Python is a versatile programming language known for its library-rich ecosystem that can help speed up your workflow. If you’re new to Python, you may find appending to a list in a dictionary a bit confusing.
But don’t worry; we’ve got you covered with some tips and tricks on how to append to a list in a dictionary in Python easily.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding Python Dictionaries
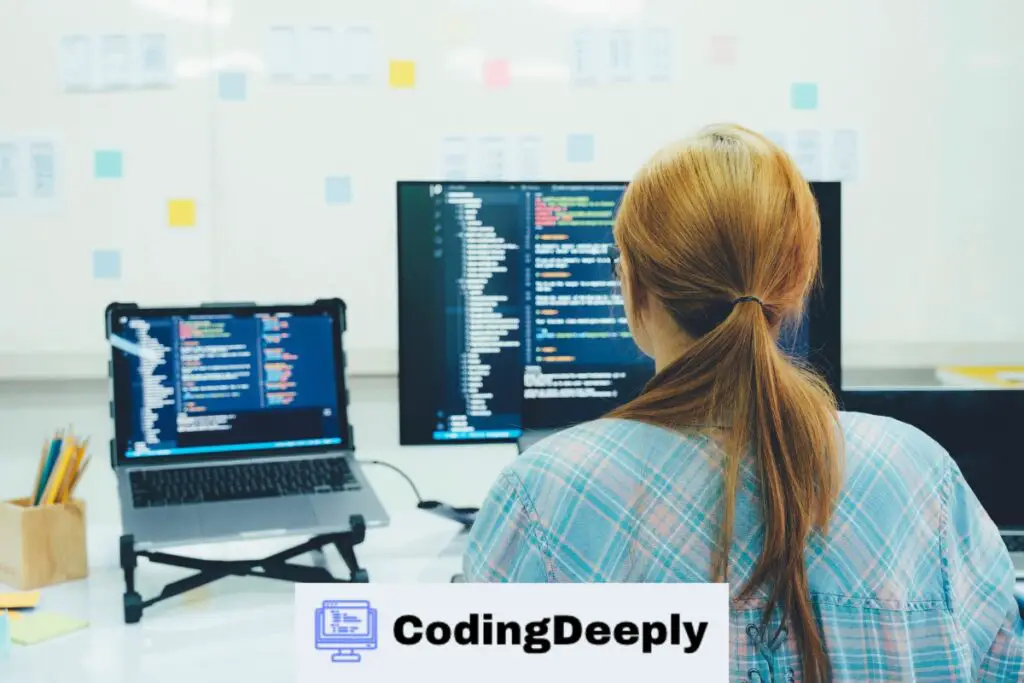
In Python, a dictionary is a collection of key-value pairs where each key is unique and associated with a corresponding value. A dictionary is mutable, which means you can modify it by adding, deleting, or updating key-value pairs.
To create a dictionary in Python, you can use curly braces {} and separate the key-value pairs with a colon:
{ "name": "John", "age": 30, "city": "New York" }
In this example, “name”, “age”, and “city” are the keys, while “John”, 30, and “New York” are their corresponding values.
You can access the value of a specific key in a dictionary by using its key in square brackets []. For example, if you want to access the value of the “age” key in the dictionary above, you can do:
my_dict = { "name": "John", "age": 30, "city": "New York" } age = my_dict["age"] print(age) # Output: 30
Dictionary Operations
Python dictionaries have several useful operations that you can use to manipulate them. Here are some of the most common ones:
Operation | Description |
---|---|
len(my_dict) | Returns the number of key-value pairs in the dictionary |
my_dict.keys() | Returns a list of all the keys in the dictionary |
my_dict.values() | Returns a list of all the values in the dictionary |
my_dict.items() | Returns a list of tuples, where each tuple contains a key-value pair |
my_dict.get(key, default=None) | Returns the value of the specified key. If the key does not exist, it returns the default value (which is None by default) |
my_dict.pop(key, default=None) | Removes the key-value pair with the specified key. If the key does not exist, it returns the default value (which is None by default) |
Understanding these basic operations of Python dictionaries is crucial to effectively append lists to them, which we will explore in the next section.
Creating Python Dictionaries
Before we can append a list to a dictionary in Python, we must create the dictionary and add items.
A dictionary in Python is a collection of key-value pairs, where each key is unique. Keys can be any immutable data type, such as strings or numbers, while values can be any data type, including lists.
To create a dictionary, we use curly braces {} and separate each key-value pair with a colon. For example:
Code | Output |
---|---|
my_dict = {'apple': 1, 'banana': 2, 'orange': 3} | {‘apple’: 1, ‘banana’: 2, ‘orange’: 3} |
We can also use the built-in function dict()
to create a dictionary:
Code | Output |
---|---|
my_dict = dict(apple=1, banana=2, orange=3) | {‘apple’: 1, ‘banana’: 2, ‘orange’: 3} |
Adding items to a dictionary is also easy, we assign a value to a new key:
Code | Output |
---|---|
my_dict['kiwi'] = 4 | {‘apple’: 1, ‘banana’: 2, ‘orange’: 3, ‘kiwi’: 4} |
Alternatively, we can use the built-in method update()
to add multiple items at once:
Code | Output |
---|---|
my_dict.update({'peach': 5, 'pear': 6}) | {‘apple’: 1, ‘banana’: 2, ‘orange’: 3, ‘kiwi’: 4, ‘peach’: 5, ‘pear’: 6} |
Now that we have our dictionary, we can move on to appending a list to it.
Creating Lists in Python
Before we can append a list to a dictionary in Python, we need to create the list itself. In Python, a list is a collection of items enclosed in square brackets and separated by commas. Here’s an example:
Example | Description |
---|---|
my_list = [1, 2, 3, 4] | A list of integers |
my_list = ['apple', 'banana', 'orange'] | A list of strings |
my_list = [1, 'apple', True] | A list of mixed data types |
You can create an empty list by simply using a pair of square brackets [] like this:
my_list = []
Once you have created a list, you can manipulate it in many ways. You can add, remove, or change items in the list, sort it, or even loop through it.
Here are some common list operations:
- Accessing list items: You can access individual items in a list by using their index number, which starts at 0. For example:
my_list[0]
returns the first item in the list. - Modifying list items: You can modify an item in a list by assigning a new value to its index. For example:
my_list[0] = 5
changes the first item in the list to 5. - Adding items to a list: Using the method, you can add items to the end of a list. For example:
my_list.append(5)
adds the integer 5 to the end of the list. - Removing items from a list: Using the method, you can remove an item from a list. For example:
my_list.remove('apple')
removes the string ‘apple’ from the list. - Sorting a list: You can sort a list using the
sort()
method. For example:my_list.sort()
sorts the list in ascending order. - Looping through a list: You can loop through a list using a
for
loop. For example:
for item in my_list: print(item)
This will print each item in the list on a new line.
Appending Lists to Dictionaries
Now that we understand how dictionaries and lists work let’s combine them by appending a list to a dictionary.
Here are the steps to follow:
- Declare a dictionary with the key-value pair where the value is an empty list.
Example:
Code: | my_dict = {‘key’: []} |
---|---|
Output: | {‘key’: []} |
- Access the key in the dictionary and append a list to it.
Example:
Code: | my_dict[‘key’].append([‘item1’, ‘item2’]) |
---|---|
Output: | {‘key’: [[‘item1’, ‘item2’]]} |
- Access the key again and append another list to it.
Example:
Code: | my_dict[‘key’].append([‘item3’, ‘item4’]) |
---|---|
Output: | {‘key’: [[‘item1’, ‘item2’], [‘item3’, ‘item4’]]} |
And that’s it! You have successfully appended lists to a dictionary in Python.
Overwriting List Values in Dictionaries
If you need to update a specific value in a list within a dictionary, you can use the index of the list to overwrite the existing value. The following code demonstrates how to overwrite a value in a list within a dictionary:
my_dict = {'key1': [1, 2, 3], 'key2': [4, 5, 6]} my_dict['key1'][0] = 0 print(my_dict)
The output of this code will be:
{'key1': [0, 2, 3], 'key2': [4, 5, 6]}
In this example, we changed the value of the first element in the list at ‘key1’ from 1 to 0. Note that we accessed the list using the key ‘key1’, and then used the index [0] to update the first value in the list.
If you need to update multiple values in the list, you can use multiple index references to overwrite them:
my_dict = {'key1': [1, 2, 3], 'key2': [4, 5, 6]} my_dict['key1'][0] = 0 my_dict['key1'][1] = 5 print(my_dict)
The output of this code will be:
{'key1': [0, 5, 3], 'key2': [4, 5, 6]}
Here, we updated the values at index 0 and 1 in the list at ‘key1’.
It’s important to note that if you try to access an index that does not exist in the list, you will get an “IndexError” exception.
Similarly, if you try to access a key that does not exist in the dictionary, you will get a “KeyError” exception. Make sure to handle these exceptions appropriately when overwriting list values in dictionaries.
Accessing List Values in Dictionaries
Now that you have learned how to append lists to dictionaries and overwrite list values let’s talk about accessing list values in dictionaries. Accessing values in a list in a dictionary is similar to accessing values in a normal list.
You can use the key value to access the list and the index value to access individual items.
Here is an example of how to access the first item in a list that is stored in a dictionary:
my_dict = {'key': ['value1', 'value2', 'value3']} print(my_dict['key'][0])
Output:
'value1'
In this example, ‘key’ is the key in the dictionary that stores the list [‘value1’, ‘value2’, ‘value3’]. We then use the index value of 0 to access the first item in the list, ‘value1’.
You can also use loops to access all the items in the list:
my_dict = {'key': ['value1', 'value2', 'value3']} for item in my_dict['key']: print(item)
Output:
'value1' 'value2' 'value3'
Using a loop, you can access all the items in the list without knowing the exact number of items in advance.
Emphasizing the Importance of Correct Syntax
When accessing values in a list in a dictionary, it’s important to use the correct syntax.
Ensure you include the key value to access the list and the index value to access individual items. Failure to use the correct syntax can result in errors or unexpected outputs.
Deleting List Values in Dictionaries
Deleting list values in dictionaries is an important part of managing the data stored in your dictionaries. There are two main ways to delete list values: deleting a specific value from the list or deleting the entire list from the dictionary.
Deleting a Specific Value from a List
To delete a specific value from a list in a dictionary, you need to use the del
keyword. Here is an example:
my_dict = {'key1': [1, 2, 3], 'key2': [4, 5, 6]} del my_dict['key1'][1]
This deletes the second item (index 1) from the list associated with ‘key1’. The resulting dictionary will look like this:
Key | Value |
---|---|
‘key1’ | [1, 3] |
‘key2’ | [4, 5, 6] |
As you can see, the second item in the list, 2, has been removed.
Deleting an Entire List from a Dictionary
You can use the keyword again to delete an entire list from a dictionary. This time, you specify the key-value pair you wish to delete. For example:
my_dict = {'key1': [1, 2, 3], 'key2': [4, 5, 6]} del my_dict['key1']
This will remove the key-value pair associated with ‘key1’, the list [1, 2, 3]. The resulting dictionary will look like this:
Key | Value |
---|---|
‘key2’ | [4, 5, 6] |
Only the key-value pair associated with ‘key2’ remains in the dictionary.
Deleting list values in dictionaries can be useful if you need to remove specific data from your dictionary. However, be careful when deleting data, as it can impact other parts of your code that rely on that data.
Using Loops for List Appends in Dictionaries
If you have many lists to append to a dictionary, doing it manually can be tedious. In such cases, it is more efficient to use a loop. Loops iterate through a sequence and execute the same code block repeatedly until the sequence is exhausted.
In Python, there are two types of loops: for loops and while loops.
Loops are used when you know the number of times you want to execute a block of code, while loops are suitable when you want to repeat a block of code until a certain condition is met.
To append multiple lists to a dictionary using a loop, you can create a list of lists and iterate through each list, appending it to the dictionary using the update()
method. Here is an example:
Code | Description |
---|---|
dict1 = {'a': [1, 2]} | Create a dictionary with a list as the value of a key |
list1 = [3, 4] | Create a list to append to the dictionary |
list2 = [5, 6] | Create another list to append to the dictionary |
list_of_lists = [list1, list2] | Create a list of the lists to append to the dictionary |
for lst in list_of_lists: dict1.update({'b': lst}) | Iterate through the list of lists and append each list to the dictionary using the update() method |
After running this code, the dictionary will have the following values:
- Key ‘a’: [1, 2]
- Key ‘b’: [3, 4] (first iteration) and [5, 6] (second iteration)
Using a loop to append multiple lists to a dictionary can save a lot of time when dealing with a large amount of data. It also allows you to write more concise and readable code.
Best Practices for Appending to a List in a Dictionary
Appending to a list in a dictionary can be a powerful tool in your Python arsenal. Here are some best practices to follow:
- Always use descriptive keys: When creating a dictionary, it’s important to use descriptive keys that make it easy to understand what each key represents. This will make it easier to append lists to the dictionary later on.
- Avoid hard-coding values: Hard-coding values can make your code inflexible and difficult to read. Instead, use variables to store values and update them as needed.
- Consider using defaultdict: If you’re working with a large dictionary and need to append to lists frequently, consider using defaultdict. This will allow you to append to a list without checking if the key exists first.
- Use try and except blocks: When appending to a dictionary, it’s possible to encounter errors if the key doesn’t exist. To avoid this, use try and except blocks to catch any errors and handle them appropriately.
- Document your code: When working with dictionaries and lists, it’s important to document your code so that others can understand how it works. Use comments to explain what each part of your code does and why you chose to do it that way.
Frequently Asked Questions (FAQs)
As you’ve learned in this article, appending to a list in a dictionary in Python can be a valuable technique for manipulating your data. However, you may still have some questions about the process.
Can you append a dictionary to a list in Python?
Yes, you can append a dictionary to a list in Python. You can use the append() method to add a dictionary to a list just like any other item.
How do you add an item to a dictionary in Python?
To add an item to a dictionary in Python, you can use the dictionary[key] = value syntax. For example:
dict = {‘name’: ‘John’, ‘age’: 25}
dict[‘address’] = ‘123 Main St’
This will add the key-value pair ‘address’: ‘123 Main St’ to the dictionary.
How do you append to a list in a dictionary without overwriting the existing values?
To append to a list in a dictionary without overwriting the existing values, you can use the += operator. For example:
dict = {‘name’: ‘John’, ‘hobbies’: [‘reading’, ‘running’]}
dict[‘hobbies’] += [‘swimming’]
This will add ‘swimming’ to the end of the ‘hobbies’ list without overwriting the existing values.
We hope these FAQs have addressed any questions you may have had about appending to a list in a dictionary in Python. If you have any further questions or concerns, don’t hesitate to consult the Python documentation or seek out assistance from the Python community.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.