Welcome to our comprehensive guide on using Selenium and Python to automate web interactions.
In this article, we’ll focus on one of the most important tasks in web automation: clicking buttons.
By the end of this guide, you’ll be able to master button clicks using Selenium and Python and use your newfound skills to automate various tasks on the web.
Whether you’re a new developer looking to automate repetitive tasks or a seasoned pro looking to enhance your web automation skills, this guide offers something.
We’ll start with the basics, then move on to more advanced techniques for mastering button clicks with Selenium and Python.
Key Takeaways:
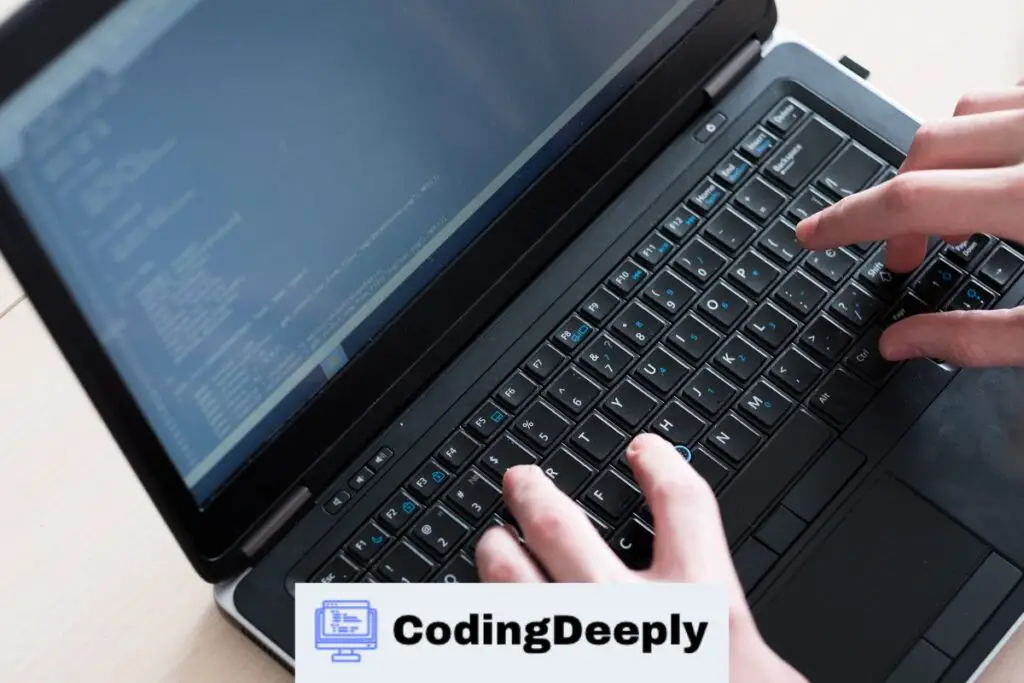
- Selenium and Python are powerful tools for web automation.
- Mastering button clicks is essential for any web automation project.
- This guide will cover basic and advanced techniques for clicking buttons with Selenium and Python.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding Selenium and Button Clicks
Welcome to the world of web automation using Selenium and Python. In this section, we’ll provide an overview of Selenium and explain how it can interact with buttons on web pages.
Selenium is a powerful browser automation tool that allows developers to automate web application testing. With Selenium, we can simulate user interactions including clicking buttons, filling out input fields, and navigating web pages.
Buttons are an essential part of any web page. They’re used to trigger actions, submit forms, or navigate to other pages. Clicking buttons with Selenium is a crucial skill developers must master to create efficient and reliable web automation scripts.
Understanding Selenium and Button Clicks
Regarding button clicks with Selenium and Python, there are several methods and events to consider. The click() method is the most commonly used event to simulate a button click. You can also use the submit() method to simulate a form submission triggered by a button.
To click a button, you need to locate it first. You can search for a button’s id, class, xpath, or other attributes. Once you’ve located the button, you can use the click() method to perform the click action.
It’s important to note that not all buttons are clickable. Some buttons may require additional steps or events before they become clickable. For example, some buttons may be disabled until a user performs a specific action or fills out a form field.
Summary
Understanding Selenium and button clicks is crucial for web automation developers. With Selenium and Python, we can simulate user interactions and automate repetitive tasks quickly and easily.
By mastering the techniques outlined in this section, you’ll be well on your way to becoming a proficient web automation developer.
Clicking Buttons with Selenium in Python
Now that we grasp the basics let’s delve deeper into the process of clicking buttons with Selenium in Python. To click a button, we must first locate it on the webpage. Selenium offers different strategies to locate buttons, such as by ID, class, name, or XPath.
For example, to locate a button by ID, we can use the find_element_by_id()
method:
button_element = driver.find_element_by_id("button_id")
Once we have located the button, we can execute a click action using the click()
method:
button_element.click()
It’s worth noting that some buttons may have different types, such as submit, reset, or image. In these cases, we may need to handle the button type accordingly.
For instance, to submit a form, we can use the submit()
method instead of click()
.
Another important consideration is how to handle dynamic web pages.
Sometimes, the button we want to click may not be immediately available, or some elements on the page may need to load first. In these scenarios, we can use the WebDriverWait()
class to wait for an element to become clickable:
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.by import By
wait = WebDriverWait(driver, 10)
button_element = wait.until(EC.element_to_be_clickable((By.ID, "button_id")))
button_element.click()
As you can see, we pass the ID of the button as a tuple argument to element_to_be_clickable()
, which returns the button element once it becomes clickable within the given timeout (10 seconds in this example).
By mastering these techniques, you can confidently perform button clicks in Selenium Python and automate various web tasks efficiently.
Examples of Clicking Buttons with Selenium in Python
Now that you understand the basics of clicking buttons with Selenium in Python, let’s look at some practical examples.
Example 1: Clicking a Button by ID
To click a button using its ID, you can use the find_element_by_id()
method of the webdriver
class. Here’s an example:
button = driver.find_element_by_id("myButton")
button.click()
This code will find the button with the ID “myButton” and click on it.
Example 2: Clicking a Button by Class Name
You can also click a button using its class name. To do this, use the find_element_by_class_name()
method. Here’s an example:
button = driver.find_element_by_class_name("myButtonClass")
button.click()
This code will find the button with the class name “myButtonClass” and click on it.
Example 3: Clicking a Button by XPath
Another way to click a button is by using its XPath. To do this, use the find_element_by_xpath()
method. Here’s an example:
button = driver.find_element_by_xpath("//button[@id='myButton']")
button.click()
This code will find the button with the ID “myButton” and click on it, using its XPath.
Example 4: Clicking Multiple Buttons
Sometimes, you may need to click on multiple buttons on a webpage. To do this, you can use a loop to iterate through a list of buttons and click on each one. Here’s an example:
buttons = driver.find_elements_by_tag_name("button")
for button in buttons:
button.click()
This code will find all the buttons on the webpage and click on each one using a loop.
These are just a few examples of clicking buttons using Selenium in Python. With these techniques, you can easily automate button clicks on any webpage, saving time and increasing productivity in your web development projects.
Advanced Techniques for Button Clicks in Selenium Python
Now that we have covered the basics of button clicks using Selenium Python, let’s explore some advanced techniques to help you take your web automation skills to the next level.
Waiting for Elements to Become Clickable
One of the most common challenges in web automation is dealing with pages that load slowly or contain elements that are not immediately clickable. Fortunately, Selenium provides several tools to help you handle these situations.
One useful technique is to use explicit waits, which allow you to wait for specific elements to become clickable before executing your click action.
For example, you can use the ExpectedConditions class to wait for an element with a certain ID to become clickable:
wait = WebDriverWait(driver, 10)
element = wait.until(EC.element_to_be_clickable((By.ID, 'myButton')))
element.click()
This code snippet creates a WebDriverWait object with a timeout of 10 seconds, and then waits for the element with the ID “myButton” to become clickable using the element_to_be_clickable method of the ExpectedConditions class.
Once the element is clickable, the code executes the click method on the element to simulate a button click.
Handling Multiple Buttons
When dealing with web pages containing multiple buttons, selecting the correct button to click can be challenging. One way to address this issue is to use unique identifiers like IDs or classes to locate your desired button.
However, there may be situations where this is impossible, such as when the buttons have the same classes or labels.
In these cases, you can use more advanced techniques like XPath or CSS selectors to locate the button based on its position on the page or its relationship to other elements. For example, you can use an XPath expression like this to select the second button on a page:
button = driver.find_element_by_xpath("(//button)[2]")
button.click()
This code uses the find_element_by_xpath method to locate the second button on the page using an XPath expression that selects the second button element on the page.
Dealing with Dynamic Web Pages
Another common challenge in web automation is dealing with pages containing dynamic content, such as pages that update in real-time or load different content based on user input.
In these cases, traditional button-click strategies may not work as expected.
One way to handle dynamic pages is to use implicit waits, which instruct Selenium to wait a certain amount before attempting to locate a button or element.
This can give the page enough time to load the necessary content before executing the button click action.
Another approach is to use JavaScript injection to simulate a button click using the execute_script method. This allows you to bypass some of the limitations of traditional button-click strategies and interact with the page in more flexible ways.
By combining these advanced techniques with the basic button-click strategies we covered earlier, you can develop powerful web automation scripts that can handle even the most complex web pages.
Conclusion
In conclusion, mastering clicking buttons in Selenium Python can significantly enhance your web automation skills.
Automating repetitive tasks can save valuable time and increase productivity in your web development projects.
Throughout this article, we have covered the basics of Selenium and button clicks, and advanced techniques for handling buttons and navigating dynamic web pages.
We have also provided practical examples for clicking buttons using Selenium in Python.
As you continue exploring the world of web automation, we encourage you to experiment with different strategies and techniques and to customize your approach based on your specific needs and requirements.
By leveraging the power of Selenium and Python, you can streamline your workflow, reduce manual errors, and achieve your web development goals more efficiently.
We hope this article has provided you with the necessary insights and knowledge to get started with web automation using Selenium Python.
FAQ
Q: What is Selenium?
A: Selenium is a popular open-source framework used for automating web browsers. It provides a platform-independent API for interacting with web elements and automating various tasks.
Q: How can I click buttons using Selenium and Python?
A: To click buttons using Selenium and Python, you can use the click()
method provided by the Selenium WebDriver. This method allows you to simulate clicking a button on a web page.
Q: What are some common strategies for locating buttons on a webpage?
A: Some common strategies for locating buttons on a webpage include using the button’s ID, class name, or XPath. You can use these strategies to identify and interact with buttons using Selenium in Python uniquely.
Q: How can I handle multiple buttons on a webpage?
A: To handle multiple web buttons, you can use techniques like finding a parent element and then locating the buttons within it using relative XPath or CSS selectors. This allows you to interact with specific buttons based on their position or attributes.
Q: How can I wait for a button to become clickable?
A: You can use the Selenium WebDriver’s built-in wait functionality for a button to become clickable. This ensures that the button is fully loaded and ready for interaction before attempting to click it.
Q: Can I automate button clicks on dynamic web pages?
A: Yes, Selenium can automate button clicks on dynamic web pages. Using techniques like implicit or explicit waits, you can handle dynamically changing elements and ensure that your button clicks are performed accurately.
Q: What are the benefits of mastering button clicks in Selenium Python?
A: Mastering button clicks in Selenium Python allows you to automate repetitive tasks, increase productivity, and save time in your web development projects. By leveraging the power of Selenium and Python, you can efficiently interact with buttons and automate various actions on web pages.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.