Welcome to our article on list sorting in Python.
This section will tackle whether or not lists are ordered in Python. We will explore the implications of list ordering and its importance in programming. Additionally, we will provide an overview of list-sorting techniques that can be used to order Python lists.
Let’s go!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
How to Check if Lists are Ordered in Python
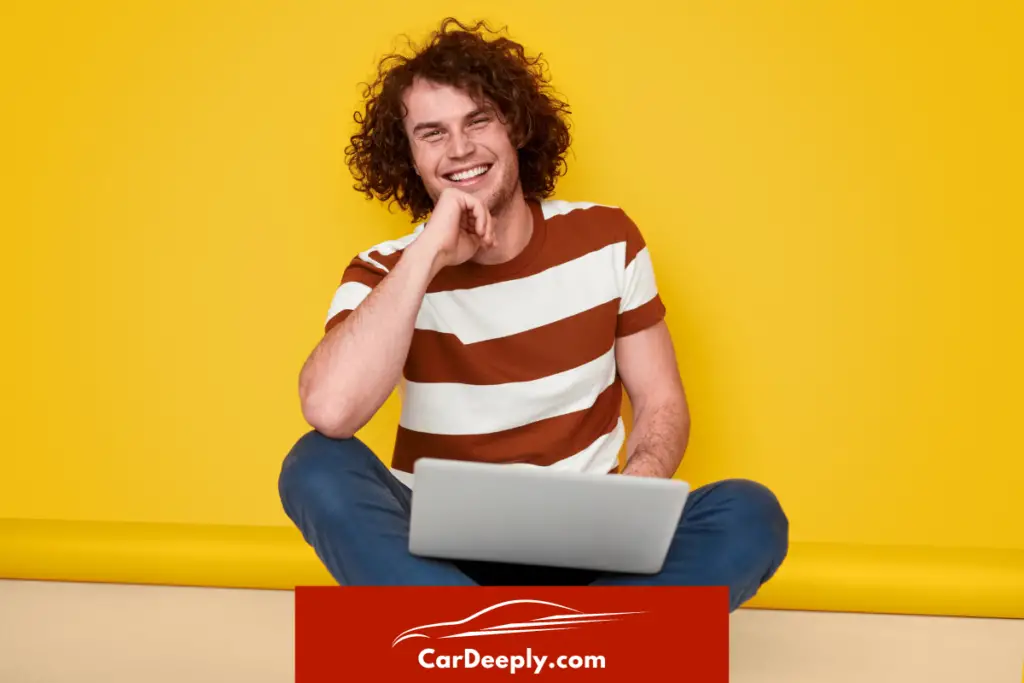
Before we dive into ways to order lists in Python, let’s first discuss how to check if a list is already ordered.
There are a few different methods for doing this.
Method 1: Comparing with a Sorted Copy
One way to check if a list is ordered is to compare it to a sorted copy of itself. We can use the sorted() function to create a sorted copy of the list and then compare the two lists using the == operator.
Code Example: |
---|
|
In this example, we first define the list ‘my_list’. We then use an if/else statement to check whether or not the list is ordered.
The sorted() function creates a sorted copy of ‘my_list’, which we then compare to the original list using the == operator. If they are equal, we print “List is ordered!”. If not, we print “List is not ordered.”
Method 2: Checking with a For Loop
Another method for checking the order of a list is to use a for loop to compare each element to the next element in the list.
The list is ordered if each element is greater than or equal to the previous element. The list is not ordered if any element is less than the previous element.
Code Example: |
---|
|
In this example, we first define the list ‘my_list’ and set the variable ‘ordered’ to ‘True’. We then use a for loop to iterate through the list, comparing each element to the next.
If any element is less than the previous element, we set ‘ordered’ to ‘False’ and break out of the loop. Finally, we check the value of ‘ordered’. If it is still ‘True’, we print “List is ordered!”. If ‘ordered’ is ‘False’, we print “List is not ordered.”
Both of these methods are effective for checking if a list is ordered in Python. Choose the method that works best for your needs!
Ordering Lists in Python
Python provides several built-in functions and custom sorting techniques that can be used to order lists.
In this section, we will explore the various techniques available for sorting lists in Python and provide examples to help you better understand how to implement them.
Built-In List Sorting Functions
Python provides two built-in sorting functions that can be used to order lists:
Function | Description |
---|---|
sort() | Sorts the elements of a list in ascending order |
sorted() | Returns a new list sorted in ascending order |
The sort() function modifies the original list, while the sorted() function returns a new sorted list, leaving the original list unchanged.
To use these functions, call them and pass the list you want to sort as an argument:
my_list = [4, 1, 3, 2] my_list.sort() # modifies original list print(my_list) # Output: [1, 2, 3, 4] my_list = [4, 1, 3, 2] new_list = sorted(my_list) # returns new sorted list print(my_list) # Output: [4, 1, 3, 2] print(new_list) # Output: [1, 2, 3, 4]
Custom Sorting Techniques
Python also allows you to define custom sorting functions that can be used to order lists based on specific criteria. To define a custom sorting function, you need to define a function that takes a single argument and returns a value for sorting.
This value could be a string, a number, or a tuple.
Once you have defined your custom sorting function, you can use it with the sort() or sorted() function by passing it as the key argument:
def sort_by_length(item): return len(item) my_list = ['apple', 'banana', 'cherry', 'date'] my_list.sort(key=sort_by_length) # sorts by length print(my_list) # Output: ['date', 'apple', 'banana', 'cherry']
Advanced List Sorting Techniques
Python provides several advanced list-sorting algorithms that can be used to order lists more efficiently. These algorithms include:
- Heap sort
- Quick sort
- Merge sort
- Timsort
The sorted() function uses Timsort by default, a combination of merge sort and insertion sort. However, you can also specify which algorithm to use by passing the algorithm argument:
my_list = [4, 1, 3, 2] new_list = sorted(my_list, algorithm='quicksort') # uses quick sort algorithm print(new_list) # Output: [1, 2, 3, 4]
Advanced sorting algorithms can be useful when dealing with large lists or when performance is a concern.
Python List Order Validation
Validating the order of a list in Python can be accomplished by checking whether the list is sorted in ascending or descending order.
If the list is not sorted, we can sort it using various techniques and then compare it to the original list to determine if it meets our desired order.
Here are some methods for validating the order of lists in Python:
Method | Description |
---|---|
sorted() | Returns a sorted copy of the original list |
all() | Returns True if all elements in the list are in the desired order |
any() | Returns True if any element in the list is in the desired order |
sorted() == original | Returns True if the sorted list is equal to the original list |
Let’s take a look at some examples of how to use these methods:
# Example list my_list = [3, 5, 1, 7, 2] # Check if the list is sorted in ascending order if my_list == sorted(my_list): print("The list is sorted in ascending order.") else: print("The list is not sorted in ascending order.") # Check if the list is sorted in descending order if my_list == sorted(my_list, reverse=True): print("The list is sorted in descending order.") else: print("The list is not sorted in descending order.") # Check if all elements are in ascending order if all(my_list[i] <= my_list[i + 1] for i in range(len(my_list) - 1)): print("All elements are in ascending order.") else: print("Not all elements are in ascending order.") # Check if any elements are in ascending order if any(my_list[i] <= my_list[i + 1] for i in range(len(my_list) - 1)): print("Some elements are in ascending order.") else: print("No elements are in ascending order.")
The above code will output:
The list is not sorted in ascending order. The list is not sorted in descending order. Not all elements are in ascending order. Some elements are in ascending order.
Using these methods, we can easily validate the order of a list in Python.
Understanding the Concept of List Ordering in Python
When working with lists in Python, the concept of order is important to understand. In simple terms, ordering refers to arranging items in a list.
Lists in Python are ordered, meaning that the items in a list have a specific order. This order is defined by the position of each item in the list, also known as its index. The first item in a list has an index of 0; the second item has an index of 1, and so on.
Understanding list ordering is crucial because it allows you to manipulate and work with lists in various ways. For example, you may want to sort a list in ascending or descending order using one of the many list-sorting techniques available in Python.
Additionally, understanding list ordering can help you determine if a list contains a specific item or has been modified somehow. For instance, if you know the order of items in a list, you can quickly determine if an item is missing or if there are duplicates.
Different Types of List Sorting Techniques in Python
Sorting lists is one of the most common tasks in programming. Python provides several built-in functions to sort lists, and many custom sorting techniques can be used to achieve more specific sorting objectives.
Three general types of sorting techniques can be used in Python:
- Built-in sorting functions: Python provides several built-in functions, including sort() and sorted() that can be used to sort lists in ascending or descending order.
- Custom sorting functions: Developers can create custom sorting functions that provide more flexibility and specificity than built-in functions.
- Advanced sorting algorithms: For particularly large or complex lists, advanced sorting algorithms such as quicksort, merge sort, and heapsort may be more appropriate.
Built-in Sorting Functions in Python
Python provides two built-in functions for sorting lists: sort() and sorted().
Function | Description |
---|---|
sort() | Sorts the list in place in ascending order. Modifies the original list. |
sorted() | Returns a sorted copy of the list in ascending order. Does not modify the original list. |
The sort() function modifies the original list, so making a copy of the original list is important if you need to preserve the original order.
Here is an example of how to use the sort() function:
my_list = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5] my_list.sort() print(my_list) # Output: [1, 1, 2, 3, 3, 4, 5, 5, 5, 6, 9]
The sorted() function returns a new sorted list and does not modify the original list. Here is an example of how to use the sorted() function:
my_list = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5] sorted_list = sorted(my_list) print(sorted_list) # Output: [1, 1, 2, 3, 3, 4, 5, 5, 5, 6, 9] print(my_list) # Output: [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
Custom Sorting Techniques in Python
Developers can create custom sorting functions using the Python append() function, which adds an element to the end of a list, and the Python lambda function, which allows you to create small, anonymous functions.
Here is an example of how to use the append() and lambda functions to create a custom sorting function that sorts a list of words by their length:
words = ["apple", "banana", "cherry", "date", "elderberry", "fig", "grape"] words.sort(key=lambda word: len(word)) print(words) # Output: ['fig', 'date', 'apple', 'grape', 'banana', 'cherry', 'elderberry']
In this example, the lambda function takes a word as an argument and returns the length of the word. The sort() function then uses this length to sort the list in ascending order.
Advanced List Sorting Techniques in Python
Advanced sorting algorithms such as quicksort, merge sort, and heapsort may be more appropriate for particularly large or complex lists.
These algorithms are beyond this article’s scope, but it is important to know if you plan to work with large datasets or perform complex sorting tasks.
Python provides various libraries for performing advanced sorting, such as NumPy and SciPy, ideal for scientific computing, and Pandas, useful for handling and manipulating large datasets.
Built-In Sorting Functions in Python
Python has several built-in functions that can be used to sort a list.
The most commonly used function is the sort() function, which sorts the list in ascending order by default. Let’s take a closer look at how to use this function.
Using the sort() Function
To use the sort() function, call it on the list you want to sort:
my_list = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5] my_list.sort() print(my_list)
This will output:
[1, 1, 2, 3, 3, 4, 5, 5, 5, 6, 9]
The sort() function can also be used to sort a list in descending order by passing the reverse=True argument:
my_list.sort(reverse=True) print(my_list)
This will output:
[9, 6, 5, 5, 5, 4, 3, 3, 2, 1, 1]
Sorting Lists of Strings
The sort() function can also be used to sort lists of strings:
my_list = ['apple', 'banana', 'cherry', 'date'] my_list.sort() print(my_list)
This will output:
['apple', 'banana', 'cherry', 'date']
Note that strings are sorted in alphabetical order by default.
Sorting Lists with Key Functions
The sort() function also has an optional key parameter that allows you to specify a function to determine the sort order.
For example, to sort a list of strings based on the length of each string, you could use the len() function as the key:
my_list = ['apple', 'banana', 'cherry', 'date'] my_list.sort(key=len) print(my_list)
This will output:
['date', 'apple', 'banana', 'cherry']
Here, the key=len argument tells Python to sort the list based on the length of each string.
Another example of using the key parameter is sorting a list of tuples based on the second element of each tuple:
my_list = [(1, 'apple'), (3, 'banana'), (2, 'cherry'), (4, 'date')] my_list.sort(key=lambda x: x[1]) print(my_list)
This will output:
[(1, 'apple'), (3, 'banana'), (2, 'cherry'), (4, 'date')]
Here, the key=lambda x: x[1] argument tells Python to sort the list based on the second element of each tuple.
Custom Sorting Techniques in Python
In addition to the built-in sorting functions available in Python, custom sorting techniques can be used to order lists to fit specific needs.
These techniques are especially useful when a standard sorting function doesn’t meet specific requirements.
Custom Sorting Function
A custom sorting function takes two arguments and returns -1, 0, or 1 depending on whether the first argument should be sorted before, at the same level, or after the second argument.
Here is an example of a custom sorting function that sorts based on the length of the strings:
Code | Output |
---|---|
| [‘apple’, ‘orange’, ‘banana’, ‘pineapple’] |
In this example, the sort_by_length function returns the length of each string element in the list “fruits”. The fruits list is then sorted based on the values returned by the sort_by_length function.
Custom Reverse Sorting
In addition to custom sorting functions, sorting a list in reverse order is possible using the reverse parameter. To use reverse sorting, set reverse=True.
Here is an example of reverse sorting using a custom sorting function:
Code | Output |
---|---|
| [9, 6, 5, 5, 5, 4, 3, 3, 2, 1, 1] |
In this example, the reverse_sort function returns the negative value of each element in the list “numbers”. The numbers list is then sorted based on the negative values returned by the reverse_sort function.
Mixed Data Type Sorting
Another use case for custom sorting is when sorting lists containing mixed data types.
Here is an example of custom sorting for a list with mixed data types:
Code | Output |
---|---|
| [‘banana’, ‘apple’, ‘orange’, 2, 4, 1] |
In this example, the custom_sort function sorts the list “mixed_list” by first grouping all string elements (0), followed by the integer elements (1).
Advanced List Sorting Techniques in Python
While Python provides several built-in functions for sorting lists, advanced techniques can also be used to achieve more specific sorting requirements.
Heap Sort
Heap sort is a sorting algorithm that can be used to sort lists efficiently. It works by creating a binary tree structure from the list and then repeatedly taking the smallest element and swapping it with the last element of the list.
Here’s an example of how to use heap sort in Python:
<!-- Python code --> import heapq def heap_sort(lst): heapq.heapify(lst) return [heapq.heappop(lst) for i in range(len(lst))] lst = [5, 3, 8, 2, 0, 11, 23, 1] print(heap_sort(lst)) # Output: [0, 1, 2, 3, 5, 8, 11, 23]
Merge Sort
Merge sort is another popular sorting algorithm that is used in Python. It works by dividing the list into smaller and smaller sub-lists until each sub-list contains only one element.
These sub-lists are then merged back together in order, until the original list has been completely sorted.
Here’s an example of how to use merge sort in Python:
<!-- Python code --> def merge_sort(lst): if len(lst) == 1: return lst middle = len(lst) // 2 left = lst[:middle] right = lst[middle:] left = merge_sort(left) right = merge_sort(right) return merge(left, right) def merge(left, right): result = [] while len(left) and len(right): if left[0] < right[0]: result.append(left.pop(0)) else: result.append(right.pop(0)) if len(left) > 0: result.extend(left) else: result.extend(right) return result lst = [5, 3, 8, 2, 0, 11, 23, 1] print(merge_sort(lst)) # Output: [0, 1, 2, 3, 5, 8, 11, 23]
Radix Sort
Radix sort is an algorithm that can be used for sorting lists of integers. It works by sorting the integers, from least significant to most significant, based on their digits.
This sorting is repeated for each digit, until the list is completely sorted.
Here’s an example of how to use radix sort in Python:
<!-- Python code --> def radix_sort(lst): m = max(lst) exp = 1 while m // exp > 0: counting_sort(lst, exp) exp *= 10 def counting_sort(lst, exp): n = len(lst) output = [0] * n count = [0] * 10 for i in range(n): index = lst[i] // exp count[index % 10] += 1 for i in range(1, 10): count[i] += count[i - 1] i = n - 1 while i >= 0: index = lst[i] // exp output[count[index % 10] - 1] = lst[i] count[index % 10] -= 1 i -= 1 i = 0 for i in range(n): lst[i] = output[i] lst = [5, 3, 8, 2, 0, 11, 23, 1] radix_sort(lst) print(lst) # Output: [0, 1, 2, 3, 5, 8, 11, 23]
These are just a few examples of the many advanced sorting techniques that can be used in Python. By using these techniques, you can gain more control over the ordering of your lists and achieve more specific sorting requirements.
FAQ – Are Lists Ordered Python
List order is a fundamental aspect of Python programming language. This section will address some common questions regarding the ordering of lists in Python.
Are Lists Ordered in Python?
Yes, lists are ordered in Python. The order of the elements in a list is determined by their position. The first element in a list is indexed as 0, the second element as 1, and so on. Therefore, the order of the elements in a list can be manipulated using indexing and slicing techniques.
How to Check if a List is Ordered in Python?
To check whether a list is ordered in Python, you can use the following methods:
- Sorted() function: This function sorts the elements of a list in ascending order, and returns a new sorted list. You can compare this sorted list with the original list to determine if it is already ordered.
- For loop: You can use a for loop to compare each list element with its succeeding element. The list is ordered if the for loop executes without any `break` statement.
- all() method: You can apply the `all()` method on the result of a for loop that compares each element of a list with its succeeding element. The `all()` method returns a Boolean value of `True` if all the elements in the loop are `True`, which means that the list is ordered.
How to Order a List in Python?
You can order a list in Python using the built-in sort() function or by implementing custom sorting techniques. The sort() function can be used to sort a list in ascending or descending order, depending on the arguments passed to it.
You can also create custom sorting functions that define your own criteria for sorting the elements in a list.
Can a List Contain Duplicate Elements but Still be Ordered?
Yes, a list can contain duplicate elements and still be ordered. The order of the elements in a list is based on their position in the list, not their value. Therefore, if two or more elements have the same value, they will still be ordered based on their positions in the list.
How to Check if a List is in Descending Order?
To check if a list is in descending order, you can reverse the order of the list using the `reverse()` method, and then compare it with the original list. If the reversed list is the same as the original list, then the original list is in descending order.
Conclusion
Understanding list ordering in Python is a crucial aspect of the language and programming in general. With the right knowledge and techniques, you can manipulate the order of the elements in a list to suit your needs.
We hope that this FAQ section has provided you with valuable insights into list ordering in Python.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.