As programmers, it’s essential to understand how to comment code. Properly commenting your code can aid in its readability, make it easier to maintain, and help your fellow developers understand your thought process.
In this article, we’ll go over how to comment a block of code in Python.
Python is a high-level programming language with a powerful syntax that is readable and easy to understand. Commenting your code in Python is similar to commenting in other programming languages, with a few differences.
Before we get started on how to comment a block of code in Python, let’s first understand what a code block is. A code block is a group of statements grouped as part of a larger code structure, such as a function or loop. Commenting a code block helps explain what it is doing, increases readability, and makes it easier to maintain.
So, let’s get started on how to comment on a block of code in Python.
Key Takeaways
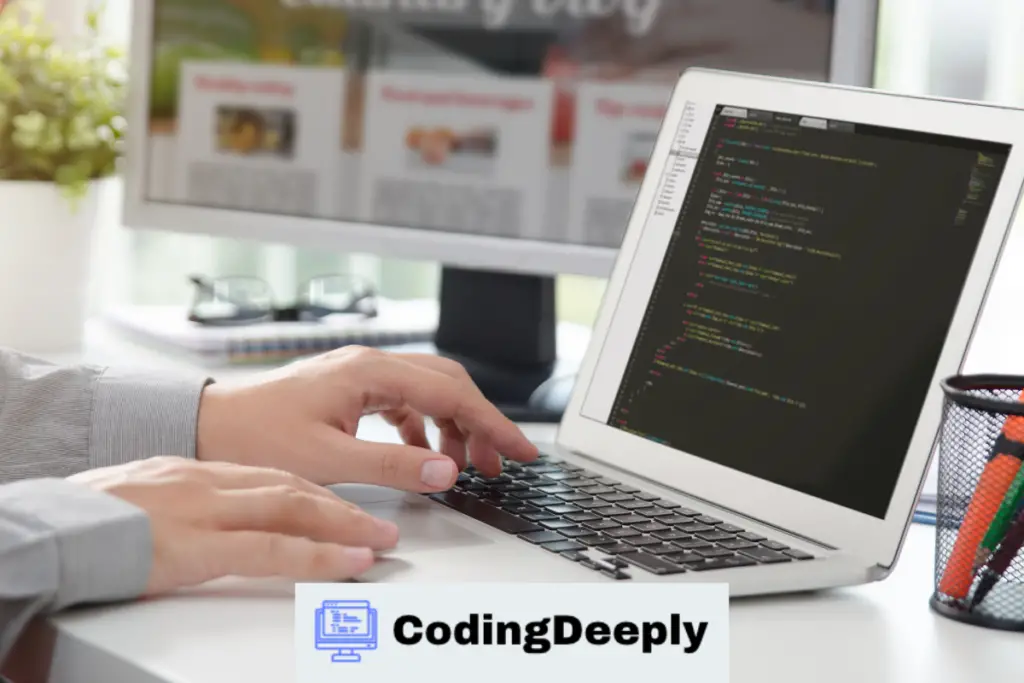
- Commenting your code in Python is important for readability and maintenance.
- A code block is a group of statements in a larger code structure.
- Commenting a code block helps explain what it is doing, increases readability, and makes it easier to maintain.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Python Comment Syntax for Block Commenting
Commenting code is essential to make it more readable and understandable. It can also help others comprehend your code and make modifications in the future.
Python provides several options for commenting code, including block commenting. In this section, we’ll go over the syntax for block commenting in Python.
Python Comment Syntax for Block Commenting
When it comes to block commenting, Python doesn’t have a specific syntax. However, programmers can use docstrings to create block comments. A docstring is simply a string that is used to document a Python module, function, class, or method.
Example:
""" This program performs addition and subtraction of two numbers. """ # Add two numbers def addition(a, b): return a + b # Subtract two numbers def subtraction(a, b): return a - b
In the above example, the docstring is a string enclosed in triple quotes. It provides a detailed description of the program’s functionality. Additionally, each function has a comment that briefly explains what it does.
Docstrings can span multiple lines and can be accessed through the __doc__
attribute of the object they document.
While docstrings may not be the official way to block comment in Python, they are widely accepted and used by the community. They are also a great way to document your code and provide context for future modifications.
Different Ways to Comment Code in Python
Now that we know the syntax for block commenting in Python, let’s explore some other ways of commenting code.
Inline Comments
Inline comments are used to add a brief description of a single line of code. They should be placed to the right of the code and start with a # symbol.
Example: x = 5 # initializes variable x with value 5
We should use inline comments sparingly, as code should be self-explanatory in most cases. However, if a particular line of code does require additional explanation, an inline comment can make the code more readable.
Documentation Strings
Documentation strings, or docstrings, are used to document a module, function, class, or method. They should be enclosed in triple quotes, and can span multiple lines.
Example:
def my_function():
“””
This function does some important stuff.
“””
# function code here
Docstrings can be accessed using the __doc__
attribute, and are an important part of creating readable and maintainable code.
Comments for Debugging
Comments can also be useful for debugging purposes. By commenting out lines of code, we can temporarily disable them to see if they may be causing errors.
Example:
# x = 5
x = 10
print(x)
In this example, we have two different values assigned to the variable x
. By commenting out the first assignment, we can test the second assignment to ensure it is working correctly.
By using different commenting techniques in Python, we can make our code more readable, maintainable, and easier to debug.
Best Practices for Commenting Code in Python
While commenting your code is a great way to make it more readable and maintainable, there are several best practices to keep in mind to ensure that your comments are effective:
Keep Comments Concise and Relevant
Comments should be concise and to the point, providing relevant information about what the code does, rather than how it does it. Avoid commenting on obvious code or repeating information that is already visible in the code itself.
Use Clear and Consistent Syntax
When commenting, use clear and consistent syntax to ensure that your comments are easy to read. For block commenting, use triple quotes with consistent indentation, and for inline comments, use the # symbol followed by a space.
Update Comments When Modifying Code
When making changes or modifications to your code, be sure to also update the corresponding comments to reflect the changes. This ensures that the comments remain accurate and helpful for future readers.
Write Comments in English (US)
While it may seem obvious, it’s important to write your comments in English (US) to ensure that they are clear and understandable to anyone reading your code.
Avoid Over-Commenting
While commenting is important, it’s also possible to over-comment your code, making it difficult to read and understand. Use comments only where necessary, and avoid commenting excessively or unnecessarily.
Use Comments for Problem Solving
If you’re struggling with a particular section of code, commenting your thought process can be helpful in problem solving. By writing down your thought process, you can better understand the problem and come up with possible solutions.
By following these best practices, you can ensure that the comments in your Python code are effective and helpful for anyone reading your code.
Conclusion
In conclusion, commenting code in Python is an essential practice that can save you time and headaches in the long run. Adding comments to your code makes it easier for yourself and others to understand what your code does.
In this article, we learned how to comment a block of code in Python and the different ways to comment code. We also discussed best practices for commenting code, such as using descriptive comments and avoiding unnecessary comments.
Remember, commenting on your code is a good programming practice and makes you a better programmer. By adding comments to your code, you can improve the readability and maintainability of your code for yourself and others.
FAQ
Q: How do I comment a block of code in Python?
A: To comment on a block of code in Python, you can use the # symbol to comment each line individually or enclose the block of code in triple quotes (”’) to create a multiline comment.
Q: What is the syntax for block commenting in Python?
A: In Python, you can comment a code block by enclosing it in triple quotes (”’). Anything within the triple quotes will be treated as a comment.
Q: Can I comment multiple lines in Python?
A: Yes, you can comment multiple lines in Python by using the block comment syntax with triple quotes (”’). Any code enclosed within the triple quotes will be commented out.
Q: Are there any other ways to comment code in Python?
A: Apart from block commenting, you can also use inline comments in Python. You can use the # symbol at the end of the line to add an inline comment.
Q: What are some best practices for commenting code in Python?
A: Some best practices for commenting code in Python include using clear and concise comments, commenting complex code sections, avoiding redundant comments, and following a consistent commenting style.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.