Python is a dynamically typed programming language allowing you to easily create and manipulate data types. One of Python’s most commonly used data types is the list, an ordered collection of items of any data type.
One of the most debated topics around lists in Python is whether or not they are immutable. In this article, we’ll explore the characteristics of lists in Python, their mutability, and how they differ from other data types.
We’ll help you understand list immutability clearly, so you can confidently work with lists in your Python projects.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding List Mutability in Python
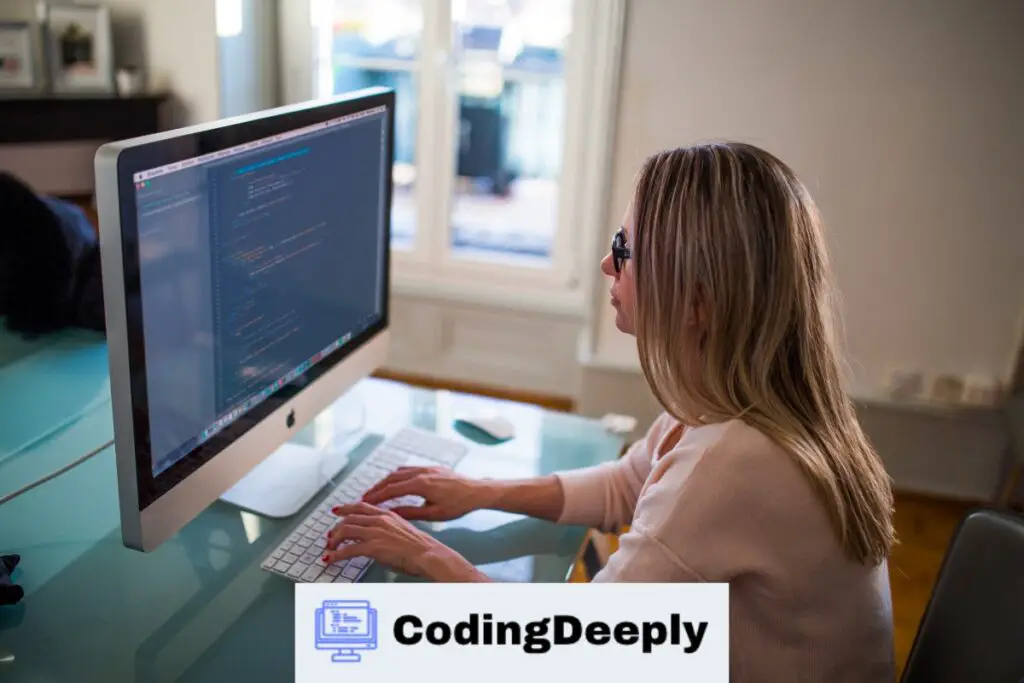
In Python, mutability refers to changing an object after its creation. Lists are mutable data types in Python, meaning you can modify them after they are created. This contrasts immutable data types, such as strings and tuples, which cannot be changed after creation.
The mutability of lists in Python is one of their key features, as it allows for efficient manipulation of large amounts of data. Being able to modify lists without creating new objects can improve the performance of your code.
Several common list operations in Python modify the list in place. These include:
- Appending items to the end of the list
- Inserting items at a specific position in the list
- Removing items from the list
- Sorting the list
Let’s take a look at each of these operations in more detail.
Appending Items to the End of the List
The append() method in Python allows you to add an item to the end of a list. Here’s an example:
Code | Result |
---|---|
my_list = [1, 2, 3] | [1, 2, 3] |
my_list.append(4) | [1, 2, 3, 4] |
You can see that the append() method adds the value 4 to the end of the list.
Inserting Items at a Specific Position in the List
The insert() method in Python allows you to insert an item at a specific position in a list. Here’s an example:
Code | Result |
---|---|
my_list = [1, 2, 3] | [1, 2, 3] |
my_list.insert(1, "a") | [1, 'a', 2, 3] |
You can see that the insert() method adds the value “a” at index 1 in the list, pushing the previous value at that index (2) to index 2.
Removing Items from the List
The remove() method in Python allows you to remove an item from a list by specifying its value. Here’s an example:
Code | Result |
---|---|
my_list = [1, 2, 3] | [1, 2, 3] |
my_list.remove(2) | [1, 3] |
You can see that the remove() method removes the value 2 from the list.
Sorting the List
The sort() method in Python allows you to sort a list in place. Here’s an example:
Code | Result |
---|---|
my_list = [3, 1, 2] | [3, 1, 2] |
my_list.sort() | [1, 2, 3] |
You can see that the sort() method sorts the list in ascending order.
Understanding list mutability in Python is an important concept to master when working with lists. You can write more efficient and concise code by modifying lists in place.
Modifying Lists in Python
Lists in Python are mutable, meaning you can modify their contents after they have been created. In this section, we’ll explore various ways to modify lists in Python, including adding and removing elements, changing elements in the list, and concatenating lists.
Adding Elements to Lists
You can use the append() method to add one element to the end of the list:
Code | Output |
---|---|
fruits = ['apple', 'banana', 'cherry'] | ['apple', 'banana', 'cherry', 'orange'] |
You can also use the insert() method to add an element at a specific index:
Code | Output |
---|---|
fruits = ['apple', 'banana', 'cherry'] | ['apple', 'orange', 'banana', 'cherry'] |
Removing Elements from Lists
You can use the remove() method to remove a specific element from the list:
Code | Output |
---|---|
fruits = ['apple', 'banana', 'cherry'] | ['apple', 'cherry'] |
You can also use the pop() method to remove an element at a specific index:
Code | Output |
---|---|
fruits = ['apple', 'banana', 'cherry'] | ['apple', 'cherry'] |
Changing Elements in Lists
You can access and change specific elements in the list using their index:
Code | Output |
---|---|
fruits = ['apple', 'banana', 'cherry'] | ['apple', 'orange', 'cherry'] |
Concatenating Lists
You can use the plus (+) operator to concatenate two or more lists:
Code | Output |
---|---|
fruits1 = ['apple', 'banana', 'cherry'] | ['apple', 'banana', 'cherry', 'orange', 'kiwi'] |
You can also use the extend() method to add all the elements of one list to another:
Code | Output |
---|---|
fruits1 = ['apple', 'banana', 'cherry'] | ['apple', 'banana', 'cherry', 'orange', 'kiwi'] |
Immutable Lists in Python
In addition to mutable lists, Python has a data type called a tuple, representing an immutable list.
What does immutable mean?
It means that once a tuple is created, its contents cannot be changed.
Like lists, tuples can store a sequence of elements, but they are defined with parentheses instead of square brackets:
my_tuple = (1, 2, 3)
Because tuples are immutable, they have some limitations compared to lists.
For example, you cannot add or remove elements from a tuple or change an element’s value once it’s been defined.
However, tuples do have some advantages over lists. They use less memory, which can be important when memory is scarce.
They also ensure that their contents cannot be accidentally modified, which can be useful in programs where data integrity is important.
Creating and Accessing Tuple Elements
Creating a tuple in Python is similar to creating a list:
Task | Code | Result |
---|---|---|
Create a tuple with three elements | my_tuple = (1, 2, 3) | my_tuple is now equal to (1, 2, 3) |
Access the first element of the tuple | my_tuple[0] | Returns 1 |
Access the last element of the tuple | my_tuple[-1] | Returns 3 |
Note that because tuples are immutable, you cannot change the value of an element:
Task | Code | Result |
---|---|---|
Try to change the first element of the tuple | my_tuple[0] = 4 | Produces an error: TypeError: ‘tuple’ object does not support item assignment |
Despite their limitations, tuples can be useful in certain situations where you need to ensure that the contents of a sequence cannot be modified.
List Comprehensions in Python
List comprehensions provide a concise way to create lists based on existing lists. They can be used as a replacement for loops and are often considered more Pythonic.
The basic structure of list comprehension is as follows:
[expression for item in list]
Where expression
is the operation to be performed on each item
in the list
. Let’s see some examples.
Examples
Example 1: We can use list comprehension to create a new list with the squares of even numbers from 1 to 10.
Code | Output |
---|---|
[x**2 for x in range(1, 11) if x % 2 == 0] | [4, 16, 36, 64, 100] |
In this example, we used the range()
function to create a sequence of numbers from 1 to 10. We then used list comprehension to square only the even numbers in the sequence by adding the condition if x % 2 == 0
.
Example 2: We can also use list comprehension to create a list of tuples from two separate lists.
Code | Output |
---|---|
[(x, y) for x in ['A', 'B', 'C'] for y in [1, 2, 3]] | [('A', 1), ('A', 2), ('A', 3), ('B', 1), ('B', 2), ('B', 3), ('C', 1), ('C', 2), ('C', 3)] |
In this example, we used list comprehension to create a list of tuples from two separate lists ['A', 'B', 'C']
and [1, 2, 3]
. The resulting list contains all possible combinations of elements from both lists.
As you can see, list comprehension can be a powerful tool for manipulating lists in Python. They offer a concise and efficient way to create new lists based on existing ones.
Sorting Lists in Python
Sorting lists is a common operation in many programming tasks, and Python provides a built-in function to accomplish this: sort(). This method allows you to sort a list in ascending order (from lowest to highest) or descending order (from highest to lowest).
To sort a list in ascending order, call the sort() method on the list:
my_list = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5] my_list.sort() print(my_list) Output: [1, 1, 2, 3, 3, 4, 5, 5, 5, 6, 9]
To sort a list in descending order, add the parameter reverse=True:
my_list = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5] my_list.sort(reverse=True) print(my_list) Output: [9, 6, 5, 5, 5, 4, 3, 3, 2, 1, 1]
Note that the sort() method modifies the original list in place rather than creating a new sorted list.
Sorting Lists of Strings
The sort() method can also be used to sort lists of strings. In this case, the strings will be sorted alphabetically by default:
my_list = ["apple", "banana", "cherry", "date", "elderberry"] my_list.sort() print(my_list) Output: ['apple', 'banana', 'cherry', 'date', 'elderberry']
To sort the list in reverse alphabetical order, use the parameter reverse=True:
my_list = ["apple", "banana", "cherry", "date", "elderberry"] my_list.sort(reverse=True) print(my_list) Output: ['elderberry', 'date', 'cherry', 'banana', 'apple']
You can also sort lists of strings based on the length of each string by using the key parameter:
my_list = ["apple", "banana", "cherry", "date", "elderberry"] my_list.sort(key=len) print(my_list) Output: ['date', 'apple', 'banana', 'cherry', 'elderberry']
This sorts the list in ascending order based on the length of each string.
Searching for Elements in Lists
Searching for specific elements in a list is a common task in Python programming.
This can be accomplished using the index() function, which returns the index of the first occurrence of the specified element in the list. The syntax for using the index() function is:
my_list.index(element)
where my_list
is the list to search and element
is the element to search for. If the element is not in the list, Python will raise a ValueError.
Let’s see a simple example:
fruits = ["apple", "banana", "cherry", "banana"] index = fruits.index("banana") print(index)
This will output:
1
Notice that the index() function returns the index of the first occurrence of the element in the list. In this case, the first occurrence of “banana” is at index 1.
Finding Multiple Occurrences
If the element you are searching for appears multiple times in the list, the index() function will only return the index of the first occurrence. If you need to find all occurrences of the element in the list, you can use a list comprehension:
fruits = ["apple", "banana", "cherry", "banana"] indices = [i for i in range(len(fruits)) if fruits[i] == "banana"] print(indices)
This will output:
[1, 3]
The list comprehension iterates over the indices of the list and adds each index to the indices
list if the element at that index is equal to “banana”.
Handling Missing Elements
If the element you are searching for is not in the list, the index() function will raise a ValueError:
fruits = ["apple", "banana", "cherry"] index = fruits.index("orange")
This will raise the following error:
ValueError: 'orange' is not in list
To prevent this error, you can check if the element is in the list using the in
keyword:
fruits = ["apple", "banana", "cherry"] if "orange" in fruits: index = fruits.index("orange") print(index) else: print("orange not found")
This will output:
orange not found
By using the in
you can safely search for elements in a list without raising a ValueError.
Slicing Lists in Python
Python makes it easy to extract specific parts of a list using slicing. Slicing works by specifying a starting and ending index and returns a new list containing the elements between those two indices.
Here’s an example:
Code | Output |
---|---|
my_list = [1, 2, 3, 4, 5] print(my_list[1:4]) | [2, 3, 4] |
In this example, we’re slicing the list my_list starting from index 1 and ending at index 4. The resulting sliced list contains the elements [2, 3, 4].
Slicing can also take a third argument, which specifies the step size. Here’s an example:
Code | Output |
---|---|
my_list = [1, 2, 3, 4, 5] print(my_list[0:5:2]) | [1, 3, 5] |
In this example, we’re slicing the list my_list starting from index 0 and ending at index 5, with a step size of 2. The resulting sliced list contains the elements [1, 3, 5].
Slicing can also be used to copy an entire list:
Code | Output |
---|---|
my_list = [1, 2, 3, 4, 5] new_list = my_list[:] print(new_list) | [1, 2, 3, 4, 5] |
In this example, we’re using slicing to create a new list new_list that contains all the elements of my_list.
Extending and Joining Lists in Python
In Python, you can easily combine multiple lists into a single list using the extend() method. This method appends the elements of one list to another list, effectively joining them together.
Here’s an example:
Code | Output |
---|---|
fruits = ['apple', 'banana', 'cherry'] colors = ['red', 'yellow', 'pink'] fruits.extend(colors) print(fruits) |
['apple', 'banana', 'cherry', 'red', 'yellow', 'pink'] |
You can also use the + operator to join two lists together. Here’s an example:
Code | Output |
---|---|
list1 = [1, 2, 3] list2 = [4, 5, 6] result = list1 + list2 print(result) |
[1, 2, 3, 4, 5, 6] |
When you use the + operator, a new list is created that contains the elements of both lists. This can be useful if you want to keep the original lists unchanged.
One thing to note is that the original lists are modified when you use the extend() method or the + operator.
If you don’t want to modify the original lists, you can create a new list using the list() constructor and join the lists together using the + operator.
Here’s an example:
Code | Output |
---|---|
list1 = [1, 2, 3] list2 = [4, 5, 6] result = list(list1) + list(list2) print(result) |
[1, 2, 3, 4, 5, 6] |
In addition to joining lists together, you can also use the join() method to combine a list of strings into a single string.
Here’s an example:
Code | Output |
---|---|
words = ['Hello', 'World'] result = ' '.join(words) print(result) |
'Hello World' |
The join() method takes a separator as an argument, separating the resulting string’s elements. In this example, we used space as the separator.
Using Lists in Python Projects
Lists are one of the most commonly used data structures in Python projects. They are versatile and can be used in various ways to manipulate and organize data. Here are some examples of how lists can be used:
- Storing and accessing data: Lists can store data in a structured way, allowing for easy access and manipulation later on.
- Iterating through data: Lists can be used in loops to iterate through the data and perform operations on each element.
- Filtering data: Lists can filter data based on certain criteria, such as finding all elements that meet a certain condition.
- Sorting data: Lists can be sorted using various methods, allowing for quick and easy data organization.
Here are some examples of how lists are commonly used in Python projects:
Project Type | Example List Use Case |
---|---|
Web Development | Storing user inputs or form data |
Data Science | Storing and manipulating large datasets |
Game Development | Storing game states or character attributes |
Lists are a fundamental part of Python programming and are used in various applications. It is essential for Python developers to understand how lists work and how to use them effectively.
FAQ about List Immutability in Python
Here are some common questions and misconceptions about list immutability in Python:
Can you make a list immutable in Python?
While lists in Python are mutable by default, you can create an immutable version of a list by using a tuple. Tuples are similar to lists but immutable, meaning that you cannot modify their contents once they are created.
What is the difference between mutable and immutable objects in Python?
Mutability refers to whether an object’s contents can be changed after creation. Mutable objects, such as lists, can be modified, while immutable objects, such as tuples, cannot.
Immutable objects are generally considered safer to use in concurrent or multithreaded environments, as they cannot be modified by multiple threads simultaneously.
Are there any downsides to using immutable objects in Python?
While immutable objects can provide certain benefits in certain situations, such as thread safety or improved performance, they also have limitations.
For example, because immutable objects cannot be modified, you may need to create new objects whenever you want to change their contents. In addition, some operations may be more difficult or less efficient with immutable objects than with mutable ones.
When should I use a list instead of a tuple?
Lists are generally used when you need to modify the contents of a sequence, while tuples are used when you need a fixed collection of values that cannot be changed.
If you are unsure whether to use a list or a tuple, consider whether you need to modify the contents of the sequence or not. If you do not need to modify the contents, use a tuple.
Can you create a copy of an immutable list in Python?
Because tuples are immutable, you can create a copy of a tuple by assigning it to a new variable.
However, if you want to create a copy of a list, you need to use the copy() method or slice notation to create a new list with the same contents. Otherwise, both variables will point to the same list object, and modifying one variable will also modify the other.
Can lists and tuples be mixed in Python?
Yes, you can create lists and tuples that contain other lists and tuples.
It is common to use lists and tuples together to represent structured data, such as rows in a database.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.