In today’s dynamic and data-driven programming world, generating random values is an essential skill for any developer. Python provides a variety of built-in modules, functions, and methods to generate random data.
This tutorial will focus on generating random true/false values in Python.
Random true/false values are commonly used in decision-making applications, simulations, and games. They can be generated using various methods available in the random module of Python’s standard library.
Whether you are a beginner or a seasoned Python developer, this tutorial is designed to provide you with a clear understanding of the random module and its functions, and enable you to generate random true/false values with ease.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding the random module in Python
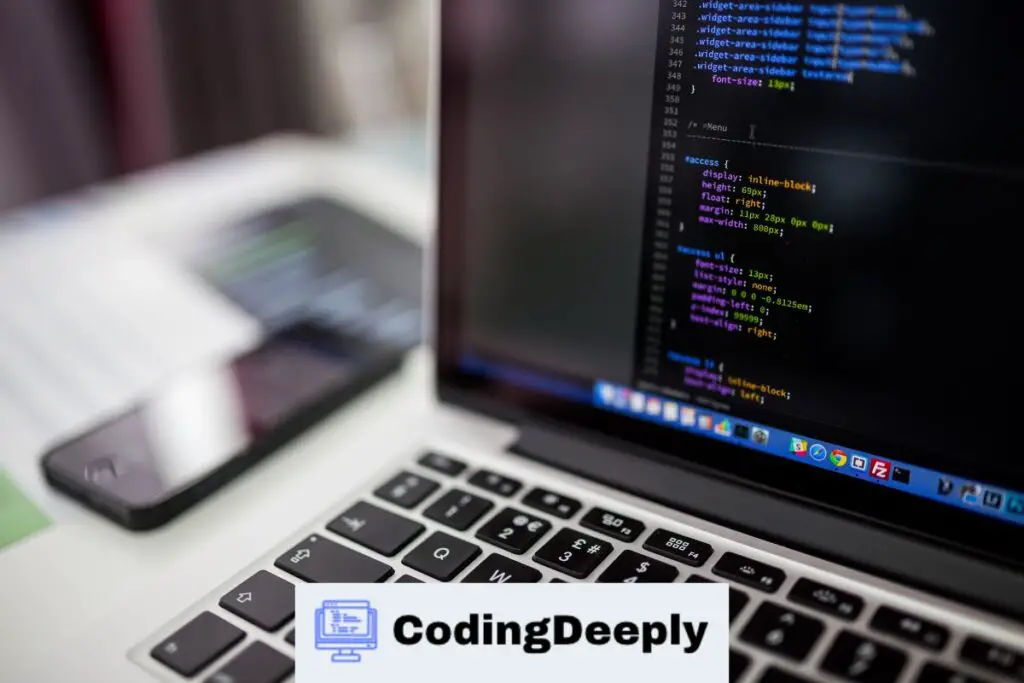
If you want to generate random true/false values, you must familiarize yourself with Python’s random module. This module provides a range of functions for generating random values, including integers, floating-point numbers, and sequences.
The random module is part of Python’s standard library and requires no additional installation. You can import the module into your Python script using the following code:
import random
Generating random values
The random module provides various functions for generating random values. Some of the most commonly used functions include:
- random.random(): This function generates a random floating-point number between 0 and 1.
- random.randint(a, b): This function generates a random integer between a and b (inclusive).
- random.choice(seq): This function returns a random element from the given sequence.
Generating true/false values
To generate true/false values, you can use any of the above functions and convert the output to a boolean value. Alternatively, you can use the randint function and map the output to a boolean value using the following code:
random_boolean = bool(random.randint(0, 1))
Another option is to use the choice function to select either True or False from a list randomly:
random_boolean = random.choice([True, False])
Both methods are effective for generating random true/false values, and the choice function may be more straightforward to use if you only need to generate true/false values.
Generating random true/false values using randint
The randint function in Python’s random module can generate random true/false values. This function returns an inclusive random integer between the specified start and end values. By setting the start value to 0 and the end value to 1, we can generate random true/false values.
Here is an example of how to generate a random true/false value using randint:
- Import the random module: import random
- Generate a random number using randint: random_number = random.randint(0, 1)
- Convert the random number to a true/false value: true_or_false = bool(random_number)
In step 2, we generate a random number between 0 and 1 (inclusive). In step 3, we convert this number to a true/false value using the bool function. If the random number is 0, bool will return False. If the random number is 1, bool will return True.
Here’s the full code:
import random random_number = random.randint(0, 1) true_or_false = bool(random_number) print(true_or_false)
This will output either True or False, chosen randomly.
Generating random true/false values using random.choice
Another popular method for generating random true/false values in Python is using the random.choice() function from the random module. This function takes an iterable as an argument and returns a randomly chosen element from that iterable.
To generate true/false values, we can pass a list or tuple containing the values True and False to the random.choice() function. Here is an example:
import random result = random.choice([True, False]) print(result) # Output: either True or False
The random.choice() function can also be used with other iterables, such as strings:
import random result = random.choice("hello") print(result) # Output: a randomly chosen letter from "hello"
While the random.choice() function can be a convenient method for generating random true/false values, it may not be suitable for all use cases. For example, if you need to generate many values, using random.choice() with a list can be inefficient.
Consider using the random.randint() function instead, as it can generate random integers representing true/false values with greater efficiency.
Best Practices for Using Random True/False Values
Random true/false values are an essential tool in Python programming. However, it is equally important to use them correctly to avoid common mistakes that can result in errors and bugs. Here are some best practices to consider when working with random true/false values.
Seed Values
Using a seed value in a random function ensures that the results are reproducible.
- Use a constant value for the seed to ensure repeatability.
- Avoid seed values that are too small or too large as they may produce less random results.
Randomness Control
Python provides various ways to control the randomness of true/false values. Here are some tips to help you achieve better randomness control:
- Use the random.seed() function to initialize the random number generator.
- Use the random.getrandbits() function to generate random bits.
- Use the random.shuffle() function to shuffle the sequence of true/false values.
Common Mistakes to Avoid
When using random true/false values in Python, it is important to avoid some common mistakes that can result in errors or bugs:
- Do not forget to import the random module before using it.
- Do not use the same seed value for different random functions.
- Do not assume that the results of a random function are truly random.
By following these best practices, you can use random true/false values effectively in your Python code and avoid common mistakes that can cause problems in your program.
Frequently Asked Questions about generating random true/false values in Python
Here are some common questions and concerns regarding generating random true/false values in Python:
Q: Why must I use random true/false values in my Python code?
A: Random true/false values can be useful in many situations, such as simulating user input or generating test data. They can add an element of unpredictability and dynamism to your code.
Q: Is it possible to control the randomness of the generated values?
A: It is possible to control the randomness using the random module’s seed function. By setting the seed value, you can generate the same sequence of random values every time you run the code.
Q: How can I avoid common mistakes when generating random values in Python?
A: One common mistake is using the random values without proper validation, which can lead to unexpected behavior. Another mistake is not setting a seed value when repeatability is important. To avoid these mistakes, always validate the random values and set a seed value if necessary.
Q: What is the difference between using randint and choice to generate random true/false values?
A: The randint function generates random integer values within a specified range, while the choice function selects a random value from a sequence. For generating true/false values, the choice is often preferred as it requires less code and clarifies the code’s intent.
Q: Are there any security concerns when using random values in Python?
A: There are potential security concerns when generating random values for cryptographic purposes. In these scenarios, it’s important to use a secure random number generator, such as the secrets module, which provides access to a cryptographically secure pseudo-random generator.
By following these best practices and understanding the random module’s capabilities, you can confidently use random true/false values in your Python code.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.