Welcome to this article that will teach you how to master appending to a list in a dictionary Python.
As a Python programmer, understanding how to manipulate data using dictionaries and lists is a fundamental skill, and appending to a list in a dictionary is a crucial operation to master.
Appending to a list in a dictionary Python is a simple yet important operation that enables you to add items to a list associated with a specific key in a dictionary.
It allows you to store and manipulate large amounts of data efficiently, making it an essential skill for any Python developer.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding Python Dictionaries
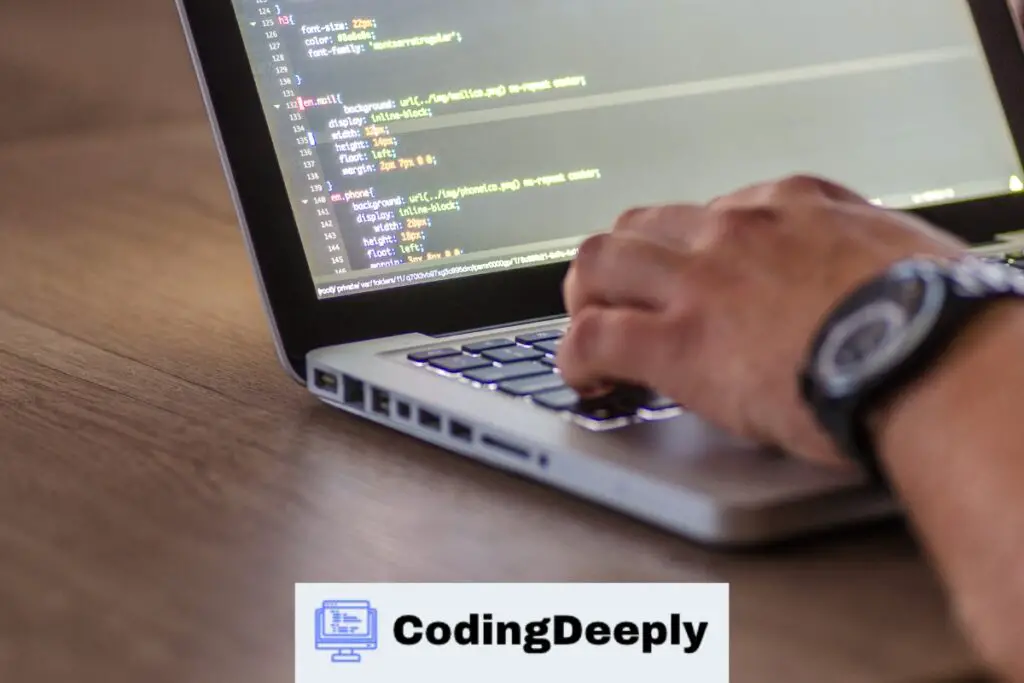
In Python, a dictionary is a collection of key-value pairs. Each key is unique and maps to a specific value. Dictionaries are mutable, which means they can be changed after creation.
The values in a dictionary can be of any data type, including strings, numbers, and even other data structures like lists.
To create a dictionary in Python, you can use curly braces {} or the dict() function. Here’s an example:
my_dict = {'name': 'John', 'age': 27, 'hobbies': ['reading', 'playing guitar']}
In the example above, we have a dictionary with three key-value pairs.
The keys are ‘name’, ‘age’, and ‘hobbies’; the corresponding values are ‘John’, 27, and the list [‘reading’, ‘playing guitar’].
You can access the values in a dictionary by using their keys. Here’s how to access the value of the ‘name’ key:
name = my_dict['name']
In the example above, the variable name will hold the value ‘John’.
Working with Lists in a Dictionary
One of the benefits of dictionaries in Python is that they can contain lists as values. To access a list in a dictionary, you can use the key and index notation.
Here’s an example:
hobbies = my_dict['hobbies'] first_hobby = hobbies[0]
In the example above, we first retrieve the list of hobbies using the ‘hobbies’ key. Then we access the first hobby in the list using the index notation.
You can also add items to a list that’s a value in a dictionary. We’ll explore this further in the next section.
Appending to a List in a Dictionary Python
Appending a value to a list in a dictionary Python is a common operation that allows you to add new items to an existing list within a dictionary. There are several ways to achieve this, and we’ll cover the most common methods below.
Method 1: Using the append() Method
The simplest method to append to a list in a dictionary in Python is by using the built-in append() method. Let’s say we have a dictionary with a list of fruits:
fruit_dict |
---|
{“fruits”: [“apple”, “banana”, “orange”]} |
We can append a new fruit to the list with the following syntax:
fruit_dict[“fruits”].append(“mango”) |
---|
The dictionary will now look like this:
fruit_dict |
---|
{“fruits”: [“apple”, “banana”, “orange”, “mango”]} |
Method 2: Using the “+” Operator
Another way to append to a list in a dictionary Python is by using the “+” operator to concatenate the existing list with a new list containing the item to be added.
Here’s an example where we add a new fruit to our dictionary of fruits:
fruit_dict[“fruits”] += [“grapefruit”] |
---|
The dictionary will now look like this:
fruit_dict |
---|
{“fruits”: [“apple”, “banana”, “orange”, “mango”, “grapefruit”]} |
Method 3: Using the extend() Method
The extend() method can also be used to append to a list in a dictionary Python. This method extends the existing list by appending all the items from the iterable passed as an argument.
Here’s an example where we add multiple fruits to our dictionary of fruits:
fruit_dict[“fruits”].extend([“kiwi”, “watermelon”]) |
---|
The dictionary will now look like this:
fruit_dict |
---|
{“fruits”: [“apple”, “banana”, “orange”, “mango”, “grapefruit”, “kiwi”, “watermelon”]} |
Appending to a list in a dictionary, Python is a powerful tool allowing you to update your data dynamically.
Keep practicing these methods to master this skill.
Updating Lists in a Dictionary Python
In addition to appending to a list in a dictionary Python, you may need to update the entire list or add elements to an existing list. This section will cover some basic methods to do so.
Replacing the Entire List
If you want to replace the entire list for a specific key in your dictionary, you can use the assignment operator (=) to assign a new list to that key. Here’s an example:
Code | Output |
---|---|
my_dict = {‘fruits’: [‘apple’, ‘banana’, ‘cherry’]} my_dict[‘fruits’] = [‘orange’, ‘grape’, ‘kiwi’] print(my_dict) | {‘fruits’: [‘orange’, ‘grape’, ‘kiwi’]} |
In this example, we replace the entire list of fruits with a new list of different fruits.
Adding to an Existing List
If you want to add elements to an existing list in your dictionary, you can use the append()
method. Here’s an example:
Code | Output |
---|---|
my_dict = {‘fruits’: [‘apple’, ‘banana’, ‘cherry’]} my_dict[‘fruits’].append(‘orange’) print(my_dict) | {‘fruits’: [‘apple’, ‘banana’, ‘cherry’, ‘orange’]} |
In this example, we add a new fruit to the existing list of fruits using the append()
method.
Updating a List with Another List
If you have another list and you want to append it to an existing list in your dictionary, you can use the extend()
method. Here’s an example:
Code | Output |
---|---|
my_dict = {‘fruits’: [‘apple’, ‘banana’, ‘cherry’]} new_fruits = [‘orange’, ‘grape’, ‘kiwi’] my_dict[‘fruits’].extend(new_fruits) print(my_dict) | {‘fruits’: [‘apple’, ‘banana’, ‘cherry’, ‘orange’, ‘grape’, ‘kiwi’]} |
In this example, we extend the existing list of fruits with a new list of fruits using the extend()
method.
These are some basic methods to update lists in a dictionary Python. Depending on your use case, you may need to use other methods such as insert()
or remove()
to manipulate the lists in your dictionary. Make sure to check the Python documentation for more information.
Adding an Element to a List in a Dictionary Python
Appending a value to a list in a dictionary is a basic operation. However, sometimes we need to add a new element to a specific position in the list.
This section will cover three methods to add an element to a list in a dictionary Python.
Adding to the beginning of a list
We use the insert method to add an element to the beginning of a list in a dictionary in Python. This method takes two arguments: the index of the position where we want to insert the element and the value of the element.
The syntax is as follows:
Method | Description |
---|---|
my_dict[‘my_list’].insert(0, ‘new_element’) | Inserts a new_element at the beginning of the list in my_dict |
Here is an example:
my_dict = {'my_list': [1, 2, 3, 4]} my_dict['my_list'].insert(0, 'new_element') print(my_dict)
The output will be:
{'my_list': ['new_element', 1, 2, 3, 4]}
Adding to the end of a list
Appending an element to the end of a list in a dictionary in Python is the same as appending to a list in Python. We use the append method, which adds the element to the end of the list. The syntax is as follows:
Method | Description |
---|---|
my_dict[‘my_list’].append(‘new_element’) | Adds a new_element to the end of the list in my_dict |
Here is an example:
my_dict = {'my_list': [1, 2, 3, 4]} my_dict['my_list'].append('new_element') print(my_dict)
The output will be:
{'my_list': [1, 2, 3, 4, 'new_element']}
Adding to a specific index in the list
To add an element to a specific index in a list in a dictionary Python, we use the insert method with the index of the position where we want to insert the element and the value of the element.
The syntax is as follows:
Method | Description |
---|---|
my_dict[‘my_list’].insert(index, ‘new_element’) | Inserts a new_element at the specified index in the list in my_dict |
Here is an example:
my_dict = {'my_list': [1, 2, 3, 4]} index = 2 my_dict['my_list'].insert(index, 'new_element') print(my_dict)
The output will be:
{'my_list': [1, 2, 'new_element', 3, 4]}
Now that you know how to add elements to a list in a dictionary Python, you can perform more complex operations on your data structures.
List Manipulation in a Dictionary Python
Manipulating lists in a dictionary Python can be a powerful tool in data analysis and other applications. Here are some advanced techniques for manipulating lists in a dictionary.
Removing Elements from a List
To remove elements from a list in a dictionary Python, you can use the remove() method. This method takes a parameter that specifies the element to remove. For example:
Code | Result |
---|---|
my_dict = {‘numbers’: [1, 2, 3, 4, 5]} my_dict[‘numbers’].remove(3) print(my_dict) | {‘numbers’: [1, 2, 4, 5]} |
This code removes the element ‘3’ from the list with key ‘numbers’ in the dictionary. The resulting dictionary has the updated list with ‘3’ removed.
Sorting a List
To sort a list in a dictionary Python, you can use the sort() method. This method sorts the list in ascending order by default. For example:
Code | Result |
---|---|
my_dict = {‘numbers’: [3, 2, 5, 1, 4]} my_dict[‘numbers’].sort() print(my_dict) | {‘numbers’: [1, 2, 3, 4, 5]} |
This code sorts the list with key ‘numbers’ in the dictionary in ascending order. The resulting dictionary has the updated list with the sorted values.
Reversing a List
To reverse a list in a dictionary Python, you can use the reverse() method. This method reverses the order of the elements in the list. For example:
Code | Result |
---|---|
my_dict = {‘numbers’: [1, 2, 3, 4, 5]} my_dict[‘numbers’].reverse() print(my_dict) | {‘numbers’: [5, 4, 3, 2, 1]} |
This code reverses the order of the list with key ‘numbers’ in the dictionary. The resulting dictionary has the updated list with the reversed order.
Best Practices for Appending to a List in a Dictionary Python
Appending to a list in a dictionary, Python can be a powerful tool for organizing and manipulating data. To get the most out of this operation, it’s important to follow these best practices:
Avoid Duplicate Entries
When appending to a list in a dictionary Python, it’s essential to avoid adding duplicate entries. To do this, first check if the value you’re appending already exists in the list using the ‘in’ operator. If it does, then don’t append it again.
Handle Errors
Always be prepared to handle errors when appending to a list in a dictionary Python. If the key you’re trying to append to does not exist, it will raise a KeyError. To avoid this, use the ‘setdefault’ method to create a new list if the key doesn’t exist already.
Optimize Performance
Appending to a list in a dictionary Python can be resource-intensive if done repeatedly. To optimize performance, consider using the ‘defaultdict’ method from the ‘collections’ module to create a dictionary with a default value of an empty list. This way, you won’t need to check if the key exists before appending to the list.
Example:
Let’s say we have a dictionary ‘favorite_foods’ that contains a list of favorite foods for each person:
Name | Favorite Foods |
---|---|
Alice | [‘pizza’, ‘pasta’, ‘sushi’] |
Bob | [‘tacos’, ‘burritos’] |
To append a new favorite food for Alice, we can use the following code:
favorite_foods['Alice'].append('ramen')
If we want to add a new person with an empty list of favorite foods, we can use the ‘setdefault’ method:
favorite_foods.setdefault('Charlie', [])
Now, we can append a new favorite food for Charlie:
favorite_foods['Charlie'].append('chicken curry')
Following these best practices can help you avoid errors and optimize performance when appending to a list in a dictionary Python. Happy coding!
Examples of Appending to a List in a Dictionary Python
Now that we’ve covered the basics of appending to a list in a dictionary Python, let’s look at some real-life examples of how this operation can be used in various scenarios.
Data Analysis
In data analysis, dictionaries often store data in a structured format. For example, you might have a dictionary with the following structure:
Employee | Department | Salary |
---|---|---|
John | HR | [50000, 55000, 60000] |
Jane | Marketing | [60000, 65000, 70000] |
In this example, the salary for each employee is stored as a list. If we want to add a new salary for John, we can use the following code:
employees['John']['Salary'].append(65000)
This will append the value 65000 to the end of the list for John’s salary.
Web Scraping
In web scraping, dictionaries can store information about web pages and their content. For example, you might have a dictionary with the following structure:
Page URL | Links |
---|---|
https://example.com | [‘https://example.com/about’, ‘https://example.com/contact’] |
https://example.com/about | [‘https://example.com’, ‘https://example.com/contact’] |
In this example, the links for each page are stored as a list. If we want to add a new link to the page https://example.com/about, we can use the following code:
pages['https://example.com/about']['Links'].append('https://example.com/services')
This will append the value ‘https://example.com/services’ to the end of the list for links on the page https://example.com/about.
Machine Learning
In machine learning, dictionaries are often used to store training data and corresponding labels. For example, you might have a dictionary with the following structure:
Image | Labels |
---|---|
image1.png | [‘cat’, ‘small’, ‘kitten’] |
image2.png | [‘dog’, ‘large’, ‘adult’] |
In this example, the labels for each image are stored as a list. If we want to add a new label to the image image1.png, we can use the following code:
training_data['image1.png']['Labels'].append('cute')
This will append the value ‘cute’ to the end of the list for labels on the image image1.png.
FAQ
Here are some frequently asked questions related to appending to a list in a dictionary Python:
1. How do I append to a list in a dictionary Python?
You can use the ‘append’ method or the ‘+=’ operator to append to a list in a dictionary Python. For example:
my_dict = {'key1': [1, 2, 3], 'key2': [4, 5, 6]} my_dict['key1'].append(4) my_dict['key2'] += [7, 8] print(my_dict)
This will output:
{'key1': [1, 2, 3, 4], 'key2': [4, 5, 6, 7, 8]}
2. How do I avoid appending duplicate entries to a list in a dictionary Python?
You can use the ‘in’ operator to check if the value you want to append exists in the list. If it does not exist, you can append it to the list. For example:
my_dict = {'key1': [1, 2, 3], 'key2': [4, 5, 6]} if 4 not in my_dict['key1']: my_dict['key1'].append(4) if 7 not in my_dict['key2']: my_dict['key2'].append(7) print(my_dict)
This will output:
{'key1': [1, 2, 3, 4], 'key2': [4, 5, 6, 7]}
3. How do I handle errors when appending to a list in a dictionary Python?
You can use try-except blocks to handle errors when appending to a list in a dictionary Python. For example:
my_dict = {'key1': [1, 2, 3], 'key2': 'not a list'} try: my_dict['key1'].append(4) my_dict['key2'].append(7) except AttributeError: print("Error: 'key2' is not a list.") print(my_dict)
This will output:
Error: 'key2' is not a list. {'key1': [1, 2, 3, 4], 'key2': 'not a list'}
4. How do I optimize performance when appending to a list in a dictionary Python?
One way to optimize performance is to use defaultdict instead of a regular dictionary. defaultdict automatically creates lists as values for new keys. For example:
from collections import defaultdict my_dict = defaultdict(list) my_dict['key1'].append(1) my_dict['key1'].append(2) print(my_dict)
This will output:
defaultdict(<class 'list'>, {'key1': [1, 2]})
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.