Python is a powerful programming language that gives developers a lot of freedom. Even seasoned programmers, nevertheless, occasionally encounter issues that are challenging to fix. Python programmers frequently run into the issue “Can only convert an array of size 1 to a Python scalar.”
When you attempt to convert an array to a scalar, but the array has many elements, this error occurs. We’ll go over various approaches to correcting this mistake in more depth in this blog post.
If this mistake has happened to you before, you are aware of how time-consuming and difficult it may be to fix.
For you to quickly and simply solve the issue, we have created this tutorial. We’ll discuss various approaches to the issue, outline when to utilize each, and offer real-world examples to show how to use each approach.
Let’s begin by examining the issue more closely and its causes.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding the problem: Can Only Convert an Array of Size 1 to a Python Scalar
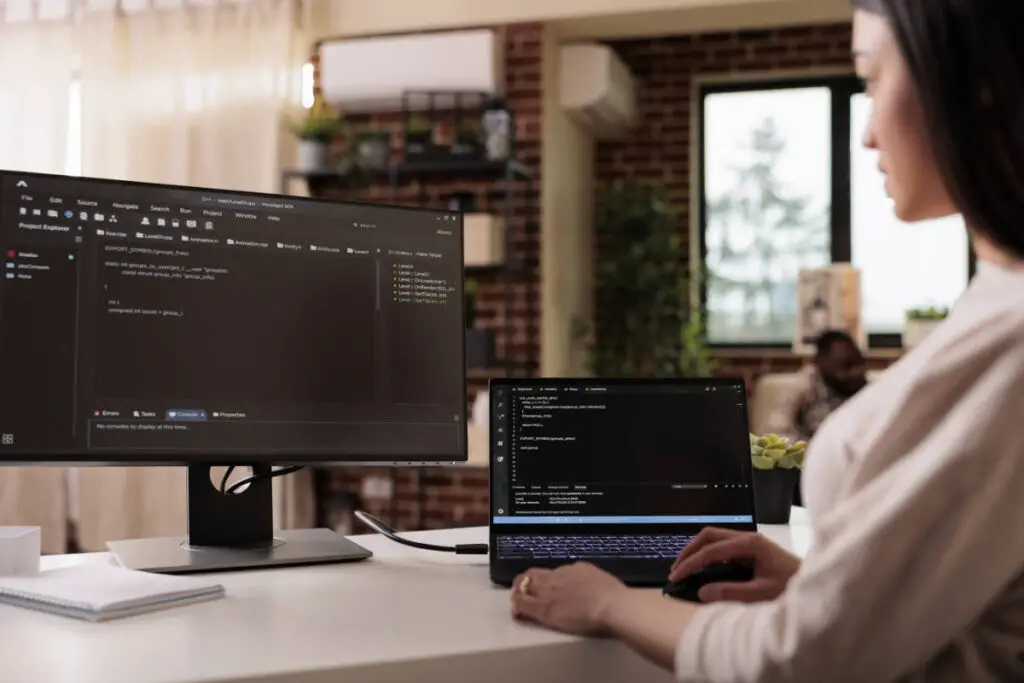
At some point, while working with arrays in Python, you might need to change them to scalar values. For instance, you might need to transform an array to a scalar before doing mathematical operations on it.
You will receive an error notice that reads, “Can only convert an array of size 1 to a Python scalar,” if you attempt to convert an array with more than one element to a scalar.
Python is unable to transform an array with multiple elements to a scalar value, which results in this error. In other words, Python is unaware of which array element you want to turn into a scalar.
Let’s imagine, for illustration, that you have an array of numbers:
numbers = [1, 2, 3, 4, 5]
If you try to use the following code to turn this array into a scalar:
scalar = int(numbers)
Can only convert an array of size 1 to a Python scalar, reads the error message that appears. This is due to Python’s inability to determine which array element should be turned into a scalar.
This error can occur when performing mathematical calculations, producing graphs, or using data science libraries like NumPy, among other things.
Now that we are aware of the issue, let’s look at some potential fixes.
Solution 1: Array indexing
Indexing the array to extract the scalar value is one method for resolving the “Can only convert an array of size 1 to a Python scalar” problem.
Here is how to go about it:
Using the len() function, first determine the array’s length:
numbers = [1, 2, 3, 4, 5] print(len(numbers))
You can easily use indexing to get the value of the first element and then convert the array to a scalar:
numbers = [1, 2, 3, 4, 5] scalar = numbers[0]
It should be noted that, depending on which element of the array you wish to convert, you can use any index to retrieve the scalar value.
Lastly, you can modify the scalar value as necessary:
numbers = [1, 2, 3, 4, 5] scalar = numbers[0] result = scalar * 2 print(result)
Indexing is a quick and efficient way to solve the issue. It only functions, though, provided you are aware of the array element you wish to turn into a scalar.
You might occasionally need to switch to a different approach that can deal with arrays with multiple elements. In the part that follows, let’s examine a different option.
Option 2: Using the numpy library
Using the numpy library is another way to get around the “Can only convert an array of size 1 to a Python scalar” problem.
There are several helpful functions for handling arrays of various sizes provided by the well-liked Python package known as Numpy.
The following describes how to use Numpy to change an array into a scalar:
Import the Numpy library first:
import numpy as np
Then, use numpy to construct an array:
numbers = np.array([1, 2, 3, 4, 5])
You can use the item() function to change the array into a scalar:
scalar = numbers.item()
This code will give the variable scalar the value of the array’s first member, which is (1).
Keep in mind that the array can only have exactly one element for the item() method to work. A ValueError exception will be thrown if the array contains more than one element.
Lastly, you can modify the scalar value as necessary:
numbers = np.array([1, 2, 3, 4, 5]) scalar = numbers.item() result = scalar * 2 print(result)
A more durable solution that can handle arrays of various sizes is to use numpy. To convert the array to a scalar, however, you must import an additional library and utilize a particular method.
You might want to utilize a less complex solution occasionally if it doesn’t call for any additional libraries. In the part that follows, let’s examine a different option.
Option 3: Reshaping the array
Reshaping the array into a scalar is a third way to fix the “Can only convert an array of size 1 to a Python scalar” problem. Here is how to go about it:
Making an array with the desired scalar value first:
scalar_array = np.array([2])
After that, modify the initial array to match the scalar array in shape:
numbers = np.array([1, 2, 3, 4, 5]) numbers = numbers.reshape(scalar_array.shape)
The numbers array will be reshaped using this code to match the geometry of the scalar array. The value of the original array’s first element will be the only element in the generated array.
The item() method can also be used to get the scalar value:
scalar = numbers.item()
This code will give the variable scalar the value of the single number (1) in the numbers array.
Reshaping the array is necessary for this technique, which might be time-consuming if you’re working with huge arrays. It’s also more complicated than the earlier solutions.
Let’s review the major ideas of the blog post in the conclusion, after looking at various fixes for the “Can only convert an array of size 1 to a Python scalar” issue.
Conclusion
We looked at many solutions to the “Can only convert an array of size 1 to a Python scalar” problem in this blog post.
There are various ways to fix the Python error “Can only convert an array of size 1 to a Python scalar,” including indexing the array, utilizing the numpy module, and reshaping the array.
The optimal option depends on the particular use case; indexing is the simplest, numpy is the most reliable, and reshaping is less easy but occasionally beneficial.
It’s critical to select the approach that addresses the issue at hand the most effectively.
FAQ
What does it indicate when you receive the error “Can only convert an array of size 1 to a Python scalar”?
When you attempt to convert an array to a scalar but the array has many elements, this error occurs. Python isn’t aware of which array element you want to turn into a scalar.
Can only convert an array of size 1 to a Python scalar—how do I fix this?
The array can be reshaped, the numpy library can be used, and indexing the array are a few options. The particular use case determines the appropriate course of action.
How does the “Can only convert an array of size 1 to a Python scalar” bug get fixed? What is the numpy library?
A well-liked Python library for working with arrays is called Numpy. It offers a variety of helpful capabilities for managing arrays of various sizes. Using the item() function offered by numpy is one technique to resolve the “Can only convert an array of size 1 to a Python scalar” problem.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.