Do you wish to learn all there is to know about the Python Not None expression?
You’ve come to the proper location if so!
The realm of Not None in Python will be thoroughly explored in this blog article, along with its significance, use, and practical applications.
You’ll have thorough knowledge of Not None and how to apply it to routine programming chores by the time you finish reading this essay.
So let’s start now!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
The Key Takeaways
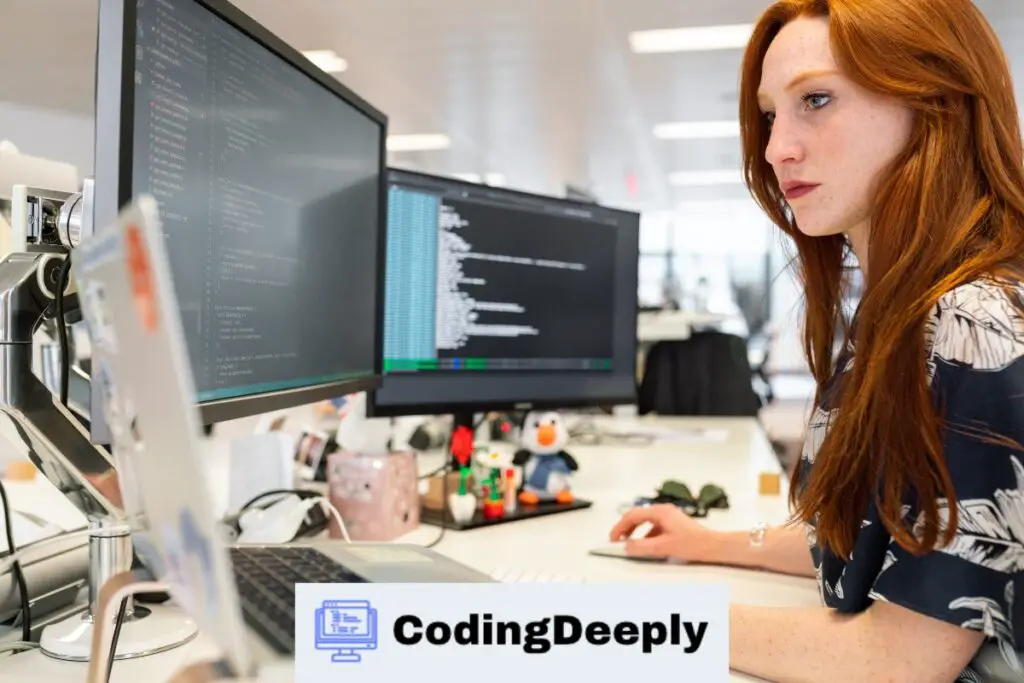
- None is a special Python object that represents the absence of a value.
- Not None is used to determine whether or not a variable has a value.
- Not None is often used in verifying user input and validating variable values and can help prevent mistakes in your code.
- Not None best practices include using the “is” operator instead of “!=” and avoiding using Not None as a default value.
- You can build correct and fast Python code by understanding the distinctions between None and Not None and following best practices.
What is None in Python?
In Python, None is a built-in constant that represents the absence of a value or the null value.
It is used to indicate that a variable has no value assigned to it or that a function or method does not return anything.
None is often used as a placeholder or a default value for variables, functions, and methods.
Complicated?
Let’s take a quick look an a few examples:
When defining a variable but not yet assigning a value to it, the default value is None.
codename = None
When a function or method does not return anything, it returns None by default.
def greet(name): print("Hello, " + name) result = greet("John") print(result) # Output: None
When a condition is not met, it may return None as well.
def get_element_at_index(lst, index): if index >= len(lst): return None return lst[index] my_list = [1, 2, 3] print(get_element_at_index(my_list, 5)) # Output: None
Why is None important in Python?
Quite simple: Understanding the use of None in Python is essential for writing clean and efficient code.
In the next section, we will dive deeper into the importance of the Not None expression in Python.
Differences between None and Not None
While None represents the absence of a value, Not None is a condition that checks whether a value exists or not.
See these examples:
None is a constant, whereas Not None is an expression that can be used in conditional statements.
codex = None if x is None: print("x is None") y = 5 if y is not None: print("y is not None")
None is often used as a default value for variables, parameters, and return values, whereas Not None is used to validate whether a value exists or not.
def add_numbers(a=None, b=None): if a is None or b is None: return None return a + b print(add_numbers(2, 3)) # Output: 5 print(add_numbers(2)) # Output: None
None is a single value that represents the absence of a value, whereas Not None can be any value apart from None.
codex = None y = 5 z = "Hello" if x is not None: print("x is not None") if y is not None: print("y is not None") if z is not None: print("z is not None")
Practical Applications
Here are two practical applications of how to use Not None:
#1: Checking if a variable has a value
When working with variables, it is essential to check whether they have a value or not.
Not None can be used in this case:
codename = None if name is not None: print("Hello, " + name) else: print("Please enter your name.")
In this example, we check if the variable “name” has a value. If it does not have a value, we prompt the user to enter their name.
#2: Validating user input
When working with user input, it’s important to validate the input to ensure that it is correct and complete.Here’s an example:
age = input("Please enter your age: ") if age is not None and age.isdigit(): print("Your age is " + age) else: print("Please enter a valid age.")
In this example, we check if the input value for age is not None and if it is a digit.
If the input is not None and is a digit, we print the age. Otherwise, we prompt the user to enter a valid age.
The best ways to use Not None
Here are some recommendations for utilizing Python’s Not None:
- To determine whether a variable has a value or not, use Not None.
- When determining whether a variable is not None, always use the “is not None” syntax rather than the “!= None” form.
- To check user input and guard against coding mistakes, utilize Not None.
- Unless absolutely required, refrain from setting variables’ default values to Not None. Instead, use a suitable default value.
You can build efficient, clear, and simple-to-read code by adhering to these best practices.
3 Tips for using Not None
While Not None is a useful expression in Python, there are a few tips and common pitfalls to keep in mind when using it.
Here are some practical tips for using Not None in Python:
Tip #1: Use Not None with “is” operator
When checking if a variable is not None, it is best to use the “is” operator instead of the “!=” operator.
x = None if x is not None: print("x has a value") else: print("x is None")
Using the “is” operator is more efficient and can prevent unexpected behavior in your code.
Tip #2: Use None as a default value for optional parameters
When defining a function or method with optional parameters, it is best to use None as the default value for the optional parameters.
def greet(name=None): if name is not None: print("Hello, " + name) else: print("Hello, world!") greet() greet("John")
In this example, we define a function “greet” with an optional parameter “name”.
If a value is passed for the “name” parameter, the function will greet the person by name. Otherwise, it will greet the world.
Tip #3: Use Not None to prevent errors
Using Not None can help prevent errors in your code. For example, if a variable has not been assigned a value, trying to use it can result in an error.
By using Not None to check if the variable has a value, you can prevent these errors.
x = None if x is not None: print("x has a value") else: print("x is None") # This will result in an error print("Value of x: " + x)
In this example, we use Not None to check if “x” has a value. If “x” is None, we print a message.
Trying to print the value of “x” without checking if it has a value will result in an error.
Common Pitfalls to Avoid
While Not None can be helpful in your Python code, there are also some common pitfalls to avoid.
Pitfall #1: Using Not None as a default value
Using Not None as a default value for a parameter can cause unexpected behavior in your code.
Instead, use an appropriate default value such as an empty list, dictionary, or string.
Pitfall #2: Using “!=” instead of “is not”
When checking if a variable is not None, always use the “is not” operator instead of the “!=” operator.
Using “!=” can lead to unexpected behavior and errors in your code.
Pitfall #3: Using Not None too often
While Not None can be helpful, overusing it can make your code less readable and more difficult to understand.
Use it only when necessary and make sure to follow best practices for using it.
Conclusion
The crucial expressions None and Not None in Python can be used to determine if a variable contains a value or not.
Not None is used to determine whether a value is present or not, whereas None denotes the absence of a value.
You can build correct and effective Python code by knowing the distinctions between None and Not None. Moreover, you can utilize Not None efficiently in your Python code by adhering to recommended practices and avoiding common mistakes.
Remember to check for None with the “is” operator rather than “!=”, to use None as the default value for optional arguments, and to refrain from using Not None excessively in your code.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.