This guide will cover converting bytes to an integer in Python.
Python provides multiple techniques for byte-to-int conversion, each with its advantages and use cases.
By the end of this guide, you will have a clear understanding of different methods and be able to choose the most appropriate one according to your programming requirements.
Key Takeaways
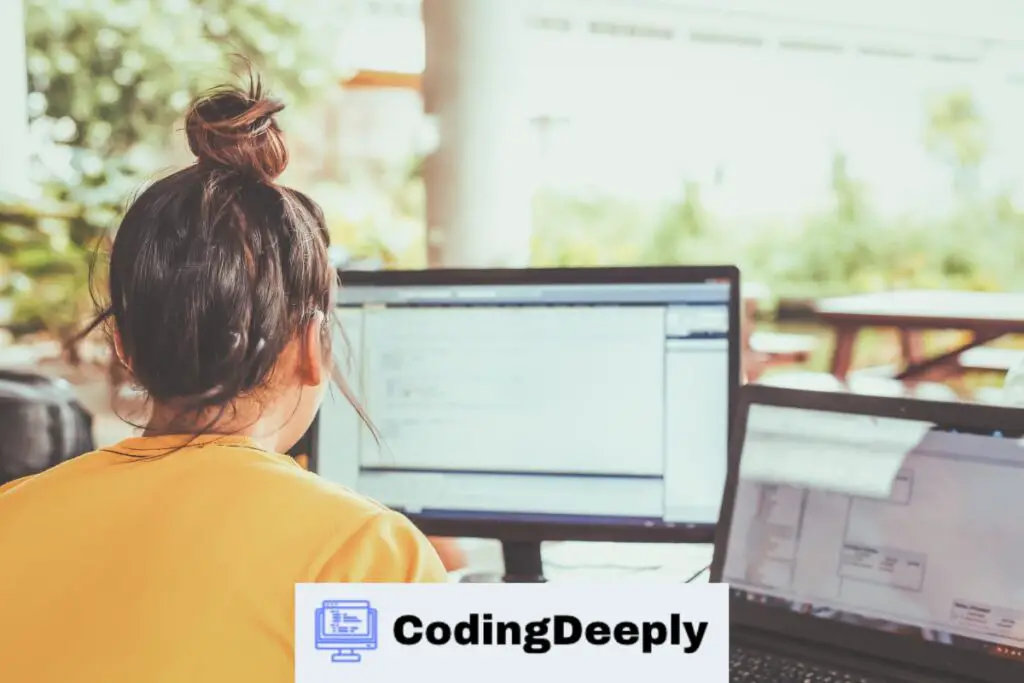
- There are several ways to convert bytes to an integer in Python.
- Some methods covered in this guide include the int.from_bytes() function, unpacking with the struct module, and using the ord() function.
- Understanding the byte size and byte order is crucial for successful conversion.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Converting Bytes to Int in Python
This section will explore various approaches to converting bytes to an integer in Python.
“Bytes to integer conversion in Python requires careful consideration of the byte order and size to ensure accurate conversion. Fortunately, Python provides multiple methods for this process.”
We will first discuss the int.from_bytes() function, which allows us to convert bytes to an integer directly. This method requires us to specify the byte order and the number of bytes to read from the input bytes.
The syntax for using the function is as follows:
int.from_bytes(bytes, byteorder, *, signed=False)
Where:
- bytes: The input bytes to be converted to an integer.
- byteorder: The byte order of the input bytes (little-endian or big-endian).
- signed: Optional Boolean value to specify whether the input bytes represent a signed integer.
Let’s take a look at an example:
bytes_data = b'\x00\x10' int_data = int.from_bytes(bytes_data, byteorder='big') print(int_data)
In this example, we have a two-byte sequence, b'\x00\x10'
, representing the integer value of 16. By specifying the byte order as 'big'
and using the int.from_bytes() function, we can convert the two-byte sequence to the integer value of 16.
Another method to convert bytes to an integer is to use the unpacking technique with the struct module. This method allows us to unpack binary data and quickly convert it to a Python object.
Let’s examine how this method functions.
Using the int.from_bytes() Function
This section will explore the int.from_bytes() function and how it can convert bytes to an integer in Python. This function takes two required arguments: the bytes object, and the byte order of the data.
The byte order argument decides whether the bytes object should be interpreted as big-endian or little-endian, which is essential for proper byte to int conversion.
This argument has two possible values: ‘big’ and ‘little’, which can be specified as a string or using the constant values sys.byteorder == ‘big’ and sys.byteorder == ‘little’.
To use the int.from_bytes() function, create a bytes object containing the data to be converted, then pass it to the function, along with the byte order argument.
The function will return an integer value.
Example:
# Create a bytes object
data = b'\x00\x10'
# Convert bytes to int using int.from_bytes()
result = int.from_bytes(data, byteorder='big')
# Output the result
print(result)
Output:
16
In this example, we created a bytes object with the hexadecimal values of ’00’ and ’10’. We then passed this object to the int.from_bytes() function and the byte order argument set to ‘big’.
The function interpreted the bytes as big-endian and returned the corresponding integer value of 16.
It is important to note that the size of the byte object must match the byte size of the integer type being converted.
For example, if you convert to a 4-byte integer, the byte object should contain 4 data. If the byte size does not match, a ValueError will be raised.
Unpacking with the struct Module
Another method for converting bytes to int in Python is by using the struct module to unpack the binary data. This approach is particularly useful when dealing with binary data of various sizes and endianness.
The first step is to define a format string that specifies the size and byte order of the data.
For example, the format string ‘>I
‘ represents an unsigned integer in big-endian byte order. The ‘>
‘ symbol indicates big-endian byte order, while ‘I
‘ represents an unsigned integer of 4 bytes in size.
Note: If you are unsure of the byte order of your data, you can use the ‘
@
‘ symbol to indicate native byte order, which will automatically detect the byte order of your system.
After defining the format string, you can use the struct.unpack()
function to unpack the binary data into a tuple of values. The function takes two arguments: the format string and the binary data.
Below is an example of how to use the struct module to convert a byte string to an integer:
# Define format string format_str = '>I' # Binary data byte_str = b'\x00\x00\x00\x01' # Unpack binary data int_val = struct.unpack(format_str, byte_str)[0] print(int_val) # Output: 1
You can also handle different byte sizes by changing the format string to match the desired size. For example, the format string ‘>h
‘ represents a signed short integer in big-endian byte order, while ‘>Q
‘ represents an unsigned long long integer in big-endian byte order.
Overall, the struct module provides a flexible and efficient method for converting bytes to int in Python. Its ability to handle various byte sizes and endianness makes it a powerful tool for working with binary data.
Using the ord() Function
This section will discuss using the ord() function to convert bytes to an integer in Python.
The ord() function is a built-in Python function that returns an integer representing the Unicode character. It can also convert a single-byte string, such as a byte, to its corresponding integer value. However, it should be noted that the ord() function can only convert a single byte at a time.
“The ord() function is a quick and easy solution for converting a single byte to an integer in Python.”
The syntax of the ord() function is straightforward. To convert a byte to an integer, pass the byte as an argument to the ord() function.
For example:
byte = b'\x01'
integer = ord(byte)
print(integer) # Output: 1
As shown in the example, the ord() function returns the integer value of the byte, in this case, 1. However, the ord() function cannot handle multi-byte integers, as it only works with single-byte characters.
The ord() function is a quick and easy solution for converting a single byte to an integer in Python. For more reliable and robust results when performing multi-byte conversions, it is recommended to use the int.from_bytes() function or the unpacking technique with the struct module.
Conclusion
In conclusion, we have explored various methods to convert bytes to int in Python. By following our step-by-step guide in section one, you should now be equipped with a broader understanding of different techniques to convert bytes to an integer in Python.
The int.from_bytes() function, discussed in section two, is a powerful method with many use cases, and we have clarified its syntax and provided examples to ensure a comprehensive understanding.
Additionally, we explored the unpacking technique with the struct module in section three, which provides flexibility in handling different byte sizes and endianness.
FAQ
How can I convert bytes to an integer in Python?
You can use several methods to convert bytes to an integer in Python. Some commonly used methods include using the int.from_bytes() function, unpacking with the struct module, and using the ord() function.
Each method has its own advantages and use cases so that you can choose the most suitable one based on your programming requirements.
What is the int.from_bytes() function?
The int.from_bytes() function is a built-in Python function that allows you to convert bytes to an integer. It takes two parameters – the bytes object to convert and the byte order. Depending on the desired byte order, the byte order can be specified as ‘big’ or ‘little’.
This function is particularly useful when converting byte arrays of known sizes.
How can I use the int.from_bytes() function to convert bytes to an integer?
To use the int.from_bytes() function, you must pass the bytes object and the byte order as parameters. For example, if you have a bytes object named ‘byte_data’ and want to convert it to an integer using big-endian byte order, you can use the following syntax: ‘integer = int.from_bytes(byte_data, byteorder=”big”)’.
This will convert the bytes object to an integer and assign it to the ‘integer’ variable.
How can I convert bytes to an integer using unpacking with the struct module?
To convert bytes to an integer using unpacking with the struct module, you must define a format string specifying the byte order and size.
The format string can be created using the ‘>’ or ‘<‘ character to indicate big-endian or little-endian byte order, followed by a letter representing the desired byte size (e.g., ‘i’ for 4-byte integer).
Once you have the format string, you can use the struct.unpack() function to unpack the bytes object and convert it to an integer.
What is the ord() function, and how can it convert bytes to an integer?
The ord() function is a built-in Python function that returns the Unicode code point of a character. While it is not specifically designed to convert bytes to an integer, it can be used in certain scenarios where the byte represents a single character.
You can obtain the corresponding integer value by calling the ord() function on the byte. However, this method has limitations when dealing with multi-byte values or byte sequences that do not represent characters.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.