Can Python be compiled?
The answer is yes… and no. Compiling Python code is a bit different than with other programming languages.
In this article, we’ll explore how Python can be compiled, the benefits of doing so, and the differences between compiled and interpreted Python code.
Key Takeaways:
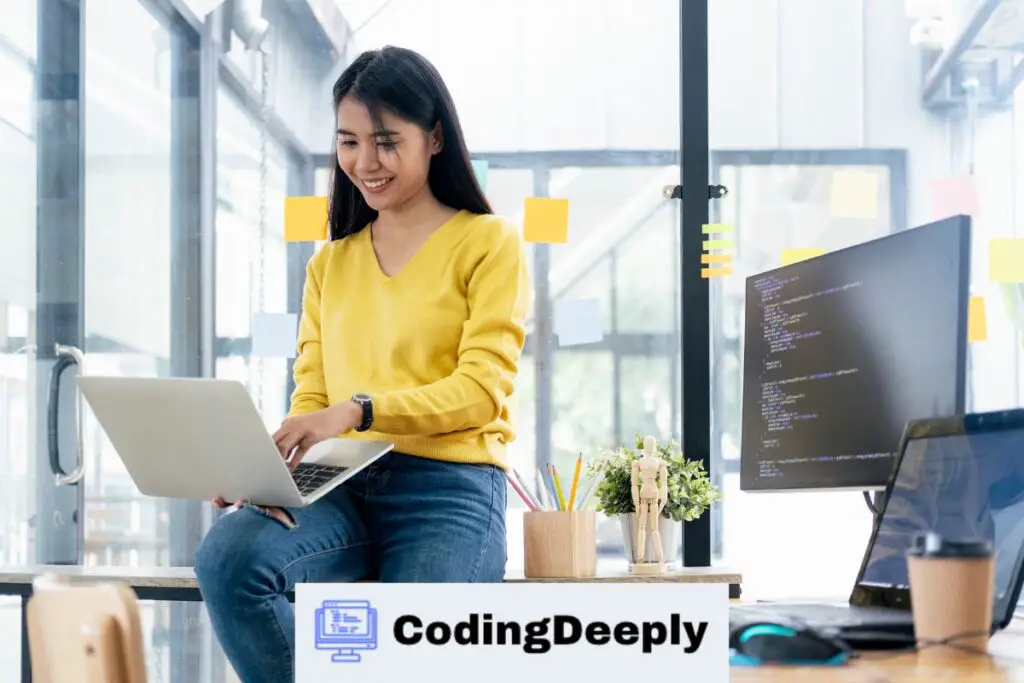
- Python code can be compiled, but the process differs from other programming languages.
- Compiling Python code can offer benefits such as increased speed and optimization.
- Compiled Python code differs from interpreted Python code.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding Python Compilation
Python is an interpreted programming language that runs code directly from source files without compiling.
However, there are situations where compiling Python scripts can provide benefits such as faster execution times and improved code optimization. Let’s dive into the details of Python compilation and explore how it works.
Compiling Python Scripts
The Python compilation process involves converting Python code into bytecode, a low-level code that can be executed on the Python interpreter.
This bytecode is generated by a tool called the Python Compiler, which is included in the standard Python distribution.
Compiling Python code is accomplished using the compile() function, which takes three arguments: the Python code, a filename, and a mode.
The filename and mode are optional parameters, but specifying a filename can be useful for debugging.
Example:
code = "print('Hello, World!')" filename = "hello.py" mode = "exec" compiled_code = compile(code, filename, mode)
The Python Compilation Process
When Python code is compiled, it goes through the following steps:
- The source code is parsed to create an abstract syntax tree (AST).
- The AST is then compiled into bytecode.
- The bytecode is executed on the Python interpreter.
During compilation, the Python compiler checks for syntax errors, ensuring the code will execute correctly once it is run. The compiled bytecode can be stored in files with the .pyc extension, allowing it to be executed faster on subsequent runs.
Now that we know how Python compilation works, let’s explore the different methods of compiling Python code.
Methods of Compiling Python
There are several ways to compile Python code, each with advantages and disadvantages. Let’s explore some of the most popular methods:
Cython
Cython is a popular tool for compiling Python code to C, allowing faster execution. It provides support for static type declarations, making it easier to optimize code.
However, Cython requires a separate installation and can have a steep learning curve for those unfamiliar with C syntax.
Numba
Numba is a just-in-time (JIT) compiler for Python, designed to compile Python code to machine code at runtime. This allows for fast execution without the need for a separate compilation step.
Numba works best with numerical computations and supports CPU and GPU acceleration.
PyPy
PyPy is an alternative Python implementation that uses a just-in-time (JIT) compiler to improve performance. It can be faster than the standard CPython implementation, especially for long-running programs.
However, PyPy does not support all Python libraries and can have compatibility issues with some code.
Nuitka
Nuitka is a Python compiler that translates Python code to C++, allowing faster execution. It supports a wide range of Python features and can generate standalone executables.
Nuitka can be challenging for those unfamiliar with C++ syntax, and performance improvements may vary depending on the code.
Overall, each of these methods has its benefits and drawbacks. It’s essential to choose the right tool for the job and consider factors such as performance, ease of use, and compatibility with existing code.
Python Compilation vs. Interpretation
There are two ways to execute code in the programming world: compilation and interpretation. Compiled code is translated into machine-readable code before it’s executed, while interpreted code is executed directly without translation.
Python can be both compiled and interpreted, but the default implementation of Python is an interpreted language. When we run a Python script, the Python interpreter reads the code line by line, compiles it into bytecode, and then executes the bytecode. This process happens automatically and behind the scenes.
However, we have several options if we want to compile Python code. We can use tools such as PyInstaller, Nuitka, and PyOxidizer to convert Python code into standalone executables that can be run without needing a Python interpreter. The benefit of this approach is that it makes the code faster and more secure.
Python Compilation vs. Interpretation: Which is Better?
The answer to this question depends on what you’re trying to achieve. Interpreted code is generally easier to develop and debug, as changes to the code can be quickly tested without recompilation.
On the other hand, compiled code is generally faster and more secure than interpreted code.
Compiling Python code can be especially beneficial for larger projects that require high performance and for distributing code to users who may not have Python installed on their machines.
However, interpretation may be sufficient for smaller projects or scripts that are only used once.
Ultimately, the choice between compilation and interpretation depends on the specific requirements of your project and the tradeoff between development speed and performance.
Optimizing Python Code with Compilation
Compiling Python code can significantly optimize the performance of your program.
By converting the source code into bytecode, the interpreter can skip the compilation step each time the code is executed, resulting in faster execution time.
The benefits of compilation are particularly evident when dealing with large or complex projects. Compiling can reduce the time it takes to execute code and improve memory usage.
It also allows for creating standalone executable files that can be distributed and run on other computers without needing the Python interpreter.
“Compiling Python code can improve performance in terms of both speed and memory usage. It is an essential tool for optimizing large and complex projects.”
How to Compile Python Code
Compiling Python code involves using the “compileall” module or the “py_compile” module in the Python Standard Library.
- The “compileall” module can compile an entire directory of Python files.
- The “py_compile” module can be used to compile individual Python files.
To compile a Python file using the “py_compile” module, import the module and use the “compile” function:
import py_compile
py_compile.compile(‘filename.py’)
The compiled bytecode will be saved in a file with a “.pyc” extension.
Optimizing with Just-In-Time (JIT) Compilation
JIT compilation is a technique that allows Python code to be compiled at runtime instead of beforehand. This can lead to even faster execution times and improved performance.
One popular JIT compiler for Python is PyPy. PyPy is compatible with most Python code and can provide significant performance improvements for certain types of applications.
However, it’s worth noting that JIT compilation may not always be the best option.
JIT compilation can take additional time during program execution, and certain programs may not see significant performance improvements.
Conclusion
Compiling Python code can be a valuable tool for optimizing your program’s performance.
By converting source code to bytecode, the interpreter can skip the compilation step each time the code is executed, resulting in faster execution time and improved memory usage.
Additionally, using JIT compilers like PyPy can provide even further performance improvements.
Conclusion
In conclusion, Python can be compiled, but the compilation process is not as straightforward as other programming languages. Understanding the compilation process and the available methods can help developers optimize their code and improve performance.
While Python is mainly an interpreted language, there are benefits to compiling certain sections of code. For instance, compiled Python code can run faster and use less memory than interpreted code.
However, Python compilation is not always necessary and should only be used when performance gains outweigh the additional development time.
Overall, Python remains a popular language for beginners and software development experts.
Whether you compile your code or not, Python offers a wide range of tools and libraries, making it a versatile and powerful language for building applications and solving complex problems.
FAQ
Can Python be compiled?
Yes, Python can be compiled. While Python is an interpreted language, it can be compiled to bytecode or machine code for improved performance and distribution.
What is the process of compiling Python scripts?
The compilation process of Python involves converting the human-readable code into a form that the computer can execute. This process includes lexical analysis, syntax analysis, and code optimization.
How can I compile Python code?
There are several methods to compile Python code. To create executable files, you can use tools like PyInstaller, cx_Freeze, or py2exe. Additionally, you can compile Python modules using the built-in compile()
function.
What are the benefits of compiling Python programs?
Compiling Python programs can improve performance by reducing the runtime overhead of interpretation. It can also make it easier to distribute Python applications as standalone executables without needing the Python interpreter.
What is the difference between compiled and interpreted Python?
Compiled Python code is converted into a form that can be executed directly by the computer, while interpreted Python code is executed line by line by the Python interpreter. Compilation can offer faster execution times, while interpretation allows for more interactive development.
How can I optimize Python code with compilation?
Optimizing Python code involves using specialized libraries, profiling tools, and techniques like just-in-time (JIT) compilation. Compiling specific parts of your code can also help improve performance by reducing overhead.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.