Python dictionaries are an essential data structure in Python programming, allowing developers to store and manipulate key-value pairs efficiently.
However, there are some limitations to be aware of when using dictionaries, such as the unique key property. In this article, we will explore the concept of duplicate keys in Python dictionaries and provide insight into best practices for working with them.
Whether you are a beginner or an experienced Python developer, understanding how Python dictionaries handle duplicate keys is important, as it can impact the reliability and efficiency of your code.
Join us in the following sections as we delve deeper into the intricacies of Python dictionaries and explore different approaches to handling duplicate keys.
Key Takeaways:
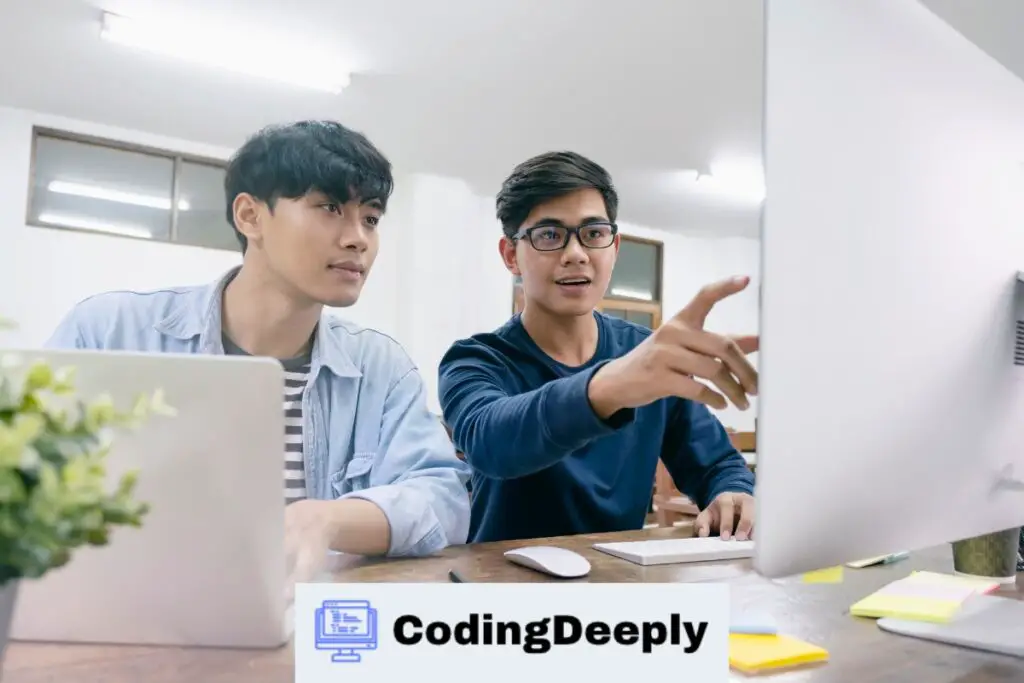
- Python dictionaries do not allow duplicate keys by default
- Alternative approaches, such as using tuples or third-party libraries, can be used to handle duplicate keys
- Following best practices for key handling is crucial to ensure optimal code performance and data integrity
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding Python Dictionaries
Before we delve into duplicate keys in Python dictionaries, let’s take a moment to understand what dictionaries are and how they work in Python programming.
In Python, dictionaries are a collection of key-value pairs. The keys within a dictionary must be unique, while the values can be of any data type, including lists, tuples, and even other dictionaries.
To access a value within a dictionary, you refer to the corresponding key.
For example:
my_dict = {‘key1’: ‘value1’, ‘key2’: ‘value2’, ‘key3’: ‘value3’}
print(my_dict[‘key2’])
The output of the above code will be:
value2
It is important to note that the keys within a dictionary must be unique. Attempting to create a dictionary with duplicate keys will result in an error.
Associating Values with Keys
When assigning values to keys within a dictionary, it is important to remember that the keys are case-sensitive. This means that ‘Key1’ will not be the same as ‘key1’ within a dictionary.
Additionally, while the keys must be unique, the values within a dictionary can be duplicated. For example:
my_dict = {‘key1’: ‘value1’, ‘key2’: ‘value1’, ‘key3’: ‘value3’}
In the above code, ‘value1’ is assigned to both ‘key1’ and ‘key2’ within the dictionary.
Now that we have a basic understanding of Python dictionaries let’s explore the unique key property in more detail.
The Unique Key Property in Python Dictionaries
As we’ve previously mentioned, one of the defining features of Python dictionaries is their unique key property. Each key in a Python dictionary must be unique, meaning no two keys can have the same value.
If an attempt is made to add a new key to a dictionary using an existing key, the dictionary will update the value associated with the existing key.
This unique key property is essential to dictionaries as it ensures efficient key-value lookups and prevents ambiguity.
Without it, retrieving specific values or knowing which key-value pairs are valid within a dictionary would be challenging.
The behavior of dictionaries when duplicate keys are attempted depends on the version of Python.
In Python 3.7 and earlier versions, the last key-value pair added to the dictionary will be stored, while the earlier values will be overwritten.
An error will be raised in Python 3.8 and later, indicating that the dictionary already contains the key.
Handling Duplicate Keys in Python Dictionaries
By default, Python dictionaries do not allow duplicate keys. Attempting to assign a value to an existing key will update the value associated with that key.
However, in certain situations, duplicate keys may be necessary.
Let’s explore some alternative approaches for handling duplicate keys in Python dictionaries.
Using Tuples as Keys
One way to handle duplicate keys is by using tuples as keys. Tuples are immutable and can contain multiple values, making them a suitable alternative for storing related data as keys.
For example:
my_dict = {('John', 'Doe'): 25, ('Jane', 'Doe'): 30}
In this dictionary, the keys are tuples containing both a first and last name, allowing for multiple entries with the same last name.
Using Third-Party Libraries
If tuples are not a suitable alternative, there are third-party libraries available that can handle duplicate keys. One such library is multidict, which allows duplicate keys and values in a dictionary-like structure.
This can be useful for certain data structures, such as graphs, where duplicate keys may be necessary.
Handling Duplicate Key Errors
It is important to note that attempting to assign a value to an existing key in a Python dictionary will result in an error.
To handle this error, it may be useful to catch the error using a try-except block and update the value associated with the key. Alternatively, you can use the setdefault()
method to assign a value to a key only if the key does not already exist in the dictionary.
Using these alternative approaches, we can effectively handle situations where duplicate keys are necessary in Python dictionaries. However, it is important to carefully consider the unique key property and follow best practices to ensure optimal code performance and data integrity.
Best Practices for Key Handling in Python Dictionaries
When working with Python dictionaries, following best practices to ensure optimal code performance and data integrity is important.
Here are some guidelines to keep in mind:
Avoid Duplicate Keys
As mentioned earlier, Python dictionaries do not allow duplicate keys by default. However, ensuring that no duplicate keys are inadvertently added to the dictionary is important. One way to prevent this is to use unique keys such as UUIDs.
Validate Input
When adding keys to a dictionary, it is crucial to validate the input to ensure data integrity. This includes checking for valid data types, avoiding null values, and verifying that the key-value pairs meet any specified criteria.
Use Meaningful Keys
Using meaningful keys can improve the readability and maintainability of code. Rather than using arbitrary keys such as “a”, “b”, “c”, it is recommended to use descriptive keys that accurately reflect the data being stored.
Consider Hashability
Keys in Python dictionaries must be hashable, meaning they can be converted into a unique integer value. Immutable types like numbers, strings, and tuples are hashable, while lists and dictionaries are not.
Keep this in mind when selecting keys for your dictionary.
Choose the Right Data Structure
If you need to store duplicate keys in a dictionary-like object, consider using a defaultdict or a Counter from the collections module. These data structures allow for duplicate keys and provide additional functionality beyond the standard dictionary.
Handle Exceptions
If you are using a third-party library or data source that may contain duplicate keys, it is important to handle any exceptions that may arise. This includes catching KeyError exceptions and implementing fallback methods if a duplicate key is encountered.
By following these best practices, you can ensure that your Python dictionaries are efficient, and error-free, and maintain data integrity.
Conclusion
After exploring the concept of duplicate keys in Python dictionaries, we can conclude that they are not allowed by default. The unique key property exists to maintain data integrity and prevent errors in code execution.
However, there are alternative approaches to handle situations where duplicate keys are needed, such as using tuples as keys or utilizing third-party libraries.
Following best practices when handling keys in Python dictionaries is important to ensure optimal code performance and data integrity. These practices include preventing duplicate keys, validating input, and maintaining consistency within the data structure.
Overall, Python dictionaries are a powerful and flexible tool for storing and manipulating data. Understanding how they work and how to handle keys properly can greatly improve the efficiency and reliability of your code.
FAQ
Can Python Dictionary Have Duplicate Keys?
No, Python dictionaries do not allow duplicate keys by default. Each key in a dictionary must be unique. Attempting to add a duplicate key will result in the value being updated instead of creating a new key-value pair.
What happens if I try to add a duplicate key to a Python dictionary?
If you try to add a duplicate key to a Python dictionary, the value associated with the duplicate key will be updated with the new value. The previous value will be overwritten.
Are there any alternatives to handle duplicate keys in a Python dictionary?
There are alternative approaches to handle situations where duplicate keys are needed. One option is to use tuples as keys instead of single values. Another option is to utilize third-party libraries that provide built-in support for dictionaries with duplicate keys.
How can I prevent duplicate keys in Python dictionaries?
To prevent duplicate keys in Python dictionaries, validating input and ensuring each key is unique before adding it to the dictionary is important. You can use conditional statements and checks to verify the uniqueness of keys before adding them.
What are some best practices for key handling in Python dictionaries?
Here are some best practices for key handling in Python dictionaries:
– Ensure each key is unique to maintain the integrity of the dictionary.
– Validate input to prevent duplicate keys from being added.
– Use descriptive and meaningful keys to enhance code readability.
– Regularly review and update keys to maintain data accuracy.
– Follow Python naming conventions for keys to maintain consistency in the codebase.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.