Welcome to our guide on converting binary data to string using Python. As programmers, we understand the significance of binary data in computing and how essential it is to convert it to string.
This article will provide step-by-step instructions and examples to help you learn how to convert binary to string in Python efficiently. By the end of this guide, you’ll be able to decode binary data and enhance your programming skills in Python.
Binary data is essential in computing, and understanding its conversion process is vital. Therefore, before diving into the conversion process, we will explain the basics of binary and string data in Python. This foundational knowledge will set the stage for the conversion process.
Key Takeaways
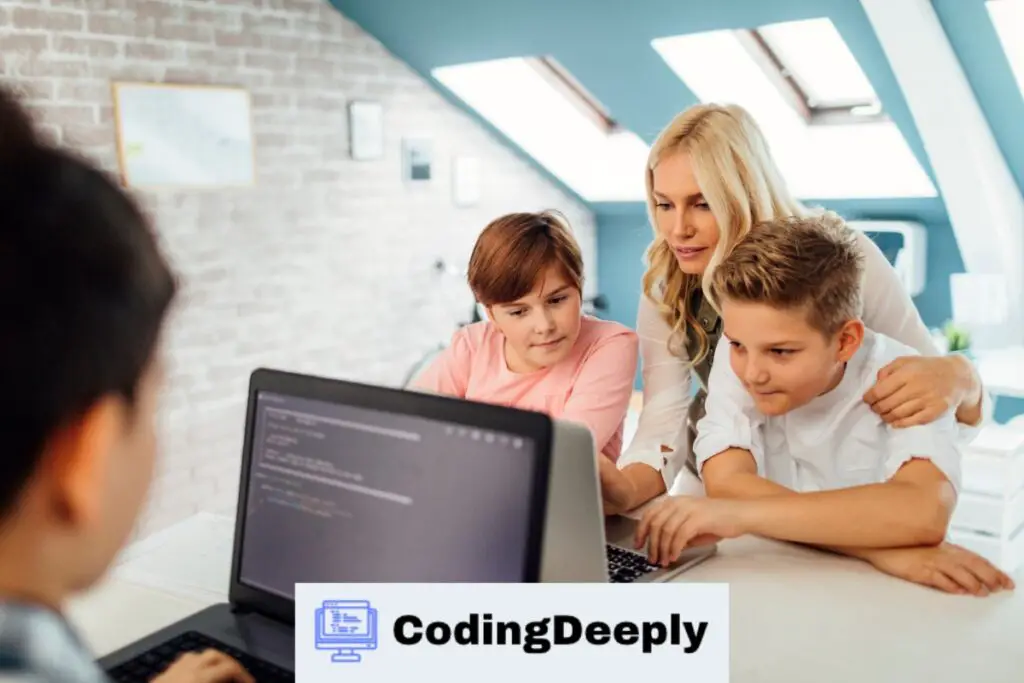
- Binary-to-string conversion is an essential process in programming.
- Python provides built-in libraries to facilitate the conversion process.
- Before starting the conversion process, understanding binary and string data in Python is necessary.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding Binary and String Data in Python
Before we delve into the conversion process, we need to understand the basics of binary and string data in Python. Binary data is represented in a unique system of 1s and 0s, while string data comprises letters, numbers, and symbols.
In Python, binary data is handled using the bytes and bytearray types, which are immutable and mutable. Strings, on the other hand, are represented using the str type.
“Binary data is compact, efficient, and fast to transmit over networks. However, it is not human-readable, which limits its use. Strings, on the other hand, can be easily read and manipulated by humans.”
When dealing with binary data in Python, ensuring that the correct encoding is used when converting to a string is essential, as this can affect the output. Additionally, it’s important to consider factors such as endianness and padding when working with binary data.
Converting Binary to String in Python: Method 1
Now that we understand the basics of binary and string data in Python, let’s dive into the first method of converting binary to string. In this method, we will use the built-in ‘int’ and ‘chr’ functions to convert binary data to decimal and then to ASCII.
The first step is to convert the binary data to decimals using the ‘int’ function. We pass the binary data and base 2 as an argument to this function.
This returns the decimal equivalent of the binary data.
Example:
binary_data = '01101000 01100101 01101100 01101100 01101111' decimal_data = int(binary_data, 2) # returns 17475
Next, we convert the decimal data to ASCII using the ‘chr’ function. We pass the decimal data as an argument to this function, which returns the corresponding ASCII character.
Example:
ascii_char = chr(decimal_data) # returns 'h'
We can repeat the above two steps for each binary data segment and concatenate the resulting ASCII characters to form the final string.
Example:
binary_data = '01101000 01100101 01101100 01101100 01101111' ascii_string = '' for binary_segment in binary_data.split(' '): decimal_data = int(binary_segment, 2) ascii_char = chr(decimal_data) ascii_string += ascii_char print(ascii_string) # returns 'hello'
You can easily convert binary data to string in Python through this method.
Converting Binary to String in Python: Method 2
This section introduces you to a second method of converting binary to string in Python. As with the first method, we will provide code examples and explain the step-by-step process. However, this method has distinct differences that may make it a better fit for certain projects.
Method 2: Using the decode() Function
The second method of converting binary to string in Python involves using the built-in decode() function. This function is specifically designed to convert binary data into a string format.
“The decode() method takes the binary data and the encoding format as parameters and returns the original string.”
You must first have a binary-encoded string to use the decode() function. This can be achieved by using the built-in bytes() function, as demonstrated in the previous section:
binary_data = bytes([72, 101, 108, 108, 111, 32, 87, 111, 114, 108, 100])
Once you have your binary-encoded string, you can use the decode() function to convert it to a normal string:
normal_string = binary_data.decode('utf-8')
In this example, we use the utf-8 encoding format, but you can replace it with any other format you prefer. If you are unsure which encoding format to use, we recommend using utf-8, as it is widely used and versatile.
Like the first method, this method can also be applied to binary data stored in files.
Here’s an example:
with open('binary_data.bin', 'rb') as f:
binary_data = f.read()
normal_string = binary_data.decode('utf-8')
Here, we are opening the file binary_data.bin in binary mode (‘rb’) and using the read() function to read its contents into the binary_data variable.
We then use the decode() function to convert the binary data to a normal string stored in the normal_string variable.
Advantages and Disadvantages of Method 2
One advantage of using the decode() function is that it is specifically designed for binary-to-string conversion, which is likely more efficient than the first method when dealing with large amounts of binary data.
Another advantage is that the decode() function can handle various encoding formats, allowing you to choose the best format for your project.
The main disadvantage of this method is that it requires a binary-encoded string in the first place, which means that you may need to use the bytes() function or read binary data from a file before converting it to a string.
Additionally, if you are working with binary data not encoded using a standard format (such as utf-8), you may need to create your custom decoding function to handle the conversion.
Conclusion
By now, you should have a solid understanding of the two methods for converting binary to string in Python. While both methods have advantages and disadvantages, they are powerful tools for working with binary data in Python.
As you continue to develop your Python skills, you may find that one method works better for your projects than the other, or you need to combine both methods to achieve your goals.
Tips and Best Practices for Binary to String Conversion in Python
Converting binary to string in Python can be challenging, and it’s easy to make mistakes that can cause errors in your code.
Here are some tips and best practices to help you avoid common pitfalls and write efficient code:
1. Understand the Structure of Binary Data
Before converting binary data to string, it’s important to understand the structure of the data. Binary data comprises 1s and 0s, and each bit represents a value in the data. The data size can vary, so it’s important to know the length of the data you are working with.
2. Use Built-in Python Functions
Python provides built-in functions to convert binary data to string. The most common function is the decode() function, which can convert binary data to a string object. This function can be applied to bytes or bytearray objects.
3. Handle Exceptions
When working with binary data, it’s important to handle exceptions properly. An error will be raised if the data is not in a valid format. Therefore, you should always use try/except statements to handle potential errors during conversion.
4. Test Your Code
Testing your code is an essential part of writing reliable code. When converting binary data to string, it’s important to test your code with different types and sizes of data to ensure it works properly in all cases.
5. Use Meaningful Variable Names
Using meaningful variable names can make your code more readable and maintainable. When converting binary data to string, use variable names that accurately describe the processed data.
6. Document Your Code
Documenting your code is important for both yourself and others who may read your code in the future. When converting binary data to string, add comments to your code explaining the conversion process and any potential issues to look out for.
Following these tips and best practices, you can write efficient and reliable code when converting binary data to string in Python.
Conclusion
In conclusion, mastering the process of converting binary to string in Python is essential for any programmer. We hope this guide has helped provide you with a thorough understanding of the conversion process.
You have learned two methods to accomplish this conversion by following the step-by-step instructions and code examples.
Remember to remember the foundational knowledge of binary and string data in Python, which we covered in Section 2. This knowledge will help you decide which conversion method to use and how to handle binary data in your programs.
As you continue to work with binary data in Python, remember to follow the best practices and tips we provided in Section 5. These will help you write more efficient and reliable code and avoid common pitfalls and challenges associated with binary data.
Keep Practicing
With practice and experience, you will become proficient in handling binary data and leveraging it within your Python programs. Continue to explore the vast potential of binary data in Python and enhance your programming skills.
FAQ
What is binary data?
Binary data refers to data represented using the binary numeral system, which consists of only two digits: 0 and 1. It is the fundamental language of computers and is used to store and transmit information within a computer system.
What is string data?
String data refers to a sequence of characters, such as letters, numbers, and symbols. In Python, strings are immutable, meaning they cannot be changed once created. They are commonly used to represent textual data and can be manipulated using string operations.
Why do I need to convert binary to string in Python?
Converting binary data to string in Python is often necessary when working with binary files or network protocols that require data to be in string format. By converting binary data to string, you can perform operations on the data, manipulate it, and display it in a human-readable format.
How can I convert binary to string in Python?
There are multiple methods to convert binary to string in Python. The two methods covered in this guide are Method 1 and Method 2. Each method has advantages and disadvantages, and the choice of method depends on your specific requirements and preferences.
Can I convert string to binary in Python?
Yes, you can convert a string to binary in Python. The process involves encoding the string using a specific encoding scheme, such as UTF-8, which assigns binary values to each character in the string. This encoded binary data can then be manipulated or transmitted.
Are there any tips for binary-to-string conversion in Python?
Yes, we provide tips and best practices in Section 5 to help you with binary-to-string conversion in Python. These tips include handling encoding and decoding errors, choosing the appropriate encoding scheme, and ensuring consistency in your code when working with binary data.
What are the advantages of mastering binary-to-string conversion in Python?
Mastering binary-to-string conversion in Python enhances your programming skills and opens up new possibilities for working with binary data. It allows you to decode and manipulate binary files, work with network protocols, and handle data more efficiently and reliably.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.