Do you encounter Python’s “maximum recursion depth exceeded” error frequently?
You’re not alone, so relax!
Especially for persons who are new to programming, this error might be upsetting.
This blog post will define this problem, examine its root causes, and offer helpful advice. We’ll also provide you with straightforward guidance on how to handle recursion in Python.
Let’s get started to learn everything there is to know about maximum recursion depth exceeded.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Key Takeaways
- A typical Python error that happens when a recursive function is called excessively and the program runs out of memory is “maximum recursion depth exceeded.”
- Deep recursion trees, endless recursion, and massive data sets are common sources of this problem.
- The best practices for dealing with recursion in Python include creating a base case, monitoring the depth of the recursion, employing tail recursion, testing your function, and utilizing debugging and optimization tools and resources.
- It’s crucial to address the root cause of the mistake and design effective code to avoid the “maximum recursion depth exceeded” error.
- Python applications for recursion include creating the Fibonacci sequence, iterating through data structures, and resolving challenging puzzles like the Tower of Hanoi.
Python Recursion: An Introduction
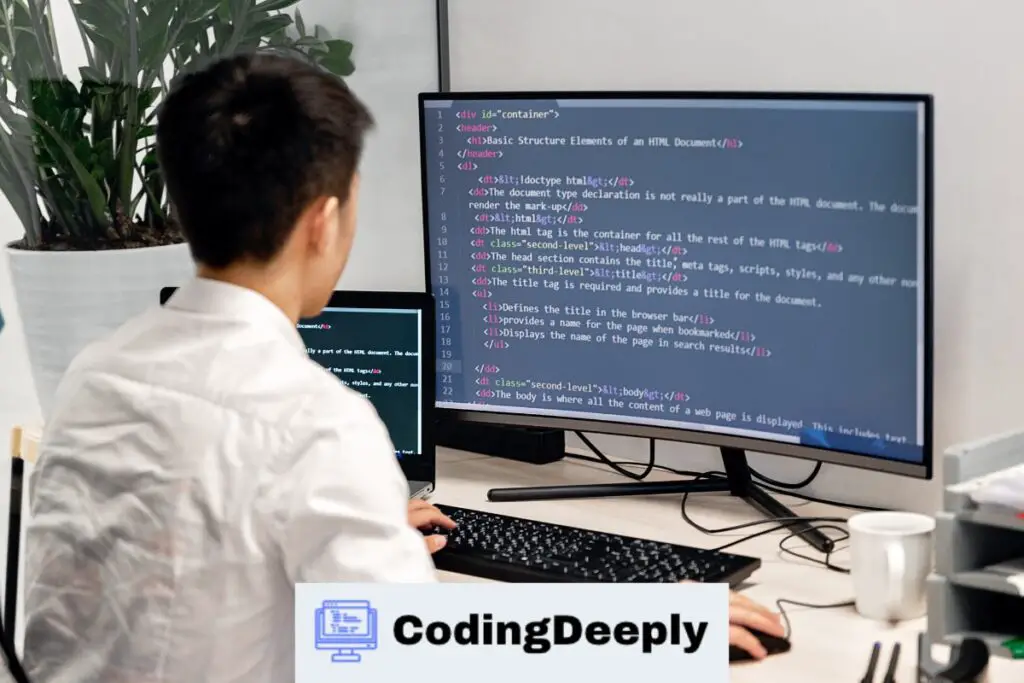
A strong programming method called recursion is used to handle big issues that can be divided into smaller, simpler ones.
A recursive function in Python executes itself several times.
A recursive function enters a new instance of itself and starts that instance’s execution when it is invoked.
This fresh instance might call itself once more, making new instances. A base case is eventually reached, marking the “bottom out” of the recursion.
A base case is a circumstance that halts recursive calls and permits the function to produce a conclusion.
Although recursion can be difficult to grasp initially, it can be a helpful technique for tackling specific issues.
The factorial of a number, creating the Fibonacci sequence, and traversing data structures like trees are a few frequent instances of recursive functions in Python.
Let’s look at a Python recursive function as an example:
def factorial(n): if n == 1: return 1 else: return n * factorial(n-1)
In this example, the function calls itself recursively until the base case is reached to determine the factorial of an integer (when n equals 1).
The function then returns the outcome.
You can use recursion to tackle complicated problems and produce effective code after you understand how it operates in Python.
Reasons for the Python-Error “Maximum Recursion Depth Exceeded”
A typical Python error that happens when a recursive function is called excessively and the program runs out of memory is “maximum recursion depth exceeded.”
The following are some of the causes of this error:
- If a recursive function doesn’t have a base case that terminates the recursion, it will loop back on itself indefinitely.
- Big data sets: A recursive function might soon run out of memory when invoked with huge data sets, resulting in the “maximum recursion depth exceeded” warning.
- Deep recursion trees: If a recursive function has a deep recursion tree, it may exceed the allowed maximum recursion depth, resulting in an error.
Typical errors that can result in this error include:
- Failing to define a base case in a recursive function might result in infinite recursion and the “maximum recursion depth exceeded” error.
- Recursion depth limit incorrectly set: If the recursion depth limit is set incorrectly, the problem may occur more frequently.
Error-Solutions: “Maximum Recursion Depth Exceeded”
The Python error “maximum recursion depth exceeded” has several fixes you can attempt. Let’s examine each answer in greater detail:
Bug-Fixing Methods
Debugging methods can be used to locate the error’s cause. Next, determine where the code is malfunctioning and resulting in the recursion issue. This can involve utilizing print statements or a debugger.
Raising the Recursion Depth Cap
You can raise the Python recursion depth limit with the sys.setrecursionlimit() function.
Raising the recursion depth limit might consume a significant amount of memory. Thus it should only be done with care.
Alternate Methods
Other methods of recursion may be employed in some circumstances to prevent the mistake.
You may choose an iterative strategy rather than a recursive one. As an alternative, you can employ tail recursion, a method that lessens the memory consumption of a recursive function.
Code Refactoring
Sometimes, the easiest way to fix the “maximum recursion depth exceeded” error is to refactor the code. You can reduce the required recursion by breaking the code into smaller, easier functions.
Using these methods, you can efficiently manage the “maximum recursion depth exceeded” problem and produce reliable Python code.
Practical Python Recursion Handling Advice
It’s critical to adhere to basic best practices and use resources and tools for debugging and optimizing recursive methods to manage recursion in Python efficiently.
Here are some helpful hints for managing recursion in Python:
- Create a base case: To prevent infinite recursion and the “maximum recursion depth exceeded” error, create a base case for every recursive function you use.
- Keep track of the recursion depth: Monitoring the recursion depth can aid in the optimization and debugging of recursive functions.
- Use tail recursion: This technique lowers the memory consumption of a recursive function. It’s a good idea to employ tail recursion.
- Test your function: Running tests on your function with various input values will allow you to find and correct any errors or problems.
- Use resources and tools: You may debug and improve your recursive function using various resources and tools. For instance, you can uncover performance issues and improve your code using a debugger or profiling tool.
Generating the Fibonacci sequence, navigating trees and graphs, and resolving challenging puzzles like the Tower of Hanoi are a few practical examples of using recursion in Python.
You can properly handle recursion in Python and produce efficient and effective code by paying attention to these pointers and using tools and resources to optimize your recursive methods.
Conclusion
Recursion is a powerful technique in Python to navigate data structures and solve challenging issues.
Recursion must be handled carefully to prevent the “maximum recursion depth exceeded” error. However, you may avoid this issue and create effective, efficient code by comprehending its reasons and adhering to best practices for developing recursive functions.
Remember to establish a base case, monitor the depth of the recursion, apply tail recursion when appropriate, test your function, and use debugging and optimization tools and resources.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.