As Python developers, we’ve all been there – staring at an error message that reads “break is outside loop python”.
This error occurs when the break statement, used to exit a loop prematurely, is placed outside the loop structure.
In this article, we will explore the common mistakes associated with using the break
statement outside of a loop in Python. We’ll explain its functionality and why it should be used inside a loop. Additionally, we’ll provide solutions and best practices for avoiding this error and optimizing loop structures.
Key Takeaways
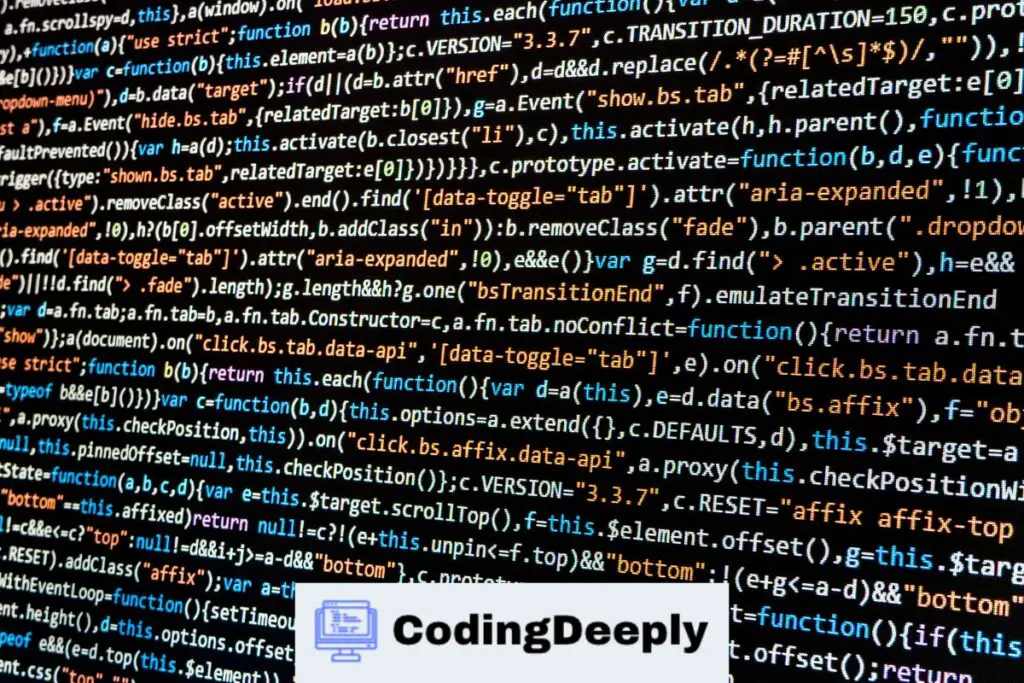
- The
break
statement is used to exit a loop in Python prematurely. - Placing the
break
statement outside of a loop structure will result in the “break is outside loop python” error. - Correct usage of the
break
statement within loops is essential for avoiding errors and maintaining program flow.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding the Break Statement in Python
Before we dive into how to avoid common mistakes, let’s first understand what the break statement does in Python.
The break statement allows us to exit a loop prematurely. When the break statement is encountered within a loop, the loop is immediately terminated, and program execution continues with the next statement after the loop.
Here’s an example of using the break statement within a for loop:
fruits = ["apple", "banana", "cherry"] for x in fruits: if x == "banana": break print(x)
In this example, the loop will terminate when the value of x equals “banana”, and the program will print only “apple” before continuing on to the next statement.
It’s important to note that the break statement should only be used within loops. Using the break statement outside of a loop, as discussed in the next section, can lead to errors and unwanted program behavior.
Common Mistakes: Break Statement Outside Loop
As we have discussed, the break statement in Python allows us to exit a loop prematurely. However, using the break statement outside a loop is a common mistake that can lead to program errors and unexpected behavior.
When the break statement is used outside a loop, the interpreter generates an error indicating that the “break is outside loop python”.
This error occurs because the break statement only works when used within a loop. Using the break statement outside of a loop disrupts the flow of the program and can cause it to terminate unexpectedly.
“Using the break statement outside of a loop is a common mistake that can lead to program errors and unexpected behavior.”
To illustrate this error, consider the following code snippet:
num = 10 while num > 0: num -= 1 break print("The loop has ended")
When executed, this code will result in a “break is outside loop python” error because the break statement is outside the while loop.
To avoid this error, the break statement must be used within the loop’s body, as demonstrated below:
num = 10 while num > 0: num -= 1 if num == 5: break print("The loop has ended prematurely")
In this revised code snippet, the break statement is used inside the while loop to terminate it early when the value of num reaches 5.
In conclusion, using the break statement outside a loop is a common mistake that can cause program errors and unexpected behavior. To avoid this error, always ensure that the break statement is used inside the loop’s body to ensure proper program flow in Python.
Solutions: Correctly Using the Break Statement
Now that we’ve discussed the common mistake of using the break statement outside of a loop in Python let’s explore solutions and best practices for correctly using the break statement.
Proper Loop Structure
To avoid using the break statement outside of a loop, properly structure your loops. Ensure that the break statement is placed within the loop body to only exit the loop when the correct condition is met.
One way to achieve this is by using a conditional statement, such as an if statement, to control when the break statement is executed.
“A common problem with break statements is that they tend to be placed outside of loops, which results in syntax errors and unexpected behavior in your Python program.”
Alternative Loop Control Statements
While the break statement is a useful tool for exiting loops prematurely, it’s not the only loop control statement available in Python. Two additional control statements are continue and pass.
- The continue statement skips over the rest of the loop body and moves on to the next iteration.
- The pass statement is a placeholder that does nothing but is used when a statement is required syntactically.
By using these additional statements in combination with the break statement, you can gain more control over loop execution and ensure that your program’s logic is correct.
Best Practices
Finally, let’s discuss some best practices for using the break statement in Python:
- Only use the break statement when it’s necessary to exit a loop prematurely.
- Ensure that the break statement is used within the appropriate loop structure.
- Use descriptive variable names and comments to clarify the loop’s purpose and the break statement’s use.
By following these best practices, you can improve the readability and maintainability of your code while avoiding common mistakes.
Breaking Out of a Loop: Examples and Techniques
In Python programming, breaking out of a loop prematurely means to exit the loop. There are many scenarios where breaking out of a loop is necessary, such as when a certain condition is met or when a user inputs a specific value.
Here, we will explore various examples and techniques for breaking out a Python loop.
Breaking out of a loop using the break statement
The simplest way to break out of a loop is by using the break statement. When the interpreter encounters a break statement within a loop, it immediately exits it and moves on to the next line of code.
Example:
for i in range(10): if i == 5: break print(i) Output: 0 1 2 3 4
In this example, the loop is set to iterate through the range of numbers from 0 to 9. However, when i reaches 5, the break statement is executed, causing the loop to terminate prematurely. As a result, only the values from 0 to 4 are printed.
Breaking out of nested loops
Sometimes, it may be necessary to break out of a loop that is nested within another loop. In this case, the break statement will only exit the innermost loop. To break out of multiple nested loops, we can use a flag variable and the break statement as shown below:
Example:
flag = False for i in range(5): for j in range(5): if j == 3: flag = True break print(i, j) if flag: break Output: 0 0 0 1 0 2 1 0 1 1 1 2 2 0 2 1 2 2 3 0 3 1 3 2
In this example, the outer loop iterates through numbers from 0 to 4, while the inner loop iterates through the same range. When j equals 3, the flag variable is set to True, and the inner loop is exited using the break statement.
The outer loop then checks the status of the flag variable and, if True, exits the loop as well.
Breaking out of a loop using the else statement
Python also provides an else statement that can be used with the break statement to execute a block of code when a loop is exited normally (i.e. without using a break statement). This can be useful for detecting when a loop has iterated through all its elements. Here’s an example:
Example:
my_list = [1, 2, 3, 4, 5] for i in my_list: if i == 6: break else: print("The loop has iterated through all elements in the list.") Output: The loop has iterated through all elements in the list.
In this example, the loop iterates through the my_list variable, but the break statement is not executed since 6 is not in the list.
As a result, the else statement is triggered, printing a message indicating that the loop has iterated through all elements in the list.
Breaking out of a loop is an important technique in Python programming, and when used correctly, it can greatly enhance program control and efficiency.
Solutions: Correctly Using the Break Statement
To avoid the “break is outside loop Python” error, it’s important to use the break statement correctly within a loop. Here are some tips for proper usage:
- Ensure that the break statement is used within the appropriate loop structure.
- Use a conditional statement to check the condition under which the loop should be broken.
- Consider using other loop control statements, such as continue or pass, along with the break statement for more complex program logic.
By following these best practices, you can use the break statement effectively while avoiding common errors in your Python programs.
“Using the break statement correctly within a loop not only prevents errors, but it also leads to more readable and efficient code.”
Proper loop management is essential for writing high-quality Python programs. By effectively using loop control statements like a break, you can optimize program logic and increase efficiency. Here are some additional tips for optimizing loop structures in Python:
- Eliminate unnecessary iterations by breaking out of a loop as soon as the desired result is achieved.
- Minimize the number of nested loops, which can result in slower program execution.
- Use list comprehensions or generator expressions instead of traditional loops for improved performance.
Remembering these tips, you can optimize your loop structures and write more efficient Python programs.
Conclusion
In conclusion, avoiding common mistakes, such as using the break statement outside of a loop, is essential in writing maintainable and efficient code in Python. By understanding the functionality of the break statement and correctly using it within loops, we can enhance program control and avoid errors.
We have also explored various techniques for breaking out loops and optimizing loop structures to improve program performance. We can create more effective and scalable programs by implementing these best practices.
Final Tip
One final tip for improving loop management in Python is always to consider the logic of the program and whether the break statement is being used in the appropriate context.
By paying attention to the flow of the program, we can avoid common mistakes and optimize program control.
FAQ
What is the common mistake of using the break statement outside a Python loop?
The common mistake is mistakenly placing the break statement outside a Python loop. This can result in a “break is outside loop python” error, as the break statement is designed to prematurely exit a loop, not to be used outside of one.
What does the break statement do in Python?
The break statement allows us to exit a loop in Python prematurely. When the break statement is encountered within a loop, the program flow is immediately transferred to the next statement outside the loop.
Why should the break statement be used inside a loop?
The break statement should be used inside a loop to control program flow and exit the loop when a certain condition is met. Placing the break statement outside a loop can result in logical errors and unexpected behavior.
How can I avoid the “break is outside loop python” error?
To avoid the “break is outside loop python” error, ensure that the break statement is only used within a loop. Double-check the break statement’s placement and ensure it is inside the intended loop structure.
How do I correctly use the break statement within a loop?
To correctly use the break statement within a loop, place the break statement inside the loop structure, typically within an if statement. This allows the program to exit the loop when a specific condition is met.
Are there any other loop control statements that can be used alongside the break statement in Python?
Yes, other loop control statements can be used alongside the break statement in Python. Examples include the continue statement, which allows you to skip the rest of the current iteration and move to the next one, and the pass statement, used as a placeholder for code that will be implemented later within the loop.
What are some techniques for breaking out of a loop in Python?
Several techniques exist for breaking out of a loop in Python. Examples include using the break statement within an if statement to exit the loop based on a condition, using a boolean flag variable to control the loop’s execution, or using a loop control statement like the continue or pass statement to bypass specific iterations of the loop.
What are the benefits of proper loop management in Python?
Proper loop management in Python, including correct usage of the break statement and other loop control statements, can lead to more efficient and maintainable code. It allows you to control program flow, optimize loop structures, and enhance performance.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.