As computer programmers, we often encounter binary and hexadecimal values, and it’s crucial to understand how to convert between them. Converting binary to hex in Python can be done using different methods, and we will showcase the most effective ones in this article.
Firstly, we will provide an overview of converting binary to hex in Python, discuss the importance of understanding binary and hexadecimal number systems in programming, and why it’s beneficial to be familiar with these systems.
Next, we will delve into binary and hexadecimal systems, showcase some sample Python code, and provide different methods for converting binary to hex. We will also explore advanced techniques and provide common pitfalls and troubleshooting tips.
By the end of this article, you’ll have the knowledge and resources to confidently convert binary to hex in Python, optimize your code, and avoid common errors. Let’s get started!
Key Takeaways
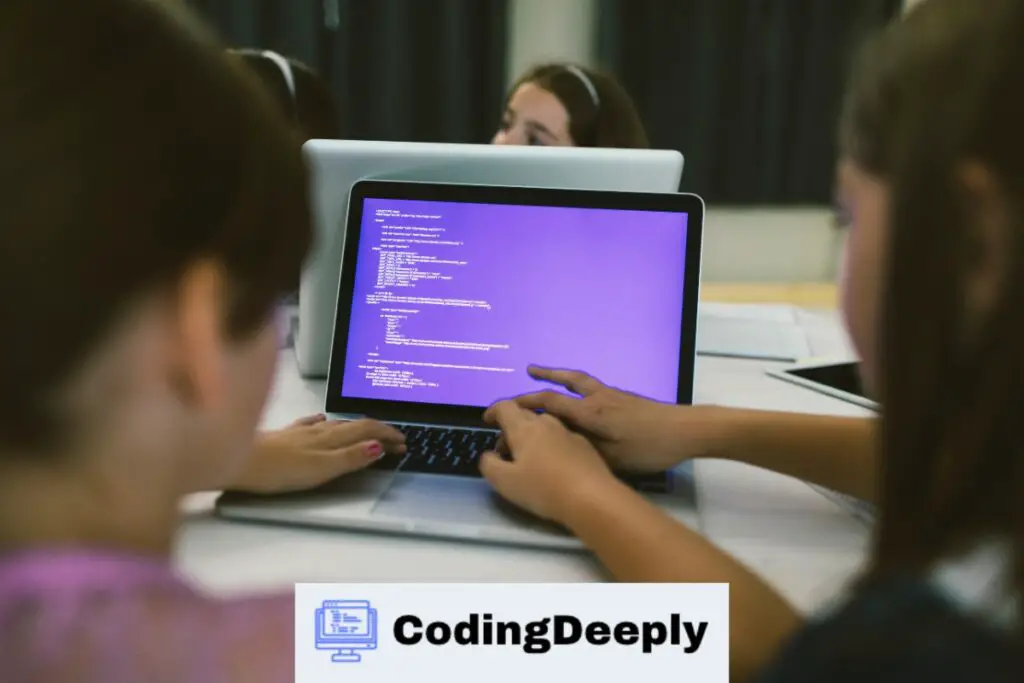
- Converting binary to hex is a crucial skill for programmers
- Understanding binary and hexadecimal number systems is essential for coding
- Python provides different methods for converting binary to hex, with built-in functions and manual conversion algorithms
- Optimizing the conversion process and handling large binary numbers are advanced techniques that can improve code efficiency
- Common pitfalls and troubleshooting tips can help avoid errors and ensure accurate conversions
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding Binary and Hexadecimal Number Systems
Before we dive into converting binary to hex in Python, it’s important to understand the basics of binary and hexadecimal number systems. Both systems are commonly used in computer programming and are vital in processing and storing data.
Binary is a base-2 number system, which only uses two digits – 0 and 1. Each digit in a binary number is referred to as a bit. Binary numbers are commonly used to represent values in digital electronics and computer systems, as they are easy to work with at the hardware level.
On the other hand, hexadecimal is a base-16 number system that uses 16 digits – 0 through 9, and A through F. Hexadecimal numbers are often used in computer programming to represent binary numbers in a more compact and human-readable format.
Each hexadecimal digit represents four binary digits.
“Binary and hexadecimal number systems are both widely used in computer programming and play a vital role in how data is processed and stored.”
To convert between binary and hexadecimal in Python, it’s essential to understand the underlying principles of these number systems.
You’ll be better equipped to work with these values in your Python programs by better understanding binary and hexadecimal.
Python Code for Converting Binary to Hexadecimal
Now that we have a basic understanding of binary and hexadecimal, let’s look at some Python code for converting binary to hexadecimal.
There are several ways to accomplish this conversion in Python, ranging from built-in functions to manual conversion algorithms.
One commonly used approach is the built-in hex() function, which converts a given integer to a hexadecimal string. We can use the int() function with a second argument of 2, specifying the input number’s base to convert a binary number to an integer.
Here’s an example:
bin_num = ‘110101’
hex_num = hex(int(bin_num, 2))
print(hex_num)
# Output: 0x35
In this example, we first define a binary number as a string variable bin_num. We then use the int() function to convert the binary string to an integer, using 2 as the base.
Finally, we pass the resulting integer to the hex() function to obtain the hexadecimal equivalent stored in the hex_num variable.
This is just one example of converting binary to hexadecimal in Python. In the next section, we’ll delve into the details of this conversion process and explore other methods for accomplishing this task.
Converting Binary to Hex in Python
Converting binary to hexadecimal is a common task in computer programming, and Python provides various methods for accomplishing this task.
One popular method is using the built-in Python function hex(), which takes an integer argument and returns a corresponding hexadecimal string:
# Using hex() to convert binary to hexadecimal
binary_num = 0b10100110
hex_num = hex(binary_num)print(hex_num) # Output: 0xa6
In this example, we first define a binary number 0b10100110
. We then use the hex() function to convert this binary number to hexadecimal, which is stored in the variable hex_num
. Finally, we print the hexadecimal string 0xa6
.
If you want to convert a binary string to a hexadecimal string, you can use the int() function to convert the binary string to an integer and then use the hex() function to convert the integer to a hexadecimal string:
# Using int() and hex() to convert binary string to hexadecimal string
binary_str = "10100110"
binary_num = int(binary_str, 2)
hex_str = hex(binary_num)print(hex_str) # Output: 0xa6
In this example, we first define a binary string "10100110"
. We then use the int() function to convert this binary string to an integer, specifying the base as 2.
Next, we use the hex() function to convert this integer to a hexadecimal string, which is stored in the variable hex_str
. Finally, we print the hexadecimal string 0xa6
.
Alternatively, you can convert binary to hexadecimal manually, using an algorithm that takes each 4-bit chunk of the binary number and maps it to its corresponding hexadecimal digit:
- Start from the rightmost digit of the binary number.
- Group the bits into chunks of 4 starting from the rightmost digit. If there are not enough bits on the left side to form a chunk of 4, add 0s to the left until the leftmost bit forms a chunk of 4.
- Map each chunk of 4 to its corresponding hexadecimal digit:
0000
maps to0
0001
maps to1
0010
maps to2
0011
maps to3
0100
maps to4
0101
maps to5
0110
maps to6
0111
maps to7
1000
maps to8
1001
maps to9
1010
maps toA
1011
maps toB
1100
maps toC
1101
maps toD
1110
maps toE
1111
maps toF
- Combine the hexadecimal digits from left to right to form the final hexadecimal number.
Here is an example Python function that converts binary to hexadecimal using this algorithm:
# Converting binary to hexadecimal using manual algorithm
def binary_to_hex(binary_str):
hex_digits = "0123456789ABCDEF" # mapping table
hex_str = ""
i = len(binary_str) - 1while i >= 0:
# Grouping the bits into 4-bit chunks
chunk = binary_str[max(0, i-3):i+1]
# Converting the chunk to its hexadecimal digit
hex_digit = hex_digits[int(chunk, 2)]
hex_str = hex_digit + hex_str
i -= 4return hex_str
In this example, we define a Python function binary_to_hex()
that takes a binary string as input and returns its hexadecimal equivalent.
The function uses a while loop to iterate through each 4-bit chunk of the binary string, convert it to its corresponding hexadecimal digit using a mapping table, and append it to the left side of the output hexadecimal string.
Finally, the function returns the output hexadecimal string.
Converting Binary to Hex: Step-by-Step Example
Let’s walk through an example of converting a binary number to hexadecimal in Python. We will use the following binary input: 101101.
- Divide the binary number into groups of four: Since each hexadecimal digit represents four binary digits, we can split the binary number into groups of four starting from the right. In this case, we can add a leading zero to the left to make it a multiple of four: 0101 1010.
- Convert each group of four to its hexadecimal equivalent: Now, we can convert each group of four binary digits into its equivalent. You can use a conversion table to do this, but converting them manually is possible. For example, 0101 is equivalent to the hexadecimal digit 5, and 1010 is equivalent to A. Thus, our binary number 101101 is equivalent to the hexadecimal number 5A.
- Convert the hexadecimal result to a string: In Python, we can use the built-in hex() function to convert our hexadecimal number to a string. We can also add a leading 0x to denote that it’s in hexadecimal format. The final result is
'0x5a'
.
It’s important to note that there are different approaches to converting binary to hexadecimal, and some may be more efficient than others, depending on the specific use case. However, this step-by-step example should give you a solid understanding of the general process.
Advanced Techniques for Binary to Hex Conversion
Now that we have covered the basics of binary-to-hex conversion in Python let’s explore some advanced techniques to optimize our code and handle larger binary numbers easily.
1. Using the Bitwise Operator
A powerful technique for binary-to-hex conversion is using the bitwise operator. This operator allows us to individually manipulate each bit of the binary number, resulting in faster and more efficient code. Here is an example:
binary_num = “101101”
hex_num = hex(int(binary_num, 2))
Using the built-in int function, we can convert the binary number to an integer, and then use the hex function to obtain the hexadecimal equivalent.
2. Handling Large Binary Numbers
We may encounter errors or overflow issues during conversion when working with very large binary numbers. We can split the binary number into smaller chunks and convert them individually to handle these scenarios. We can then combine the results to obtain the final hexadecimal value.
Here is an example:
binary_num = “10101100100111010101101110011010111”
chunks = [binary_num[i:i+8] for i in range(0, len(binary_num), 8)]
hex_num = “”
for chunk in chunks:
hex_num += hex(int(chunk, 2))[2:]
In this code, we divide the binary number into chunks of 8 bits each and convert each chunk to hex individually. We then concatenate the resulting hexadecimal values to obtain the final output.
3. Improving Code Efficiency
Another way to optimize our binary-to-hex conversion code is to eliminate unnecessary steps and reduce computation time.
One technique is to use lookup tables to store frequently used values and reduce the number of calculations needed. Another technique is to use the Python bytearray function, which allows us to convert the binary number to a hexadecimal string directly.
Here is an example:
binary_num = “1100111011011011”
lookup_table = {“0000”: “0”, “0001”: “1”, “0010”: “2”, “0011”: “3”, “0100”: “4”, “0101”: “5”, “0110”: “6”, “0111”: “7”, “1000”: “8”, “1001”: “9”, “1010”: “a”, “1011”: “b”, “1100”: “c”, “1101”: “d”, “1110”: “e”, “1111”: “f”}
hex_num = “”
for i in range(0, len(binary_num), 4):
chunk = binary_num[i:i + 4]
hex_num += lookup_table[chunk]
Using the lookup table, we can directly convert each chunk of 4 bits to its corresponding hexadecimal value without any calculations. This significantly reduces computation time and improves our code efficiency.
By utilizing these advanced techniques, we can convert binary to hex in Python more efficiently and accurately, even when dealing with large or complex binary numbers.
Common Pitfalls and Troubleshooting Tips
Converting binary to hex in Python can be challenging, with various potential pitfalls and errors to watch out for. Here are some common issues you may encounter when converting binary to hex and how to troubleshoot them:
1. Input errors
One of the most common mistakes when converting binary to hex is inputting the wrong value. Double-check that the binary number inputted is correct and contains only 0s and 1s. If the input is not a binary number, a ValueError will be raised.
2. Trailing zeroes
Be wary of trailing zeroes in your binary input, as they can affect the resulting hex value. One approach to avoid this issue is to use string slicing to remove the trailing zeroes before the conversion.
3. Endianness
Endianness refers to the order bytes are arranged in a binary number. In some cases, you may need to convert the endianness of your binary input before converting to hex. This can be done using the struct module in Python.
4. Incorrect output format
When converting binary to hex in Python, you can output the result as a string or integer. Depending on your use case, you may need to convert the output format after the initial conversion.
You can use the int() function with a base of 16 to convert the hex string to an int.
5. Optimization
The conversion process can be slow and inefficient if you’re working with large binary numbers. One optimization technique uses bitwise operations instead of converting the entire binary number to decimal.
This can significantly improve the performance of your code.
By considering these common pitfalls and implementing the appropriate troubleshooting tips, you can ensure accurate and reliable binary-to-hex conversions in your Python programs.
Conclusion
Converting binary to hex in Python is an essential skill for any programmer. Understanding the binary and hexadecimal number systems is crucial to working with binary and hex values in your Python programs.
We hope this step-by-step guide has given you the knowledge and resources to accomplish this task effortlessly.
Remember that there are different techniques for converting binary to hex in Python, each with strengths and weaknesses. By exploring multiple methods, you can optimize the conversion process, handle large binary numbers, and improve code efficiency.
Stay Aware of Common Pitfalls
While converting binary to hex in Python is useful, there are common pitfalls and challenges. If you encounter an unexpected result or error, troubleshoot and analyze the issue using the tips and techniques discussed in this article.
By understanding and addressing these challenges, you can ensure accurate and reliable binary-to-hex conversions.
Thank you for reading, and we wish you success in your programming endeavors!
FAQ
Why is converting binary to hex in Python useful?
Converting binary to hex in Python is useful in computer programming because hexadecimal numbers are often used to represent binary data in a more compact and readable format.
Hexadecimal numbers are also commonly used in various applications, such as network protocols and memory addresses.
How can I convert binary to hex in Python?
There are multiple methods to convert binary to hex in Python. You can use built-in functions like `hex()` or manually convert each binary digit to its hexadecimal equivalent using an algorithm.
Both approaches will be explained in detail in the respective sections of this guide.
Are there any built-in functions for converting binary to hex in Python?
Yes, Python provides a built-in function called `hex()` that can be used to directly convert a decimal or binary number to its hexadecimal representation.
This function simplifies the conversion process and is recommended for its convenience.
Can I convert a binary string to hex in Python?
Yes, you can convert a binary string to its hexadecimal equivalent in Python. You can input a binary string and obtain the corresponding hex value using appropriate conversion techniques.
Sample code and step-by-step explanations are provided in the relevant sections of this guide.
What are some common pitfalls when converting binary to hex in Python?
Some common pitfalls when converting binary to hex in Python include incorrect input formatting, mishandling leading zeros, or misunderstanding the conversion algorithm.
These issues can lead to incorrect results or unexpected errors. The troubleshooting section of this guide provides tips and solutions to overcome these challenges.
How can I optimize the binary-to-hex conversion process in Python?
The binary-to-hex conversion process can be optimized in Python using more efficient algorithms or techniques. For example, bitwise operations can perform the conversion more quickly. The advanced techniques section of this guide explores various optimization strategies in detail.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.