Beautifulsoup is a popular Python library for parsing HTML and XML documents. Its flexibility in handling complex HTML structures and extracting data from web pages makes it an invaluable tool for web scraping tasks.
In this comprehensive guide, we will explore the basics of Beautifulsoup and delve into advanced techniques and features.
Key Takeaways:
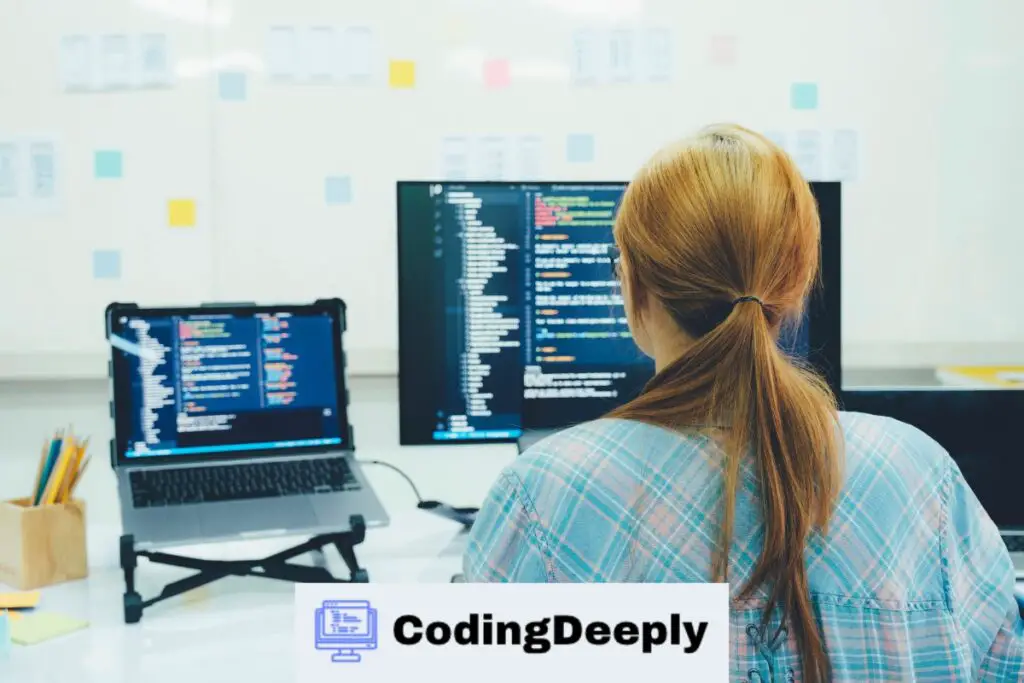
- Beautifulsoup is a powerful Python library for web scraping tasks.
- It simplifies data extraction by providing flexible methods to navigate through HTML documents.
- Our guide covers the basics of Beautifulsoup, installation, data extraction, documentation, advanced techniques, and best practices for web scraping.
- Mastering Beautifulsoup can enhance your data extraction capabilities and streamline your web scraping workflow.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Introduction to Beautifulsoup for Python 3.6
This section will provide a comprehensive overview of Beautifulsoup and its capabilities. Beautifulsoup is a Python library for parsing HTML and XML documents and is commonly used for web scraping tasks.
With Beautifulsoup, we can easily and flexibly extract data from web pages.
Beautifulsoup offers many advantages over other web scraping tools, including:
- Easy to learn and use
- Flexible parsing options
- Robust error handling
- Compatibility with a wide range of operating systems and Python versions, including Python 3.6
Using Beautifulsoup, we can extract data from web pages in various ways. We can search for specific HTML elements, navigate the document tree, and access attributes and contents.
Beautifulsoup’s versatility and power make it an essential tool for any web scraper.
Installing Beautifulsoup 4 for Python 3.6
We need to install it on our system before we can start using Beautifulsoup for web scraping tasks.
Installing Beautifulsoup for Python 3.6 is a straightforward process, and we’ll guide you through it step-by-step.
Step 1: Check Your Python Version
Firstly, you must ensure that your system has Python 3.6 or higher installed. To check your Python version, open your terminal or command prompt and enter the following command:
python –version
You’re good to go if you see a version number greater than 3.6! If not, you must download and install Python 3.6 or higher from the official Python website.
Step 2: Install Beautifulsoup 4
Once you install Python 3.6 or higher, you can install Beautifulsoup 4. Here’s how:
- Open your terminal or command prompt.
- Enter the following command to install Beautifulsoup 4 using pip:
pip install beautifulsoup4
- Once the installation is complete, you can test whether Beautifulsoup is installed correctly by running the following command:
python
from bs4 import BeautifulSoup
If you don’t encounter any errors, Beautifulsoup 4 is now installed and ready to use!
Extracting Data using Beautifulsoup
Now that we have Beautifulsoup installed and ready to use, let’s dive into the core functionality of this powerful library: extracting data from HTML documents.
One of the key advantages of using Beautifulsoup is its ability to search for specific HTML elements and navigate the document tree to access their contents and attributes.
Searching for HTML Elements
The find() method searches for the first occurrence of a specific HTML element. For example, to extract the title of a web page, we can use the following code:
title_tag = soup.find(‘title’)
title_text = title_tag.text
The text attribute retrieves the text content of the HTML element.
The find_all() method searches for all occurrences of a specific HTML element. We can use this method to extract multiple elements, such as all the links on a web page:
links = soup.find_all(‘a’)
for link in links:
print(link.get(‘href’))
The get() method retrieves the value of a given attribute of an HTML element.
Navigating the Document Tree
HTML documents are organized as a tree structure, with each element as a node in the tree. Beautifulsoup provides various methods to navigate this tree and access its elements and attributes.
The parent attribute retrieves the parent node of an HTML element:
parent_tag = title_tag.parent
The next_sibling attribute retrieves the next sibling node of an HTML element:
next_sibling_tag = title_tag.next_sibling
The previous_sibling attribute retrieves the previous sibling node of an HTML element:
prev_sibling_tag = title_tag.previous_sibling
Accessing Attributes and Contents
Beautifulsoup provides various attributes and methods to access the contents and attributes of an HTML element.
The string attribute retrieves the string content of an HTML element:
text = title_tag.string
The attrs attribute retrieves a dictionary of the attributes of an HTML element:
attrs_dict = title_tag.attrs
With these methods and techniques, we can effectively extract data from HTML documents using Beautifulsoup.
Beautifulsoup Python Documentation
As with any tool, it is essential to understand its documentation well to make the most out of it. Beautifulsoup has excellent documentation that covers all aspects of the library and provides numerous examples to assist you in your projects.
Firstly, the official Beautifulsoup documentation provides a comprehensive overview of the library and its features. It explains the structure of HTML and XML documents and how Beautifulsoup can navigate, search, and parse them. You can also find explanations of each method and attribute of Beautifulsoup, as well as examples of their application.
Next, the Python documentation offers detailed information on all Python libraries, including Beautifulsoup. It also provides useful resources on Python syntax and programming tips that can aid in utilizing Beautifulsoup effectively.
The Beautifulsoup documentation and Python documentation are valuable resources that will serve as useful references throughout your web scraping journey.
Finally, besides the official documentation, the Python community has developed various tutorials, blog posts, and online courses covering Beautifulsoup and its applications extensively.
- Real Python provides a comprehensive tutorial on using Beautifulsoup for web scraping. It is an excellent resource for beginners and advanced Python developers alike.
- Dataquest offers a practical web scraping course, including Beautifulsoup and other essential tools for data extraction.
- ScrapingBee offers a blog post that provides expert advice on web scraping best practices and tips for avoiding anti-scraping measures.
By leveraging these resources, you can enhance your knowledge of Beautifulsoup and improve your web scraping capabilities.
Advanced Techniques with Beautifulsoup
Now that you have a solid foundation in the basics of Beautifulsoup, it’s time to explore more advanced techniques and features. Here are a few examples of how you can leverage the full power of Beautifulsoup for complex web scraping scenarios:
1. Handling Nested Elements
When dealing with complex HTML documents, you’ll often encounter nested elements that can make data extraction tricky. Beautifulsoup provides several methods to navigate through nested elements and extract the relevant data. One useful technique is to use the descendants method, which searches through all the child and grandchild elements of a parent element:
parent = soup.find(‘div’, {‘class’: ‘parent’})
for child in parent.descendants:
if child.name == ‘span’:
print(child.text)
2. Scraping Tables
Web pages often include tables that contain valuable data, such as pricing information, product specifications, or stock prices. Beautifulsoup has built-in support for parsing and extracting data from HTML tables. You can use the find_all method to locate the table element and then navigate through the rows and cells of the table:
table = soup.find(‘table’)
rows = table.find_all(‘tr’)
for row in rows:
cells = row.find_all(‘td’)
for cell in cells:
print(cell.text)
3. Scraping Dynamic Data
Some web pages use dynamic content that is loaded or updated using JavaScript. This can make it difficult to extract all the relevant data in one go, as the content may not be present in the initial HTML source code.
Beautifulsoup can help you retrieve dynamic data by simulating a web browser and loading the page with all its content:
from bs4 import BeautifulSoup
from selenium import webdriverdriver = webdriver.Chrome()
driver.get(‘https://www.example.com’)
html = driver.page_source
soup = BeautifulSoup(html, ‘html.parser’)
Using Selenium in conjunction with Beautifulsoup, you can extract all the data from even the most complex and dynamic web pages.
Best Practices for Web Scraping with Beautifulsoup
Web scraping can present challenges, from handling dynamic content to avoiding anti-scraping measures. However, with the right approach and techniques, you can optimize your workflow and ensure the integrity of your data. Here are some best practices for web scraping with Beautifulsoup:
1. Understand the website structure
Before diving into web scraping, it’s important to familiarize yourself with the website’s structure. This includes identifying the HTML elements containing the needed data and understanding the website’s organization.
Understanding the website’s structure allows you to write more efficient scraping scripts and avoid unnecessary requests.
2. Handle dynamic content
Many modern websites rely on dynamic content, which changes based on user input or other factors. This can present challenges for web scraping, as the data you need may not be present in the initial HTML response.
To handle dynamic content, you can use a combination of tools, including Beautifulsoup, Selenium, and API requests.
3. Deal with anti-scraping measures
Some websites implement anti-scraping measures to prevent automated data extraction. These measures can include CAPTCHAs, IP blocking, and rate limiting.
To avoid these measures, you can use rotating proxies, adding delays between requests, and using user-agent headers to mimic human behavior.
4. Ensure data integrity
When scraping data from websites, it’s important to ensure the integrity of your data. This includes checking that the data is accurate, consistent, and complete.
To do this, you can use data validation, cleaning, and normalization techniques.
5. Write efficient and maintainable scraping scripts
Writing efficient and maintainable scraping scripts is essential for long-term success in web scraping. This includes using modular code, commenting your code, and following best practices for coding style and structure.
Writing efficient and maintainable code saves time and avoids errors in your web scraping projects.
By following these best practices, you can optimize your web scraping workflow and avoid common pitfalls. With Beautifulsoup and other tools, you can extract valuable data from the web and enhance your data extraction capabilities.
Conclusion
As we wrap up our exploration of Beautifulsoup for Python 3.6, we can confidently say that mastering this library is a significant step towards simplifying web scraping tasks.
By utilizing Beautifulsoup’s powerful capabilities, you can extract data from web pages efficiently and effectively.
We have covered the basics of Beautifulsoup, including installation and data extraction techniques, and explored its advanced features. Additionally, we have emphasized the importance of following best practices when web scraping with Beautifulsoup to optimize your workflow and avoid common pitfalls.
By applying the knowledge gained from this article, you will be able to leverage the full potential of Beautifulsoup and unlock new possibilities for your web scraping projects. We encourage you to continue exploring the rich documentation and examples provided by Beautifulsoup’s community and experiment with practical use cases.
FAQ
What is Beautifulsoup?
Beautifulsoup is a Python library that simplifies web scraping tasks by providing an easy-to-use interface for parsing HTML and XML documents.
How can Beautifulsoup enhance my project’s efficiency?
Beautifulsoup allows you to easily extract data from web pages, saving you time and effort compared to manual extraction methods. It provides methods for searching specific HTML elements, navigating the document tree, and accessing attributes and contents.
How do I install Beautifulsoup 4 for Python 3.6?
To install Beautifulsoup 4 for Python 3.6, you can use pip, the Python package installer. Simply run the command “pip install beautifulsoup4” in your terminal or command prompt.
Where can I find the official documentation for Beautifulsoup?
The official documentation for Beautifulsoup can be found on the Beautifulsoup website. It provides comprehensive information about the library’s features, methods, and examples.
What are some advanced techniques I can use with Beautifulsoup?
With Beautifulsoup, you can employ advanced techniques such as handling dynamic content, dealing with anti-scraping measures, and tackling complex web scraping scenarios. Practical examples and use cases will be showcased to demonstrate the power and flexibility of Beautifulsoup.
What are some best practices for web scraping with Beautifulsoup?
When web scraping with Beautifulsoup, handling dynamic content, dealing with anti-scraping measures, ensuring data integrity, and writing efficient and maintainable scraping scripts is recommended. These best practices will help optimize your web scraping workflow and avoid common pitfalls.
What are the key takeaways from exploring Beautifulsoup for Python 3.6?
Mastering Beautifulsoup can simplify web scraping tasks and enhance your data extraction capabilities. Beautifulsoup is an effective tool for web scraping, making extracting data from web pages easier and more efficient.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.