In Python, we use both classes and def to write programs. However, they serve different purposes and have distinct characteristics.
In this article, we will explore the differences between classes and def, and how they relate to object-oriented programming in Python.
It is important to understand the differences between classes and def in Python, as they are essential components of the language syntax.
We can write more efficient and maintainable code by understanding how they work.
Key Takeaways
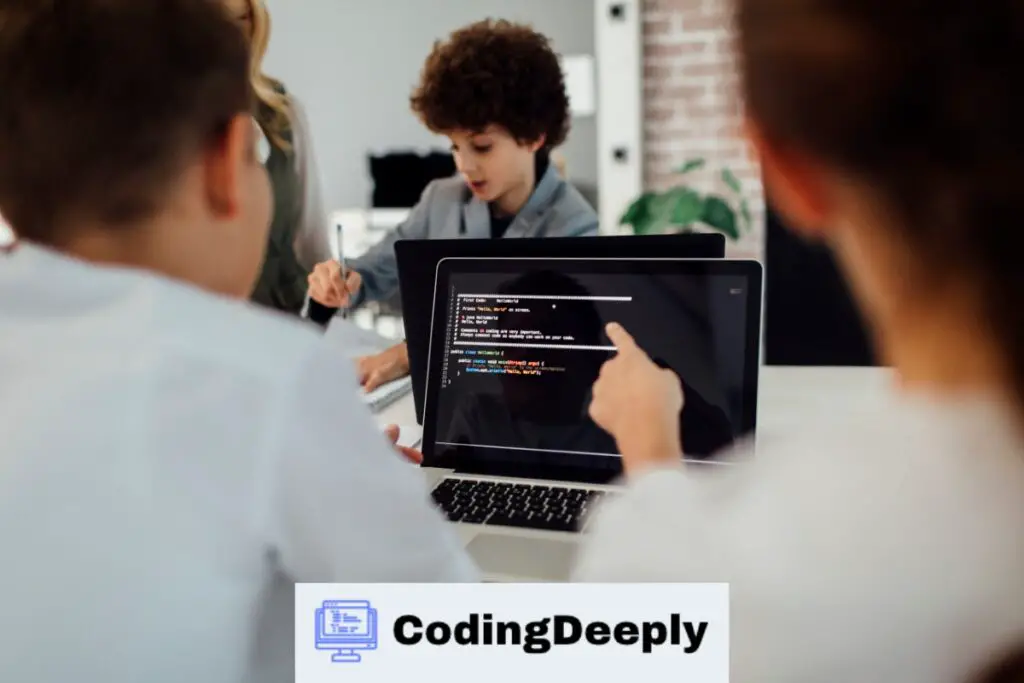
- Classes and def serve different purposes in Python.
- Functions are standalone blocks of code that operate on data, whereas classes define the structure and behavior of objects.
- We can create powerful and maintainable code in Python by utilizing both classes and functions.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
What is a Function in Python?
In Python, a function is valuable for dividing your code into smaller, more manageable pieces. These reusable code blocks are defined using the “def” keyword, followed by the function name, parentheses, and a colon.
Functions can accept arguments, perform operations, and return values – making them customizable to specific needs. For example, you can define a function to return the square of a given number:
def square(x):
return x**2
You can then use this function to calculate the square of any number, simply by calling the function and passing the number as an argument:
result = square(5)
print(result) # Output: 25
Functions are also useful for promoting code reusability. By defining a function once, you can call it multiple times throughout your code, without having to rewrite the same code each time.
It’s important to note that functions can be standalone blocks of code that operate on data, separate from any class declarations.
Introducing Classes in Python
Classes are a fundamental concept in object-oriented programming (OOP). In Python, a class is a blueprint for creating objects, which are instances of the class. Classes encapsulate data attributes and define methods that operate on that data.
They provide a structure for organizing related functions and variables, enabling code modularity and reusability.
When defining a class, you use the class keyword followed by the name of the class and a colon. The body of the class is then indented, and you can define attributes and methods within it. For example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print(f’Hello, my name is {self.name} and I am {self.age} years old.’)
In the example above, we define a class called Person. It has two attributes, name and age, and a method called greet. The __init__ method is a special method used to initialize the class’s attributes when an object is created.
Once a class is defined, you can create instances of that class, also called objects. To create an instance of a class, you use the class name followed by parentheses. For example:
person1 = Person(‘John’, 30)
The code above creates an instance of the Person class named person1, with the name ‘John’ and age 30. You can then call the greet method on the object to print a greeting:
person1.greet()
This will output, “Hello, my name is John and I am 30 years old.”
Key Differences Between Classes and Functions
Understanding the difference between classes and functions is crucial in mastering object-oriented programming in Python. While both classes and functions play important roles in Python programming, they have distinct characteristics and serve different purposes.
Functions are standalone blocks of code
Functions in Python are defined with the def keyword and perform a specific task on data, accepting input arguments and returning values. They can be used without creating an instance and can promote code reusability.
Note: Functions are relatively simple to use and often the first step in learning Python programming.
Classes are blueprints for objects with attributes and methods
Classes are defined with the class keyword and are used to create objects based on blueprints that specify their attributes and behavior.
They encapsulate data and provide a structure for organizing related functions and variables, promoting code modularity and reusability.
Note: Classes are fundamental to object-oriented programming and powerful tools for creating complex applications.
Classes require objects to be instantiated before use
In contrast to functions, classes require objects to be instantiated or created before their methods and attributes can be used. Once an object is created, its methods and attributes can be accessed and modified.
- Tip: Classes can be considered a blueprint for a house, while objects are the actual houses built based on the blueprint.
By understanding the key differences between classes and functions, we can use them effectively, creating powerful and maintainable code in Python.
Working with Functions and Classes Together
Functions and classes can work together in Python programming to promote code reusability and modularity. By defining functions within classes, you can create methods that are specific to that class.
This allows for complex logic and organization of code.
For example:
We can define a class called Rectangle with attributes length and width. We can also define a method within the class called area() that calculates the area of the rectangle by multiplying its length and width.
class Rectangle:
def __init__(self, length, width):
self.length = length
self.width = width
def area(self):
return self.length * self.width
We can use this class to create a rectangle object and then call the area() method to calculate its area.
my_rectangle = Rectangle(5, 10)
print(my_rectangle.area()) # Output: 50
By defining the area() method within the Rectangle class, we encapsulate functionality specific to rectangles and promote code reusability.
Benefits of Using Classes and Functions
Combining classes and functions in Python provides various benefits for building modular, reusable, and maintainable code.
Classes enable us to create custom objects with specific attributes and behaviors, while functions allow us to encapsulate functionality and promote code reuse.
Modularity: Using classes and functions, we can create modular code that is easier to read, understand, and maintain. We can break down complex tasks into smaller, more manageable pieces and reuse them in different parts of our code. This approach also enables us to isolate and fix problems more easily.
“Modular programming is a technique that allows complex software to be separated into independent, interchangeable components. This allows developers to focus on one piece of the software at a time and enables code reuse, easier debugging, and quicker development.”
Code reuse: Both classes and functions promote code reuse by allowing us to define functionality once and use it multiple times.
We can define classes that can be instantiated into objects with specific attributes and behaviors, or we can create functions that can be called with different arguments to produce different results.
“Code reuse is the practice of using existing code to create new software. This can save time and resources, reduce errors, and promote consistency across projects.”
Maintainability: Using classes and functions, we can write code that is easier to maintain and update over time. We can isolate and fix problems more easily, change specific parts of our code without affecting others, and ensure project consistency.
This ultimately leads to more efficient and effective software development.
Conclusion
As we have seen, understanding the difference between classes and functions is critical to mastering object-oriented programming in Python. Functions provide a way to encapsulate logic, while classes offer a blueprint for creating objects with their characteristics and behaviors.
By combining both classes and functions, we can build modular, reusable, and maintainable code in Python. Utilizing functions within classes allows us to implement more complex logic and organize our code more effectively.
Furthermore, classes enable us to create custom objects with specific attributes and behaviors, promoting code modularity and reusability. Functions enable us to encapsulate functionality and promote code reuse. By leveraging the power of these two concepts, we can create powerful and maintainable code.
So, whether you are an experienced Python programmer or just starting your journey, it is essential to understand the differences between classes and functions and how to use them effectively. By doing so, you can take advantage of the full potential of Python’s object-oriented programming capabilities.
FAQ
What is the difference between a class and a def in Python?
In Python, a class is a blueprint for creating objects and encapsulates data attributes and methods. On the other hand, a def (function definition) is a block of reusable code that performs a specific task. While functions are standalone blocks of code, classes define the structure and behavior of objects.
What is a function in Python?
In Python, a function is a block of reusable code that performs a specific task. It is defined using the def keyword followed by the function name, parentheses, and a colon. Functions can accept arguments, perform operations, and return values, making them useful for dividing code into smaller, manageable pieces and promoting code reusability.
What are classes in Python?
Classes are a fundamental concept in object-oriented programming (OOP). In Python, a class is a blueprint for creating objects, which are instances of the class. Classes encapsulate data attributes and define methods that operate on that data. They provide a structure for organizing related functions and variables, enabling code modularity and reusability.
What are the key differences between classes and functions in Python?
While both classes and functions are essential in Python, they have distinct differences. Functions are standalone blocks of code that operate on data, whereas classes are blueprints that define the structure and behavior of objects. Functions can be used without creating instances, while classes require objects to be instantiated before using their methods and attributes.
What are the benefits of using classes and functions in Python?
Utilizing both classes and functions in your Python code offers a range of benefits. Classes enable you to create custom objects with specific attributes and behaviors, promoting code modularity and reusability. Functions allow you to encapsulate functionality and promote code reuse. By combining the two, you can create powerful and maintainable code.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.