As a programmer, you know that efficient string manipulation is essential in any project. In this section, we will share some valuable tips and tricks that will help you locate the second occurrence of a specific substring in Python.
By the end of this section, you will better understand how to manipulate strings in Python.
You’ll learn how to use the find() method to locate the first occurrence of a substring and how to slice the string to find the second occurrence. So, let’s dive in and get started!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding String Manipulation in Python
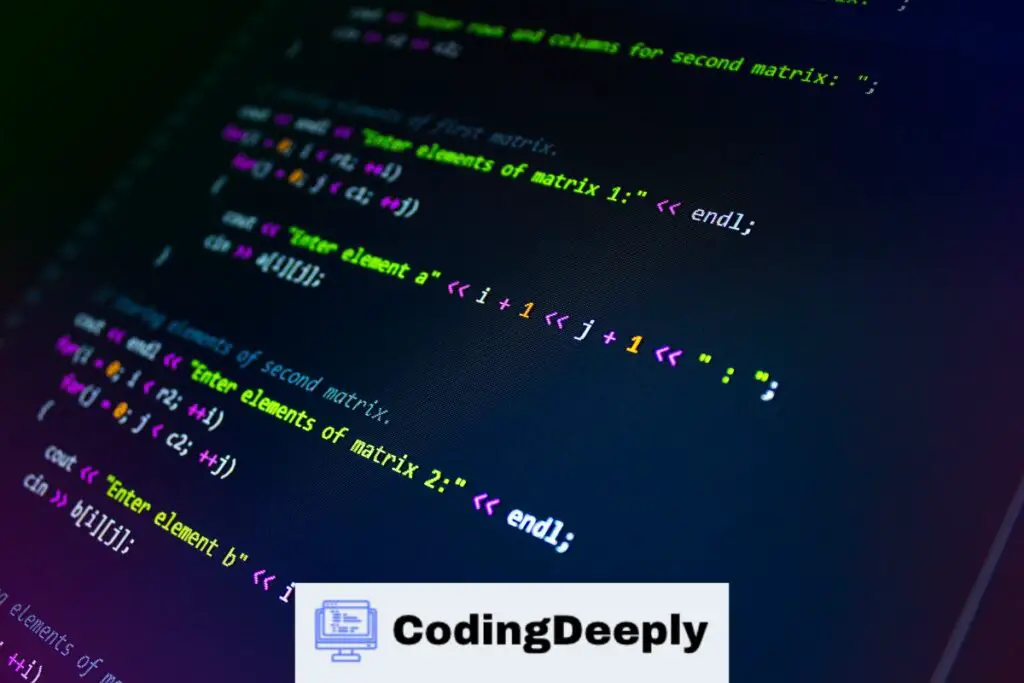
Python is a powerful programming language that offers a variety of tools for manipulating strings. String manipulation refers to the process of modifying and extracting information from a string of characters.
This can be particularly useful when working with data, text-based applications, or even web development.
What is a String?
In Python, a string is a sequence of characters. It can be created by enclosing a sequence of characters in quotes, either single quotes or double quotes. For example:
'hello'
"world"
"123"
Strings are immutable, meaning that once they are created, they cannot be modified. However, you can create a new string based on the original string with modifications, such as using slicing or concatenation.
Common String Operations
Python provides a wide range of built-in functions and methods for manipulating strings. Here are some common operations:
len(string)
: returns the length of the stringstring.lower()
: returns a new string with all uppercase characters converted to lowercasestring.upper()
: returns a new string with all lowercase characters converted to uppercasestring.strip()
: returns a new string with all leading and trailing whitespace removedstring.split()
: returns a list of substrings separated by a specified delimiter
These operations can be useful for a variety of tasks, such as cleaning and formatting data or parsing text-based files.
Using the find() Method to Locate the First Occurrence
Before we can find the second occurrence of a substring in a string, we first need to know how to locate the first occurrence. This is where the find() method comes in handy.
What is the find() method in Python?
The find() method is a built-in function in Python that allows us to search for a specific substring within a string. It returns the index of the first occurrence of the substring, or -1 if the substring is not found.
How to use the find() method to locate the first occurrence?
The syntax for using the find() method is as follows:
- string.find(substring, start_index, end_index)
Where:
- string – the string to be searched
- substring – the substring to search for
- start_index (optional) – the index to start the search from
- end_index (optional) – the index to end the search at
If start_index and end_index are not specified, the search will start from the beginning and end at the end of the string, respectively.
Example:
Suppose we have the following string:
string = “Python is a great language. Python is used for web development.”
To find the index of the first occurrence of the substring “Python”, we can use the following code:
- index = string.find(“Python”)
This will return the value 0, since “Python” is located at the beginning of the string.
If we wanted to start the search from a specific index, we could include the start_index parameter:
- index = string.find(“Python”, 15)
This will return the value 27, since the first occurrence of “Python” after index 15 is at position 27.
It’s important to note that the find() method is case-sensitive. If we searched for “python” instead of “Python”, it would not be found.
Finding the Second Occurrence Using find() and slicing
Now that we have located the index of the first occurrence using the find() method, we can use slicing techniques to search for the second occurrence within the remaining portion of the string.
Step 1: Use Slicing to Define the Substring
We need to define the substring we want to search for the second occurrence. We can use slicing to do this.
The starting index for our slice should be the index of the first occurrence plus the length of the substring, as we want to exclude the first occurrence from our search.
Here’s an example:
string = "apple banana apple cherry apple"
first_occurrence = string.find("apple")
substring = string[first_occurrence+len("apple"):]
In this example, the substring
variable will be assigned the value " banana apple cherry apple"
, which is the portion of the original string that comes after the first occurrence of “apple”.
Step 2: Use find() to Locate the Second Occurrence
With our substring defined, we can now use the find() method again to locate the second occurrence:
second_occurrence = substring.find("apple")
This will give us the index of the second occurrence within the substring
variable. However, we need to adjust this index to account for the portion of the original string that we excluded with our slice:
actual_index = first_occurrence + len("apple") + second_occurrence
The actual_index
variable will now hold the index of the second occurrence within the original string.
Step 3: Repeat for Further Occurrences
If you need to find the third, fourth, or any further occurrences of the substring, you can repeat the above steps. You’ll need to redefine the substring each time, using the actual index of the previous occurrence as the starting index for your slice.
With these techniques, you can efficiently locate multiple occurrences of a substring within a string using Python.
Advanced Techniques for Finding Multiple Occurrences
If you need to find multiple occurrences of a substring within a string, you can use some advanced techniques to make your code more efficient.
Here are a few options:
- Regular Expressions: Regular expressions, or regex, can be used to search for patterns within a string. They are extremely powerful and flexible, but can also be complex to work with. In Python, you can use the
re
module to perform regex operations. Here’s an example:import re
string = "The quick brown fox jumps over the lazy dog."
result = re.findall("the", string, re.IGNORECASE)
print(result)
->['the', 'the']
- Custom Functions: If your requirements are more specific, you may want to create a custom function to search for multiple occurrences. Here’s an example:
def find_occurrences(string, substring):
occurrences = []
start = 0
while True:
start = string.find(substring, start)
if start == -1:
return occurrences
occurrences.append(start)
start += 1
string = "The quick brown fox jumps over the lazy dog."
result = find_occurrences(string, "the")
print(result)
->[0, 31]
These are just a few examples of the advanced techniques you can use to find multiple occurrences of a substring in Python. Consider your specific requirements and choose the method that best fits your needs.
Frequently Asked Questions (FAQ)
Are you still struggling to find the second occurrence of a substring in Python? Don’t worry; we’ve compiled a list of frequently asked questions to help you.
Whether you’re a beginner or an experienced programmer, these tips will be useful.
Q: What if the substring occurs only once?
If the substring occurs only once, you can use the find() method to locate it. However, if you’re unsure whether the substring occurs multiple times, you can still use the techniques discussed in this article.
If you only need to find the first occurrence, you can stop there; otherwise, you can search for subsequent occurrences.
Q: Can I use regular expressions to find the second occurrence?
Absolutely! Regular expressions are a powerful tool for pattern matching and can be used to find multiple occurrences of a substring within a string.
However, if you’re dealing with a simple use case, using the find() method and slicing techniques may be more efficient.
Q: How can I optimize my code for performance?
One way to optimize your code is to avoid unnecessary loops or iterations. Instead, you can use built-in methods or functions that are designed for efficient searching or manipulation of strings. Additionally, you can consider using external libraries that are optimized for specific tasks.
Q: Can I search for a substring using a custom function?
Yes, you can define a custom function that accepts a string and a substring as arguments and returns the index of the second occurrence (if it exists). This approach can be useful if you need to perform additional processing or validation on the string or if you want to encapsulate the search logic in a reusable function.
We hope these FAQs have provided some useful insights and tips for finding the second occurrence of a substring in Python. Remember, practice makes perfect, so don’t be afraid to experiment and try out different techniques until you find the one that works best for your specific use case.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.