If you’re working with data in Python, you may encounter a scenario where you need to download a CSV file from a URL.
Luckily, this process can be achieved in just a few simple steps.
In this tutorial, we will guide you through downloading a CSV file from a URL using Python and provide some helpful tips.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Prerequisites for Downloading CSV Files in Python
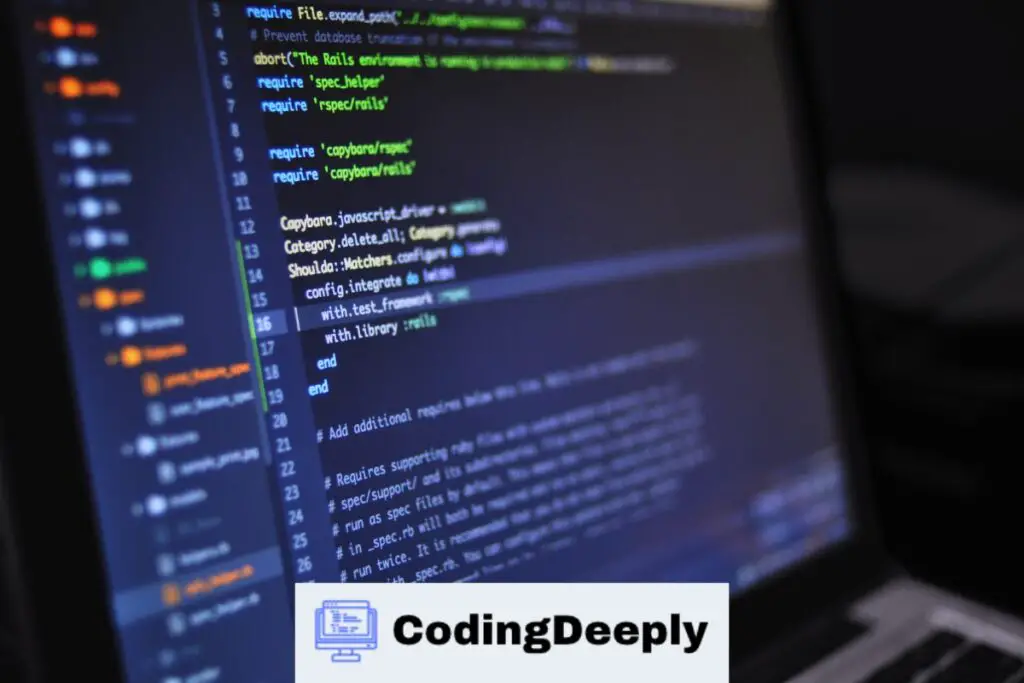
Before we dive into the steps for downloading a CSV file from a URL using Python, it is important to understand Python and CSV files.
Python Prerequisites: You will need to have Python installed on your computer and a basic understanding of coding in Python. If you need to brush up on your Python skills, plenty of online resources are available to help you get started.
CSV Files: CSV (Comma Separated Value) files are a popular data format used for storing and exchanging data between different software applications. These files usually contain tabular data, such as rows and columns of data separated by commas.
Prerequisites for Downloading CSV Files in Python:
- Basic understanding of Python
- Python installed on your computer
- Understanding of CSV files and their format
These prerequisites will enable you to effectively follow the steps for downloading a CSV file from a URL using Python.
Step-by-Step Guide to Downloading CSV from URL
Now that we have covered the prerequisites let’s dive into the step-by-step process of downloading a CSV file from a URL using Python. Here’s what you need to do:
- Import the Required Libraries: The first step is to import the required libraries, including ‘urllib’, ‘csv’, and ‘codecs’.
- Specify the URL and File Path: Next, you need to specify the URL of the CSV file you want to download. You also need to specify the file path where you want to save the CSV file on your local machine.
- Download the File: Now that you have specified the URL and file path, you can use the ‘urllib.request.urlretrieve’ function to download the CSV file from the URL and save it to your local machine.
- Read and Process the File: Once you have downloaded the CSV file, you can read and process the file using the ‘csv.reader’ function. This function allows you to read the CSV file row by row and perform operations on the data.
Let’s take a closer look at each of these steps:
Step 1: Import the Required Libraries
You need to use the ‘import’ statement to import the required libraries. Here’s what you need to type:
import urllib.request import csv import codecs
Step 2: Specify the URL and File Path
To specify the URL and file path, you must create two variables: one for the URL and one for the file path. Here’s what you need to type:
url = 'https://www.example.com/data.csv' file_path = 'C:/Users/YourUserName/Downloads/data.csv'
Step 3: Download the File
To download the CSV file from the URL and save it to your local machine, you need to use the ‘urllib.request.urlretrieve’ function. Here’s what you need to type:
urllib.request.urlretrieve(url, file_path)
Step 4: Read and Process the File
To read and process the CSV file, you need to use the ‘csv.reader’ function. Here’s what you need to type:
with codecs.open(file_path, 'r', encoding='utf-8', errors='ignore') as f: reader = csv.reader(f) for row in reader: print(row)
And that’s it! With these simple steps, you can easily download a CSV file from a URL using Python.
Handling Errors and Exceptions
Sometimes, errors and exceptions may occur during the CSV download process.
Knowing how to handle them effectively in your Python code is important to avoid potential issues.
Common CSV download errors
- HTTPError: This error occurs when the server returns an HTTP error code, such as 404 (not found) or 403 (forbidden).
- URLError: This error occurs when the URL is invalid.
- ConnectionError: This error occurs when the server is unreachable.
- TimeoutError: This error occurs when the server takes too long to respond.
Handling these errors will make your code more robust and prevent it from crashing. Here’s an example of how to handle an HTTPError:
import urllib.request import urllib.error try: response = urllib.request.urlopen(url) except urllib.error.HTTPError as e: print('Error code: ', e.code) except urllib.error.URLError as e: print('Reason: ', e.reason)
Exceptions
Exceptions can also occur during the CSV download process. These are errors that occur within your Python code. There are several built-in exception types in Python that you can use to handle these errors, such as ValueError, TypeError, and IndexError.
Here’s an example of how to handle a ValueError exception:
try: date_format = '%m/%d/%Y' date_string = '01/01/2021' date_obj = datetime.datetime.strptime(date_string, date_format) except ValueError: print('Incorrect data format, should be MM/DD/YYYY')
By handling exceptions effectively, you can avoid potential issues in your code and ensure a smoother CSV download process.
Best Practices and Additional Tips
Now that you have a basic understanding of downloading a CSV file from a URL using Python, let’s explore some best practices and tips to optimize your code.
1. Check Your Internet Connection
Before initiating the download, it’s important to check your internet connection. Slow or unstable internet connections can cause the download to fail or take longer than expected.
2. Use a Robust Library
Python offers several libraries to download CSV files from a URL, including the built-in urllib module and the third-party requests module. It’s recommended to use a robust library that offers additional functionalities, such as error handling and HTTPS support.
3. Handle Errors and Exceptions
As mentioned in the previous section, errors and exceptions may occur while downloading a CSV file from a URL. It is crucial to handle them effectively to prevent the program from crashing. Some common errors include connection timeouts, invalid URLs, and missing files.
- Use the try-except block to catch exceptions and raise meaningful error messages.
- Use the requests module’s status_code attribute to check the server’s response and raise errors accordingly.
4. Optimize Your Code
Optimizing your code can result in faster downloads and smoother performance. Here are some tips to optimize your Python code:
- Use multithreading to download multiple files simultaneously and speed up the process.
- Use the with statement to close the file and connection objects automatically.
- Use the CSV module to read and write CSV files efficiently.
Following these best practices and tips can ensure a more efficient and reliable CSV download process using Python.
Frequently Asked Questions (FAQ)
In this final section, we will address some common questions about downloading CSV files from a URL using Python.
Q1: What is a CSV file, and why is it popular?
A: CSV stands for Comma Separated Values, a file format used for storing and exchanging data between different software applications. The popularity of CSV files is attributed to their simplicity, flexibility, and compatibility with various data management tools.
Q2: Can I modify the file before downloading it?
A: Yes, you can modify the CSV file using Python before downloading it from the URL. You can use various Python libraries, such as Pandas and csv, to manipulate the file’s content and structure.
Q3: How can I download multiple CSV files at once?
A: You can download multiple CSV files at once by using Python to automate the process. You can create a loop to visit each URL and download the corresponding CSV file.
Q4: What should I do if the download stops or fails?
A: If the download stops or fails due to network issues or other errors, you can catch the exception in your Python code and retry the download after a specific time interval. You can also verify the URL and the file size before initiating the download.
Q5: Can I download CSV files from password-protected URLs using Python?
A: Yes, you can download CSV files from password-protected URLs by providing your credentials in the Python code. You can use various Python libraries, such as requests and urllib, to handle authentication and authorization.
We hope these answers have provided useful insights and information for downloading CSV files from URLs using Python. Happy coding!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.