As developers, we understand the importance of writing code that is efficient and easy to read.
Bold text is an excellent way to make specific sections of your code stand out, allowing you to communicate more effectively.
In this article, we will explore various techniques for formatting text in Python, with a particular focus on bold text. By the end of this article, you’ll be able to enhance the readability of your code and make a stronger impact on your audience.
Key Takeaways:
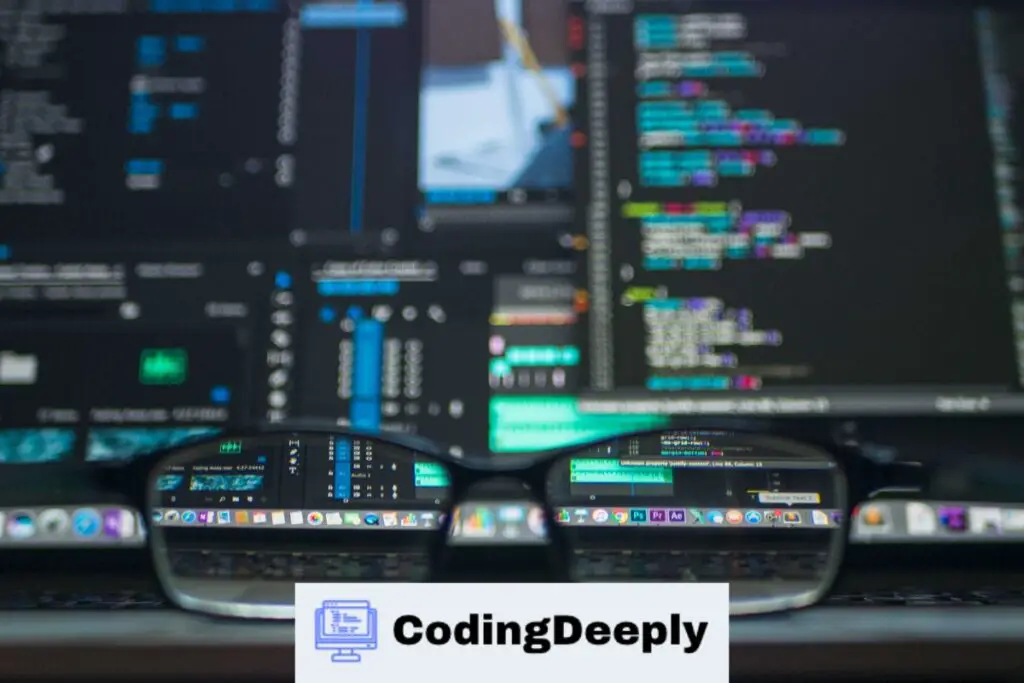
- Bold text formatting is essential in improving code readability.
- Python offers different methods for formatting bold text, including using ANSI escape sequences and third-party libraries.
- By mastering bold text formatting, you can enhance the aesthetics and effectiveness of your code.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding Text Formatting in Python
Before we dive into the exciting world of bold text formatting in Python, let’s first make sure we have a fundamental understanding of text formatting in Python.
When working with text in Python, we can use various methods and functions to manipulate and style it according to our needs. One of the most common methods is through the use of string methods.
Python provides several built-in string methods that allow us to manipulate and format text. For example, the .upper() method converts a string to uppercase, while the .lower() method converts it to lowercase.
The .strip() method removes leading and trailing whitespace from a string, while the .replace() method replaces a specified substring with another string.
We can also use special characters and escape sequences to format text in Python. For instance, the newline character (\n) inserts a line break, while the tab character (\t) inserts a tab space.
Finally, Python’s string formatting syntax provides a powerful way to format text dynamically. This syntax allows us to insert values into placeholders within a string, making it easy to construct complex output strings with variable information.
Python String Formatting
Python uses a powerful string formatting syntax that allows us to insert values into strings dynamically. This syntax is based on placeholders enclosed in curly braces, replaced by the values we provide.
Here’s an example:
name = "John" age = 25 print("My name is {} and I am {} years old".format(name, age))
In the example above, we use curly braces ({}) to indicate where we want to insert values into the string. We pass the values we want to insert into the .format() method, which replaces the placeholders with the values we provide.
The output of this code would be:
My name is John and I am 25 years old
Python’s string formatting syntax offers many additional features, such as specifying the number of decimal places for a floating-point number, padding values with zeros or spaces, and more.
We encourage you to explore this syntax further to discover its full potential.
Using ANSI Escape Sequences for Bold Text
Regarding formatting text in Python, ANSI escape sequences are a popular and platform-independent method. They allow us to control text color, background, and style in the terminal, including bold text.
The syntax for creating bold text using ANSI escape sequences is straightforward. We start with the escape character (“\u001b”), followed by the code for the desired style.
In the case of bold text, we use the code “\[1m”. We then include our text and end with the reset code “\[0m” to return the text to its normal style.
Note: The specific codes for text styling may differ depending on the platform and terminal being used. It’s essential to test your code on different platforms to ensure it works correctly.
Here’s an example of how to create bold text using ANSI escape sequences in Python:
print("\u001b[1mThis text is bold!\u001b[0m")
Running this code will output the text “This text is bold!” in bold style in the terminal.
Using ANSI escape sequences for bold text formatting is a simple and lightweight solution. However, it may not be suitable for complex formatting tasks. In such cases, third-party libraries may offer more advanced options.
Styling Text with Third-Party Libraries
Python offers several third-party libraries that provide advanced styling capabilities for text, including making it bold. These libraries offer a range of features and can be installed easily with pip.
1. termcolor
termcolor is a Python library that provides ANSI colored output and text styling. Its lightweight library is easy to use, making it a popular choice for styling text in Python.
To install, run:
pip install termcolor
Here’s an example of how to use termcolor to print bold text:
from termcolor import colored
print(colored('This text is bold', 'bold'))
2. colorama
colorama is a Python library that allows cross-platform printing of colored text, supporting multiple output formats. It is easy to use and widely adopted, making it a valuable addition to your text styling toolkit.
To install, run:
pip install colorama
To print bold text using colorama, you first need to initialize it:
from colorama import init
init()
After that, you can use the Style class to make text bold:
from colorama import Style
print(Style.BRIGHT + 'This text is bold')
3. rich
rich is a Python library that provides rich text and beautiful formatting for console output. It is a powerful library with many advanced features, including table support and syntax highlighting.
To install, run:
pip install rich
Here’s an example of how to use rich to print bold text:
from rich.console import Console
console = Console()
console.print('[bold]This text is bold[/bold]')
Each of these third-party libraries offers unique features and capabilities for styling text in Python, and choosing the right one depends on your specific needs and preferences.
However, all of them provide a straightforward way to create bold text within your Python code, allowing you to enhance the aesthetics and readability of your output.
Implementing Bold Text Formatting in Python Code
Now that we have explored various techniques for achieving bold text formatting in Python, let’s implement it directly in our Python code.
Using the print() Function
The most common use case for bold text formatting in Python is in the print() function output. To make text bold in the console output, we can use ANSI escape sequences with the print() function as follows:
print("\u001b[1m This text is bold! \u001b[0m")
The escape sequence \u001b[1m
sets the text to be bold, and the sequence \u001b[0m
resets the formatting back to normal. We can replace “This text is bold!” with any text we want to format.
Using the Rich Library
The Rich Library provides a simple and elegant way to format text, including making it bold. To use Rich, we first need to install it by running the following command:
!pip install rich
After installing Rich, we can make text bold by using the following code:
from rich.console import Console
console = Console()
console.print("This text is bold!")
The <b>
tag inside the console.print()
function makes the enclosed text bold.
Using the Termcolor Library
The Termcolor library enables us to add colors and styles to text, including bold text. To install Termcolor, we can run the following command:
!pip install termcolor
To use Termcolor and make text bold, we can use the following code:
from termcolor import colored
print(colored("This text is bold!", 'blue', attrs=['bold']))
The colored()
function takes two arguments: the text we want to format and the color we want to use. We can set the attrs=['bold']
argument to make our text bold.
With these simple and effective methods for implementing bold text formatting in Python, we can now enhance the aesthetics and readability of our code with ease.
Conclusion
In conclusion, mastering bold text formatting in Python is essential for improving code readability and making a stronger impact. We have explored various techniques, including using ANSI escape sequences and third-party libraries, to achieve bold text formatting in Python.
By following the techniques discussed in this article, you can enhance the aesthetics and effectiveness of your code. You can make specific lines or blocks of code stand out and draw attention to critical information. Bold text formatting can also make your code easier to navigate and understand.
So why not start incorporating bold text formatting in your Python projects today? With the right tools and techniques, it’s an easy and effective way to make your code more professional and aesthetically pleasing.
FAQ
Why is bold text formatting important in Python?
Bold text formatting in Python is important because it enhances code readability. It allows you to highlight important parts of your code, making it easier to understand and follow.
How can I create bold Python text without third-party libraries?
One way to create bold text in Python without third-party libraries is by using ANSI escape sequences. These sequences allow you to format text in the terminal, making it bold.
You can find examples and explanations of the syntax and usage of ANSI escape sequences in our section on using ANSI escape sequences for bold text.
Are there any third-party libraries available for text styling in Python?
Yes, Python offers various third-party libraries with advanced text styling capabilities. These libraries allow you to make text bold and perform other styling tasks easily.
In our section on styling text with third-party libraries, we discuss some popular libraries, their features, and how to use them to achieve bold text formatting.
Can I implement bold text formatting directly in my Python code?
Absolutely! Implementing bold text formatting directly in your Python code is one of the techniques we cover in our article. We provide step-by-step instructions on how to make text bold within your Python programs, allowing you to apply this formatting wherever necessary.
How can I start incorporating bold text formatting in my Python projects today?
To start incorporating bold text formatting in your Python projects, we recommend reading through our sections on understanding text formatting in Python, using ANSI escape sequences for bold text, and styling text with third-party libraries.
These sections provide all the information you need to get started and enhance the aesthetics and effectiveness of your code.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.