You cannot possibly comprehend how strong the iterables are.
A group of tools for handling iterators may be found in the Python itertools package. If you look closely, they are intriguing, and this article will explain a little portion of that fascination.
In this article, find out about the itertools, chain, and extend. Also, find answers to some of the most asked questions online about itertools.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
What is Itertools in Python?
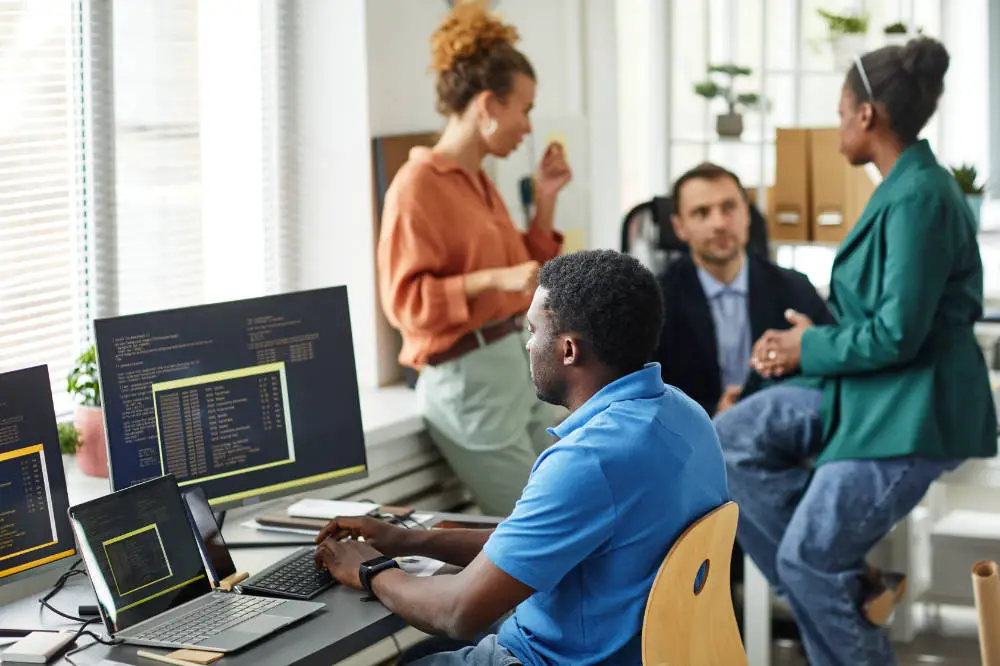
Itertool is a package in Python that offers several operations on iterators to create complicated iterators.
Iterator algebra may be created quickly and efficiently with the help of this module, either alone or in combination.
Let’s say, for illustration, that you wish to multiply the items of two lists.
This may be done in a variety of ways.
One might employ the naive technique by iterating through the components of both lists concurrently and multiplying them.
Another strategy involves utilizing the map function, which may be used by giving the mul operator as the function’s first parameter and lists as its second and third parameters.
There are two types of itertools.
You can either tun endlessly using infinite iterators or terminate using finite iterators.
Infinite Iterators include count, cycle, and repeat.
Finite Iterators include but are not limited to chain, compress, and dropwhile.
When Should You Use Itertools?
The Python itertools package cycles over data structures that may be walked over with a for-loop.
Iterables is another name for this kind of data structure.
Iterator algebra may be created quickly and efficiently with the help of this module, either alone or in combination.
This module includes features that make optimal use of computing resources. Additionally, using this module makes the code easier to understand and maintain.
What is a Chain in Itertools?
The method only returns one iterable after receiving a list of iterables. It compiles all of the iterables into a single output iterable. Its output must be explicitly turned into iterables because it cannot be utilized directly.
This procedure falls within the category of iterators that terminate iterators.
Let’s have a look at its syntax.
chain (*iterables)
The chain’s internal operation may be put into practice as follows:
def chain(*iterables): for it in iterables: for each in it: yield each
Example: There are different lists for odd and even integers. Create a new list by combining them.
from itertools import chain
# a list of odd numbers
odd = [1, 3, 5, 7, 9]
# a list of even numbers
even = [2, 4, 6, 8, 10]
# chaining odd and even numbers
numbers = list(chain(odd, even))
print(numbers)
And the output will be:
[1, 3, 5, 7, 9, 2, 4, 6, 8, 10]
What is an Extend in Itertools?
The supplied list members (or any iterable) are added to the end of the current list via the extend() function.
So, the extension in Python itertools would be a method that adds some list elements to the existing list. It extends it.
Let’s take a quick look at the syntax.
list.extend(iterable)
The example would be this one:
fruits = ['strawberry', 'orange', 'clementine']
points = (2, 5, 6, 1)
fruits.extend(points)
print(fruits)
Run it yourself and see the output!
What is the Difference Between Chain and Extend in Itertools Python?
To see the differences between the two, it is best to use a code and test it out.
We looked at the performance gap to compare the performance gains of various approaches.
The approaches in question are chain and extend.
Let’s see the results.
import timeit
from itertools import chain
def using_extend():
one = range(1, 1000000)
two = range(1, 1000000)
three = range(1, 1000000)
four = range(1, 1000000)
one.extend(two)
one.extend(three)
one.extend(four)
one.extend(two)
one.extend(three)
one.extend(four)
def using_chain():
one = range(1, 1000000)
two = range(1, 1000000)
three = chain(one, two, one, two, one, two)
if __name__ == '__main__':
repeat = 1000000
print('repeat {}'.format(repeat))
print('using extend --> {}'.format(timeit.timeit("using_extend", number=repeat, setup="from __main__ import using_extend")))
print('using chain --> {}'.format(timeit.timeit("using_chain", number=repeat, setup="from __main__ import using_chain")))
And here is the output in 2.7s:
Output:
repeat 1000000
using extend --> 0.0156660079956
using chain --> 0.0156710147858
And now, here is the output in 3.5s:
Output:
repeat 1000000
using extend --> 0.013913063099607825
using chain --> 0.013966921018436551
Frequently Asked Questions
As said at the beginning, we will answer a few frequently asked questions online about Itertools.
Let’s get started.
In Python, How Would You Chain Two Functions Together?
If you wish to design a function that may be called several times, you must first return a callable object (such as a function) each time.
If you don’t, you must declare a __call__ property on your object to make it callable.
Is Itertools Quick?
Yes.
Itertools are fast and very efficient in memory.
Itertools: Are They Quicker Than Loops?
Iteration through a typical Python for loop is frequently slower than iteration through itertools.
So, the answer would be – yes. The Itertools is quicker than Loops.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.