Welcome to our informative guide on how to check if a number is in a list in Python. This is a common task in programming, and Python offers several efficient solutions to accomplish it.
This section will explore various methods and techniques to check if a number exists in a list.
Whether a beginner or an experienced programmer, this guide will provide you with the knowledge and skills needed to complete this task. So, let’s get started!
Key Takeaways
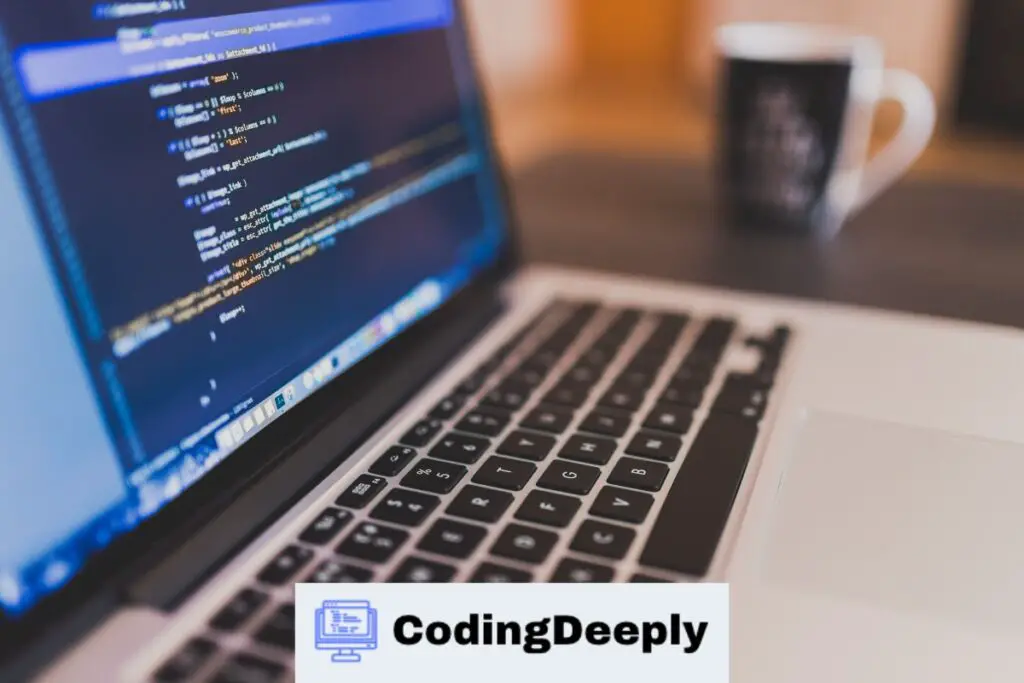
- Several methods exist to check if a number exists in a list in Python.
- We will cover the ‘in’ and ‘not in’ operators, the ‘any’ function, and list comprehension.
- Using these techniques, you can efficiently determine if a number exists in a list and perform further actions based on the result.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Introduction to List in Python
Lists are one of Python’s most versatile and commonly used data structures. A list is an ordered collection of elements, which can be of any type, including integers, strings, and even other lists. Lists are mutable, meaning that their contents can be changed or updated.
To create a list in Python, we enclose its elements in square brackets, separated by commas:
Example:
my_list = [1, 2, "three", [4, 5]]
We can access individual elements of a list using their index. Python uses a 0-based indexing system, meaning that the first element of a list has an index of 0:
Example:
my_list = [1, 2, 3, 4, 5] print(my_list[0]) # output: 1 print(my_list[2]) # output: 3
Lists can contain duplicate elements and have a variable length, meaning we can add or remove elements at any time.
The knowledge of lists will be essential as we explore different methods to check if a number is present in a list using Python.
Using the ‘in’ Operator
One of the simplest and most commonly used ways to check whether a number exists in a list is by using the ‘in’ operator. This operator returns True if the number is in the list and False otherwise.
Let’s take a look at an example:
Example:
numbers = [1, 2, 3, 4, 5]
if 3 in numbers:
print("Number found in the list")
In the above example, we have a list of numbers and check whether the number 3 is present using the ‘in’ operator. Since 3 is present in the list, the output will be “Number found in the list”.
We can use the ‘not in’ operator to check if a number is present in the list. This operator returns True if the number is not found in the list and False otherwise.
Let’s see an example of this:
Using the ‘not in’ Operator
The ‘not in’ operator is another simple and effective method to check if a number is present in a list using Python. As the name suggests, this operator checks if a value is absent in a given list.
Like the ‘in’ operator, the ‘not in’ operator returns a Boolean value: True if the value is not present in the list and False otherwise.
Let’s look at an example:
numbers = [1, 2, 3, 4, 5]
if 6 not in numbers:
print(“6 is not present in the list”)
In this example, we have a list of numbers from 1 to 5. The ‘not in’ operator checks if the number 6 is not in the list. Since 6 is not in the list, the condition is True, and “6 is not present in the list” is printed.
The ‘not in’ operator can also be used with other logical operators like ‘and’ and ‘or’. For example, we can use the ‘not in’ operator with ‘and’ to check if two values are not present in the list:
numbers = [1, 2, 3, 4, 5]
if 6 not in numbers and 7 not in numbers:
print(“Neither 6 nor 7 are present in the list”)
In this example, we added another condition to the ‘not in’ operator to check if the numbers 6 and 7 are not in the list. If both conditions are True, the statement “Neither 6 nor 7 are present in the list” is printed.
Overall, the ‘not in’ operator is a valuable tool to check if a value does not exist in a list. With its flexibility to combine with other logical operators, the ‘not in’ operator is useful in various scenarios.
Using the ‘any’ Function
Another approach to check if a number is present in a list is by using the ‘any’ function. This function takes an iterable as an argument and returns True if any element is True.
We can use this to our advantage by passing a list comprehension that checks if a particular number is present in the list. Here is an example:
my_list = [2, 5, 8, 10, 22]
number = 8
if any(num == number for num in my_list):
print("Number is present in the list")
else:
print("Number is not present in the list")
This code will print “Number is present in the list” since 8 is present.
However, it’s important to note that the ‘any’ function will return True if any element in the iterable is True. This means that the function will still return True if the list contains a non-integer element and we are searching for an integer.
To avoid this, we can use a conditional statement in the list comprehension to ensure that only integers are considered.
Here is an example:
my_list = [2, 'apple', 8, 10, 'banana']
number = 8
if any(isinstance(num, int) and num == number for num in my_list):
print("Number is present in the list")
else:
print("Number is not present in the list")
This code will also print “Number is present in the list” since 8 is present in the list and is an integer.
The ‘any’ function provides a flexible way to check if a number is present in a list and can be useful in scenarios where we need to search for a value in multiple elements of an iterable.
Using List Comprehension
List comprehension is a concise and powerful technique in Python that allows us to create new lists by applying operations to existing lists. We can also use it to check if a number is present in a list.
To do this, we can start by defining a list and then use list comprehension to iterate over each element of the list and check if it is equal to the number we are looking for.
Example: Suppose we want to check if the number 5 is present in a list of numbers. We can use list comprehension as follows:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] check_number = 5 result = check_number in [i for i in numbers] print(result) # Output: True
In this example, we first define the list of numbers and the number we want to check. We then use list comprehension to create a new list that contains all the numbers from the original list.
Finally, we use the ‘in’ operator to check if the number we are looking for is in the new list. The result is a boolean value that indicates whether the number is present.
List comprehension allows us to write compact and readable code, especially with large and complex lists. It is also a flexible technique that is easily adapted to different scenarios.
Conclusion
Checking if a number is present in a list is a common problem in programming, and Python provides several built-in methods to accomplish this task efficiently. In this article, we have explored different techniques to check if a number exists in a list.
We started with the basics of a list in Python and learned how to create and access list elements. Then, we examined the ‘in’ and ‘not in’ operators, which are simple ways to check if a number is present or absent in a list.
Flexibility and Power
The ‘any’ function provides more flexibility, allowing us to check if a number exists in any list element. Additionally, we explored the power of list comprehension in Python, a concise and elegant way to generate new lists based on existing ones.
Using these methods, we can efficiently determine if a number exists in a list and perform further actions based on the result. Whether you are a beginner or an experienced Python programmer, these techniques will help you improve your code and make it more efficient.
Thank you for reading this article on how to check if a number is in a list using Python. We hope you found it informative and useful. Stay tuned for more Python tutorials and tips.
FAQ
How can I check if a number is in a list using Python?
There are several approaches to checking if a number is in a list using Python. Some common methods include using the ‘in’ operator, the ‘not in’ operator, the ‘any’ function, and list comprehension.
What is a list in Python?
A list in Python is a collection of ordered and changeable items. It can contain elements of different data types, such as numbers, strings, and other lists.
How do I use the ‘in’ operator to check if a number is in a list?
To use the ‘in’ operator, write the number followed by the ‘in’ keyword and the list you want to check. It will return True if the number is in the list and False otherwise.
How can I use the ‘any’ function to check if a number is in a list?
The ‘any’ function checks if any element in a list satisfies a certain condition. To use it, you can pass a condition that checks if the number equals any element in the list. It will return True if at least one element satisfies the condition and False otherwise.
How does list comprehension help check if a number is in a list?
List comprehension is a concise and efficient way to create lists in Python. It can also be used to check if a number is present in a list. Using list comprehension, you can create a new list containing only the elements equal to the number you are checking for. If the new list is not empty, the number is present in the original list.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.