each with its own specific file format or type:
Extension | File Type |
---|---|
.txt | Text file |
.csv | Comma-separated values file |
.json | JavaScript Object Notation file |
.py | Python script file |
.html | HTML file |
Portable Document Format file |
Understanding file extensions is important when working with files in Python, as it allows you to correctly identify the type of file you’re working with and determine the appropriate methods for manipulating it.
Using the os.path module for File Operations
Python provides several built-in modules for performing different file operations. One such module is the os.path module, which handles file pathnames.
The following functions are commonly used for file operations:
Function | Description |
---|---|
os.path.basename() | Returns the base name of a file path, which is the last component of the path. |
os.path.splitext() | Splits a file path into its base name and extension, returning them as a tuple. |
The os.path.basename() function is used to extract the file name from the file path, while the os.path.splitext() function separates the file name and extension.
We can easily extract the file name without the extension by using these functions together.
Example:
Let’s say we have a file path /path/to/myfile.txt
. We want to extract the file name without the extension, which should be myfile
.
Here’s how we can do it using the os.path module:
import os file_path = "/path/to/myfile.txt" # get file name with extension file_name = os.path.basename(file_path) # get file name without extension file_name_without_extension = os.path.splitext(file_name)[0] print(file_name_without_extension)
The output of this program will be:
myfile
As you can see, we have successfully extracted the file name without the extension.
Extracting File Name Without Extension
Now that we understand the significance of file extensions and how to use the os.path module for file operations, let’s dive into the code for extracting the file name without the extension in Python.
To extract the file name without the extension, we can use the os.path.basename() function to get the base name of the file path, which includes the file extension.
Then, we can use the os.path.splitext() function to split the file name and extension into a tuple, and return only the first element of the tuple, which is the file name without the extension.
Code Snippet:
Code: | file_path = ‘/path/to/myfile.txt’ file_name = os.path.basename(file_path) file_name_without_extension = os.path.splitext(file_name)[0] |
---|---|
Output: | ‘myfile’ |
Let’s break down the code and explain each step in detail:
- First, we define the file path as a string variable named file_path.
- Next, we use the os.path.basename() function to get the base name of the file path, which is the file name including the extension. We assign the value to a new variable named file_name.
- Finally, we use the os.path.splitext() function to split the file name and extension into a tuple, and return only the first element of the tuple using the index [0], which is the file name without the extension. We assign the value to a new variable named file_name_without_extension.
It’s important to note that this solution works for files with any extension, not just .txt files. Additionally, the code snippet is concise and efficient, making it a great solution for extracting file names without extensions in Python.
Benefits of Using the Provided Solution
The solution for extracting file names without extensions in Python using the os.path module offers various benefits:
- Simplicity: The code to extract file names without extensions is relatively simple and easy to understand, making it a great solution for beginners.
- Efficiency: Using the os.path module saves you time and effort as it provides a ready-made method to extract file names without extensions.
- Readability: The solution makes your source code more readable and organized by eliminating the need for complex code and reducing clutter.
“The provided solution using the os.path module is a great way to simplify your code and save time when working with file names in Python.”
Frequently Asked Questions (FAQ)
Here are the most commonly asked questions about extracting file names without extensions in Python:
Q: Can I still access the file extension after removing it from the file name?
A: You can still access the file extension separately using Python’s built-in string methods.
Q: What happens if the file doesn’t have an extension?
A: The entire file name will be returned if the file doesn’t have an extension.
Q: Does this method work for all file types?
A: This method works for all file types, including those with multiple extensions.
Q: Can I use os.path to change the file name without extension?
A: Yes, you can use os.path to rename the file without the extension by specifying a new file name that does not include the extension.
Q: Is this method more efficient for extracting file names?
A: Yes, this method is more efficient and less prone to errors than other methods, such as using regular expressions.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.