Python deletes an object from memory” – a phrase that might seem daunting but holds the key to efficient programming. Understanding how to manage and free up memory can be your secret weapon in Python.
By diving into this comprehensive guide, you’ll avoid common pitfalls, optimize your code, and elevate your Python skills.
Here’s what’s in store for you:
- Unravel Python’s memory management
- Master practical deletion techniques
- Troubleshoot common memory issues
Ready to unlock the secrets of Python memory management?
Let’s dive in!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Key Takeaways
- Python’s memory management involves the automatic allocation and deallocation of memory.
- The
del
statement in Python is used to delete objects from memory. - Python’s garbage collector uses reference counting to remove unreferenced objects.
- Memory leaks in Python occur when objects are not freed from memory.
- Efficient memory management in Python involves deleting unnecessary objects and controlling garbage collection.
Understanding Python Memory Management
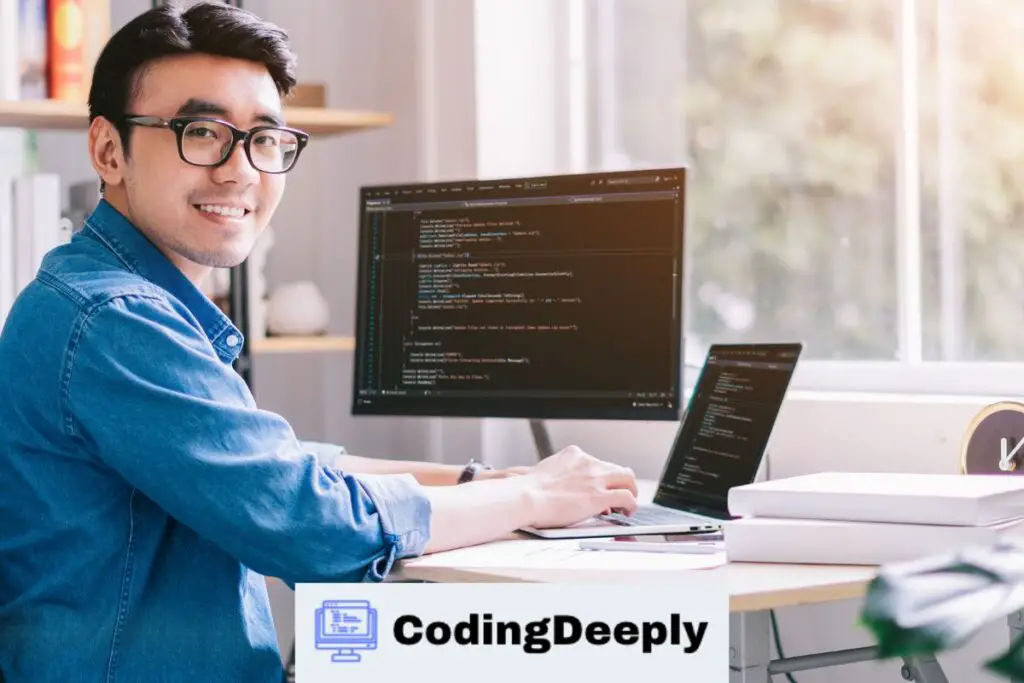
Why Understanding Memory Management in Python is Crucial
In the realm of Python, memory management is a game-changer. The invisible force keeps your programs running smoothly, efficiently, and without hiccups. But, like any powerful tool, it must be understood and wielded correctly.
The Basics of Python Memory Management
Python’s memory management might seem like a complex maze but don’t worry; we’re here to guide you. Let’s break it down:
- Python’s Memory Allocator: This is the wizard behind the curtain. It’s responsible for allocating and deallocating memory for your Python objects.
- Garbage Collection: Python’s garbage collector is like the janitor of your program. It cleans up unused or ‘garbage’ objects, freeing up precious memory.
The Role of Garbage Collection in Python
Python’s garbage collector is a silent hero. It uses a technique called ‘reference counting.’ Each object has a count of the number of references to it. When the count drops to zero, the thing is no longer accessible, and the garbage collector sweeps it away.
Understanding Python Objects and Their Impact on Memory
Python objects are more than just variables and data. They’re the building blocks of your program, and they take up space in memory. When you create an object, Python allocates memory for it.
When you’re done with a thing, it’s crucial to delete it, freeing up that memory for other parts of your program.
In the next section, we’ll dive into the practical side of things. We’ll explore how to delete objects, control the garbage collector, and write memory-efficient Python code. So, buckle up and prepare for a deep dive into Python memory management!
Practical Guide to Deleting Objects from Memory in Python
The ‘del’ Statement: How and When to Use It
Let’s start with the del
statement, Python’s built-in tool for deleting objects. It’s like a magic eraser that makes objects vanish from memory.
Here’s how it works:
# Deleting a variable variable_name = "Hello, Python!" del variable_name # Deleting an item from a list list_name = ["Python", "Java", "C++"] del list_name[1] # This will remove "Java" from the list # Deleting an item from a dictionary dict_name = {"Python": 1, "Java": 2, "C++": 3} del dict_name["Java"] # This will remove the key "Java" and its value from the dictionary
Remember, del
doesn’t immediately free up memory. It just decreases the reference count of the object. When the count hits zero, the garbage collector steps in.
The ‘gc’ Module: Controlling the Garbage Collector
Next up, we have the gc
module. This is your control panel for Python’s garbage collector. You can use it to manually trigger garbage collection, which can be useful in memory-intensive programs.
Here’s how:
import gc # Triggering garbage collection gc.collect()
But remember, use this with caution. Triggering garbage collection too often can slow down your program.
Practical Examples: Deleting Objects from Memory in Python
Now, let’s put theory into practice with some examples:
# Deleting a single object obj = "Hello, Python!" del obj # Deleting objects in a collection list = ["Python", "Java", "C++"] del list[0] # This will remove "Python" from the list # Deleting objects in a class class MyClass: def __init__(self): self.attribute = "Hello, Python!" obj = MyClass() del obj.attribute # This will remove the attribute from the object
Tips and Best Practices for Efficient Memory Management in Python
Finally, let’s wrap up this section with some tips for efficient memory management:
- Understand Your Code’s Memory Footprint: Use tools to track your program’s memory usage.
- Use
del
Wisely: Don’t just delete objects for the sake of it. Understand when it’s necessary and beneficial. - Control Garbage Collection: Use the
gc
module to control when garbage collection happens, especially in memory-intensive programs.
Stay tuned for the next section, where we’ll dive into troubleshooting common issues with Python memory management.
Troubleshooting Common Issues with Python Memory Management
Identifying Memory Leaks in Python
Memory leaks can be sneaky. They’re like tiny holes in a boat, slowly letting water in until, before you know it, you’re sinking.
In Python, memory leaks happen when objects are no longer needed but aren’t freed from memory. Here’s how to spot them:
- Monitor Your Program’s Memory Usage: Keep an eye on your program’s memory usage. If it keeps increasing, you might have a memory leak.
- Use Memory Profiling Tools: Tools like memory_profiler can help you identify memory leaks.
Understanding and Handling ‘MemoryError’
Ever encountered a MemoryError in Python? It’s Python’s way of telling you it’s run out of memory. Here’s what you need to know:
- What Causes MemoryError: This error is raised when a Python operation needs more memory than is available. It’s often due to creating too many objects or large data structures.
- How to Handle MemoryError: If you encounter a MemoryError, you must optimize your code. This could involve deleting unnecessary objects, using more memory-efficient data structures, or reducing the size of your data.
Solutions to Common Memory Management Issues in Python
Now that we’ve identified common issues let’s look at some solutions:
- Delete Unnecessary Objects: Use the del statement to delete no longer needed objects.
- Use Memory-Efficient Data Structures: Choose your data structures wisely. Some are more memory-efficient than others.
- Control Your Garbage Collector: Use the gc module to control when garbage collection happens.
Frequently Asked Questions
What happens if I don’t manage memory in Python?
If you don’t manage memory in Python, you might encounter issues like slow program execution, MemoryError, or even crashes. Understanding and implementing memory management techniques is crucial to ensure your program runs efficiently and avoids these issues.
Can I disable the garbage collector in Python?
You can disable the garbage collector in Python using gc.disable(). However, this is generally not recommended as it can lead to memory leaks and increased memory usage. The garbage collector is crucial in freeing up memory by removing unreferenced objects.
How can I check the current memory usage of my Python program?
You can use memory profiling tools like memory_profiler or tracemalloc to check the current memory usage of your Python program. These tools provide detailed insights into your program’s memory usage, helping you identify potential memory leaks or areas for optimization.
How does Python’s memory management differ from other programming languages?
Python’s memory management is automatic, meaning the language automatically allocates and deallocates memory for you. This differs from languages like C or C++, where you have to assign and deallocate memory manually. Python’s garbage collector also uses reference counting, which is not used in all languages.
What are some common causes of memory leaks in Python?
Common causes of memory leaks in Python include retaining references to no longer needed objects, circular references (where two objects reference each other, preventing the garbage collector from deleting them), and global variables that persist for the program’s lifetime.
Conclusion: Mastering Memory Management in Python
And there you have it! You’ve journeyed through Python memory management, learned to delete objects from memory, and discovered how to troubleshoot common issues.
Remember, mastering memory management is a journey, not a destination. Keep exploring, keep learning, and keep coding.
Your Python skills will thank you for it!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.